6.1vector常见用法详解
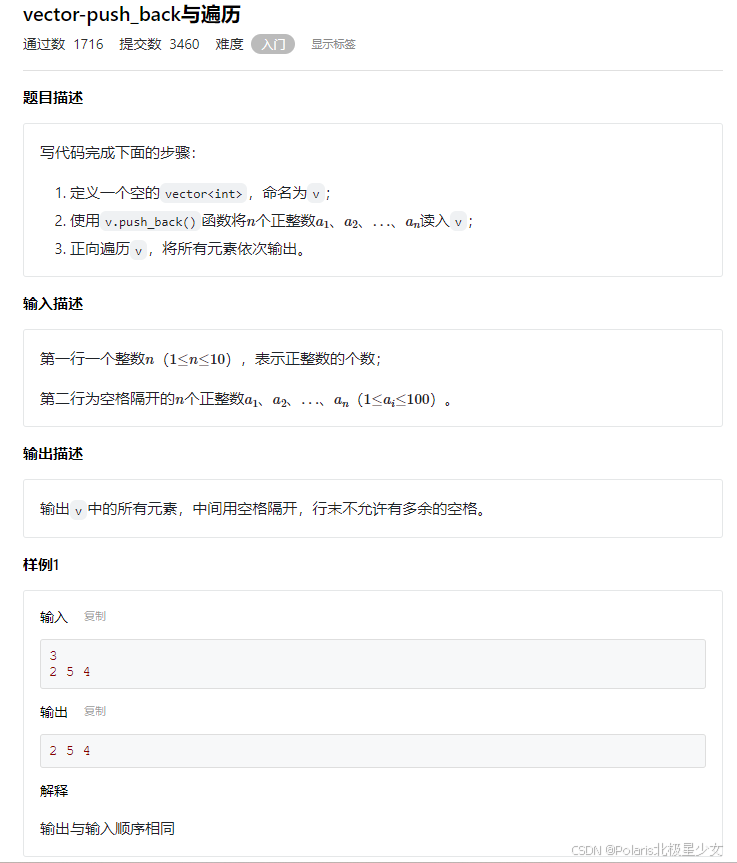
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, x;scanf("%d", &n);vector<int> v;for (int i = 0; i < n; i++) {scanf("%d", &x);v.push_back(x);}for (int i = 0; i < (int)v.size(); i++) {printf("%d", v[i]);if (i < (int)v.size() - 1) {printf(" ");}}return 0;
}
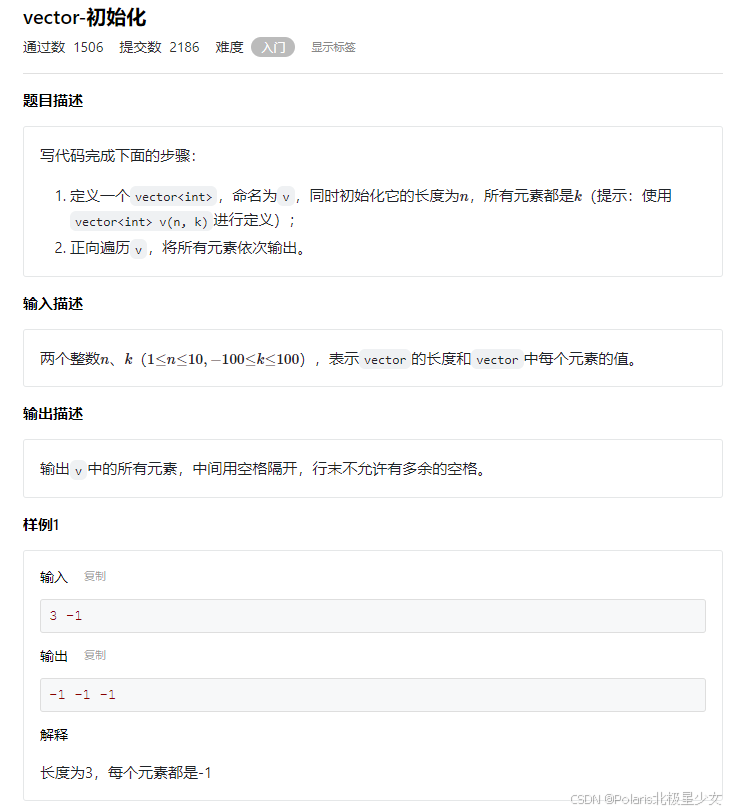
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, k;scanf("%d%d", &n, &k);vector<int> v(n, k);for (int i = 0; i < (int)v.size(); i++) {printf("%d", v[i]);if (i < (int)v.size() - 1) {printf(" ");}}return 0;
}
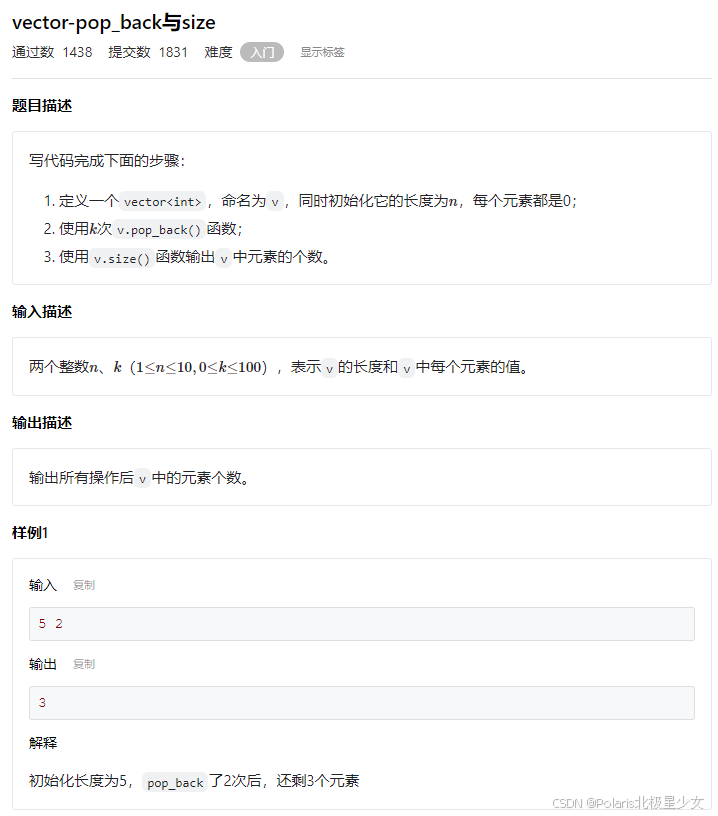
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, k;scanf("%d%d", &n, &k);vector<int> v(n, 0);for (int i = 0; i < k; i++) {v.pop_back();}printf("%d", (int)v.size());return 0;
}
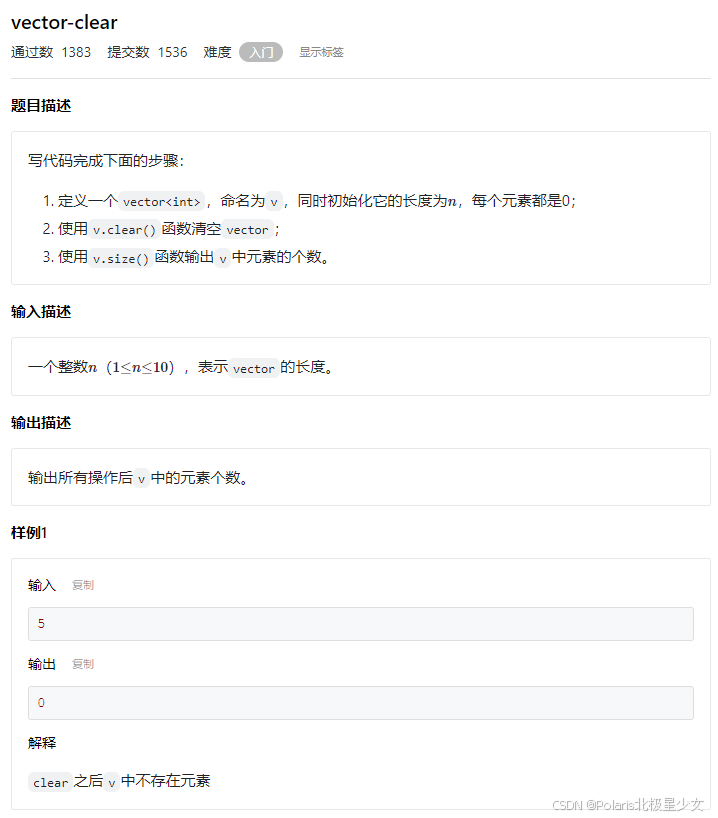
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, k;scanf("%d%d", &n, &k);vector<int> v(n, 0);v.clear();printf("%d", (int)v.size());return 0;
}
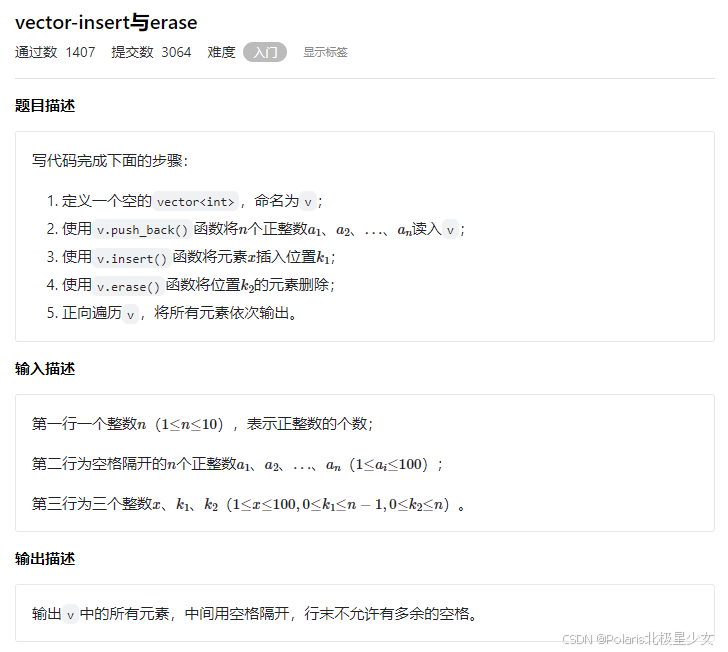
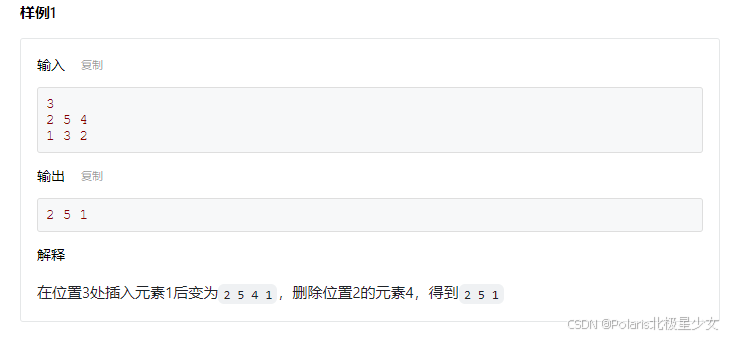
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, x, k1, k2;scanf("%d", &n);vector<int> v;for (int i = 0; i < n; i++) {scanf("%d", &x);v.push_back(x);}scanf("%d%d%d", &x, &k1, &k2);v.insert(v.begin() + k1, x);v.erase(v.begin() + k2);for (int i = 0; i < (int)v.size(); i++) {printf("%d", v[i]);if (i < (int)v.size() - 1) {printf(" ");}}return 0;
}
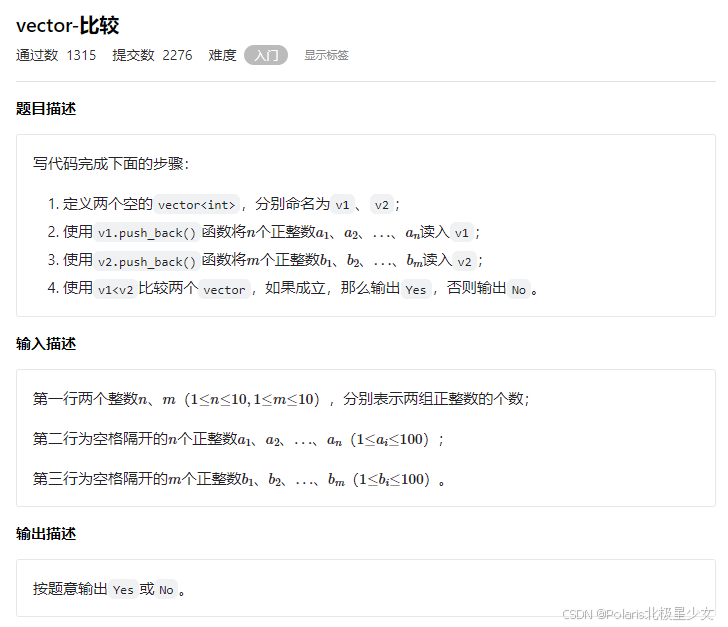
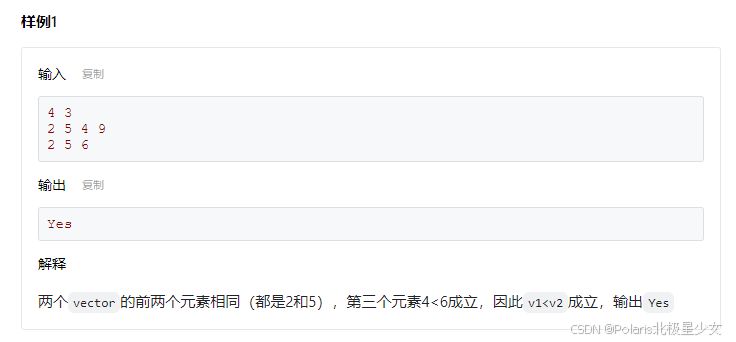
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, m, x;scanf("%d%d", &n, &m);vector<int> v1, v2;for (int i = 0; i < n; i++) {scanf("%d", &x);v1.push_back(x);}for (int i = 0; i < m; i++) {scanf("%d", &x);v2.push_back(x);}printf(v1 < v2 ? "Yes" : "No");return 0;
}
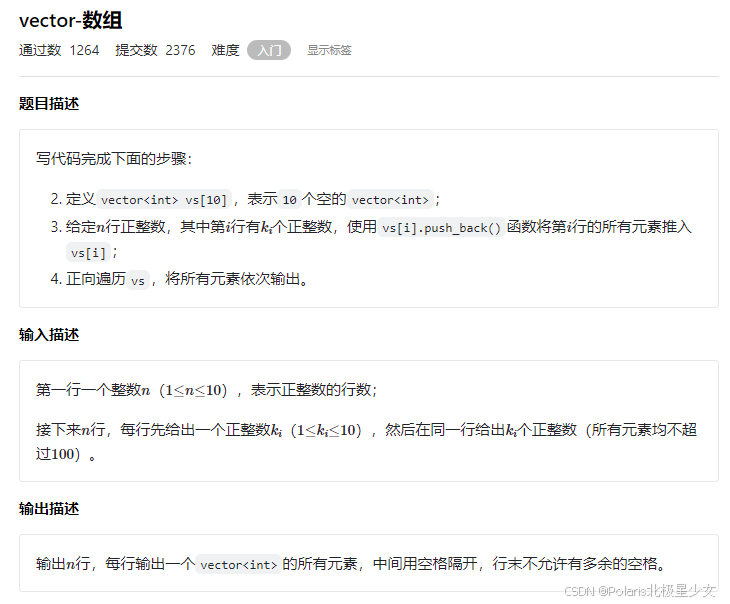
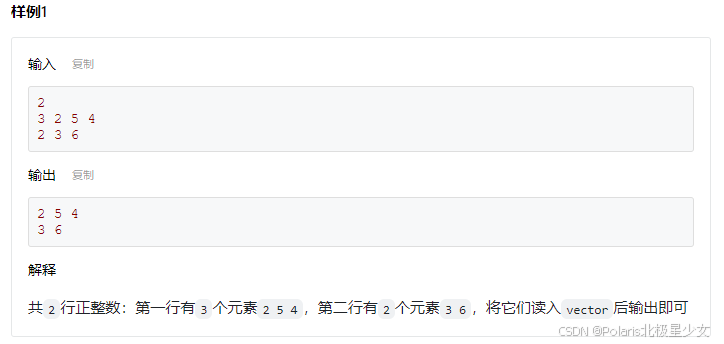
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, k, x;scanf("%d", &n);vector<int> vs[10];for (int i = 0; i < n; i++) {scanf("%d", &k);for (int j = 0; j < k; j++) {scanf("%d", &x);vs[i].push_back(x);}}for (int i = 0; i < n; i++) {for (int j = 0; j < (int)vs[i].size(); j++) {printf("%d", vs[i][j]);if (j < (int)vs[i].size() - 1) {printf(" ");} else {printf("\n");}}}return 0;
}
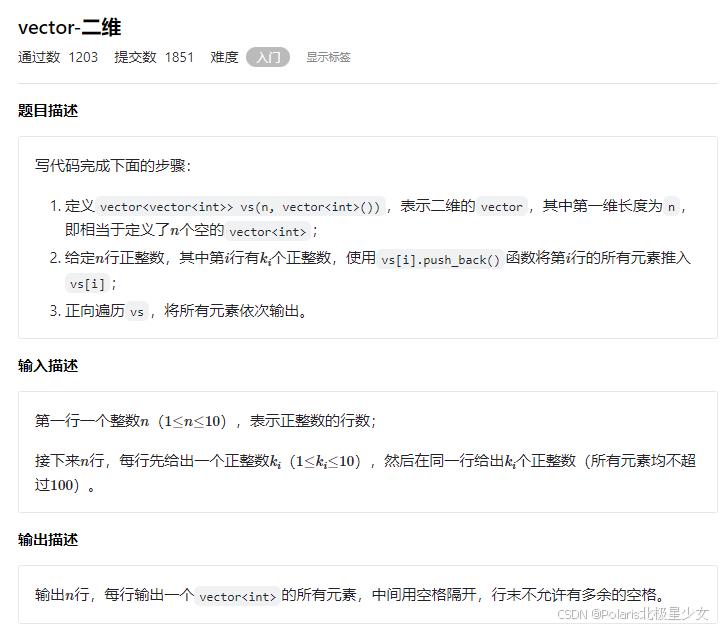
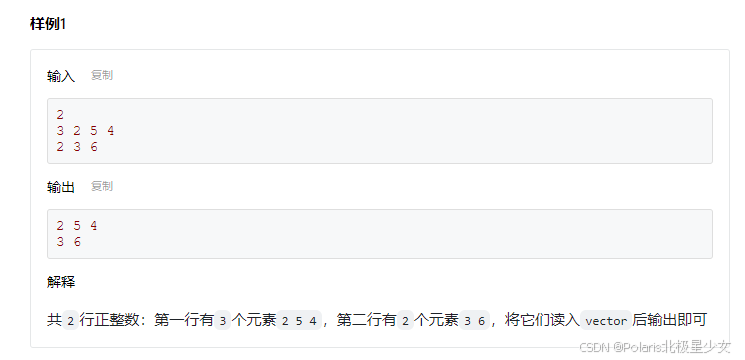
#include <cstdio>
#include <vector>
using namespace std;int main() {int n, k, x;scanf("%d", &n);vector<vector<int> > vs(n, vector<int>());for (int i = 0; i < n; i++) {scanf("%d", &k);for (int j = 0; j < k; j++) {scanf("%d", &x);vs[i].push_back(x);}}for (int i = 0; i < n; i++) {for (int j = 0; j < (int)vs[i].size(); j++) {printf("%d", vs[i][j]);if (j < (int)vs[i].size() - 1) {printf(" ");} else {printf("\n");}}}return 0;
}
6.2set的常见用法详解
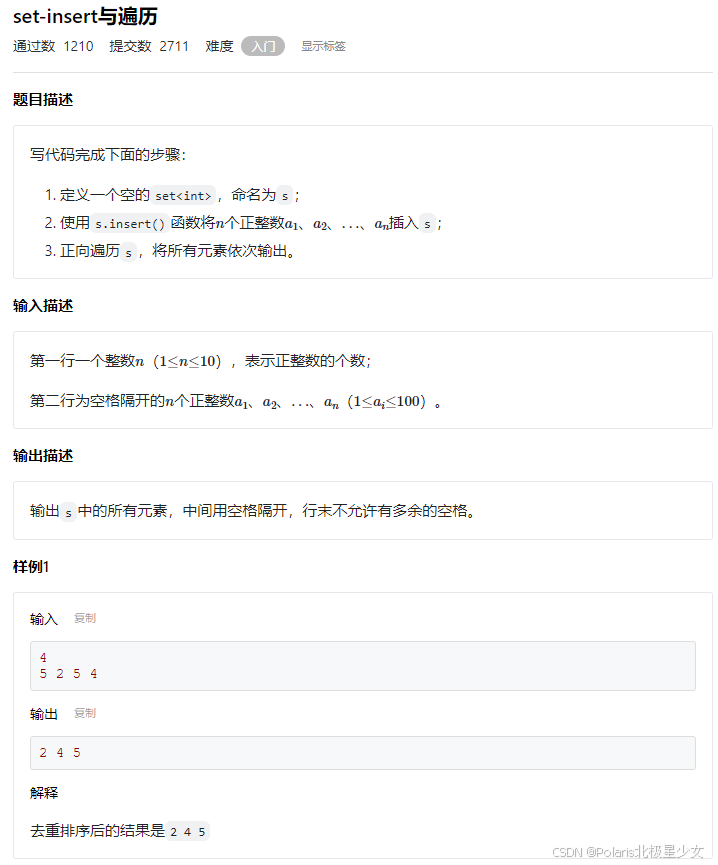
#include <cstdio>
#include <set>
using namespace std;int main() {int n, x;scanf("%d", &n);set<int> s;for (int i = 0; i < n; i++) {scanf("%d", &x);s.insert(x);}for (set<int>::iterator it = s.begin(); it != s.end(); it++) {if (it != s.begin()) {printf(" ");}printf("%d", *it);}return 0;
}
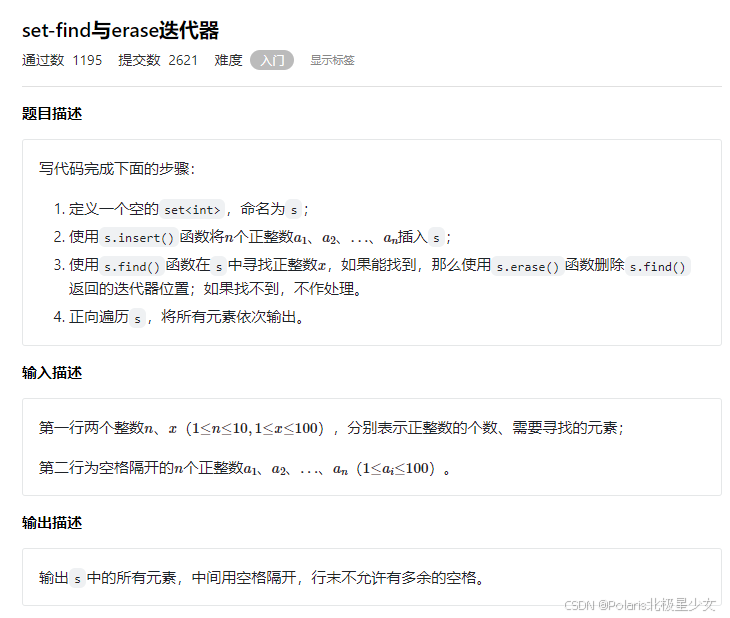
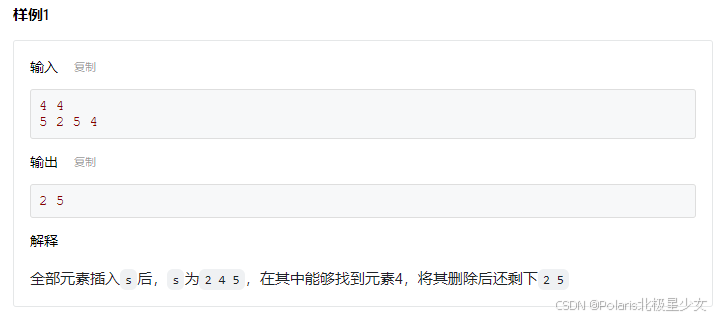
#include <cstdio>
#include <set>
using namespace std;int main() {int n, x;scanf("%d%d", &n, &x);set<int> s;for (int i = 0; i < n; i++) {int a;scanf("%d", &a);s.insert(a);};set<int>::iterator it = s.find(x);if (it != s.end()) {s.erase(it);}for (set<int>::iterator it = s.begin(); it != s.end(); it++) {if (it != s.begin()) {printf(" ");}printf("%d", *it);}return 0;
}
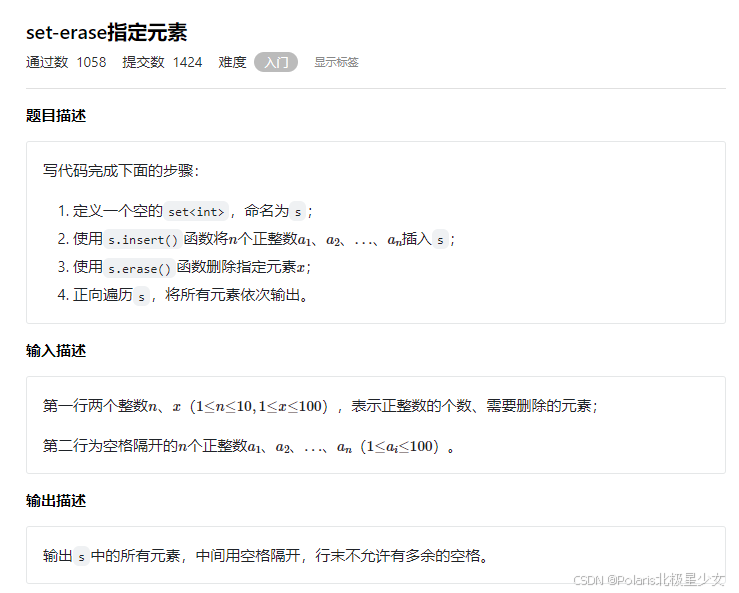
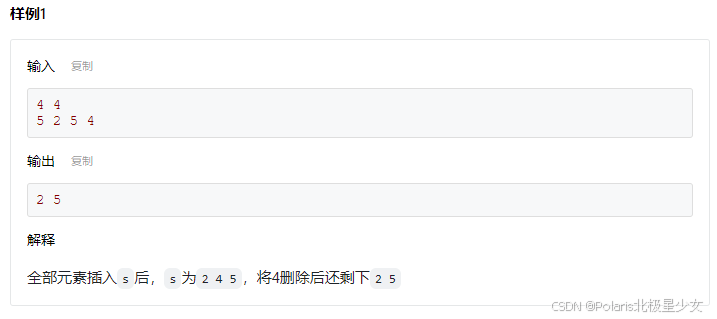
#include <cstdio>
#include <set>
using namespace std;int main() {int n, x;scanf("%d%d", &n, &x);set<int> s;for (int i = 0; i < n; i++) {int a;scanf("%d", &a);s.insert(a);};s.erase(x);for (set<int>::iterator it = s.begin(); it != s.end(); it++) {if (it != s.begin()) {printf(" ");}printf("%d", *it);}return 0;
}
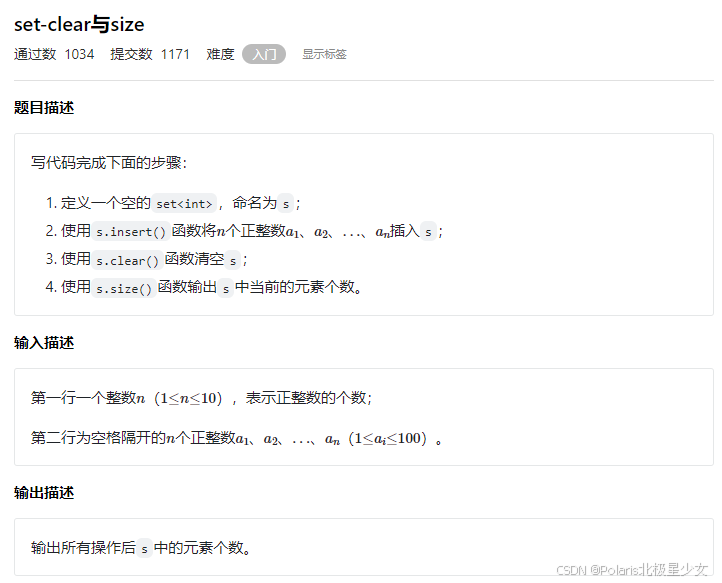
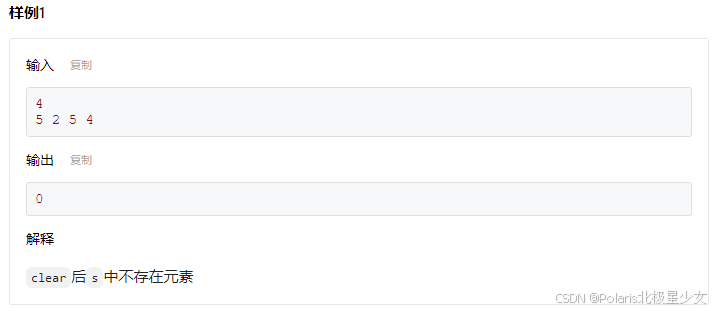
#include <cstdio>
#include <set>
using namespace std;int main() {int n, x;scanf("%d%d", &n, &x);set<int> s;for (int i = 0; i < n; i++) {int a;scanf("%d", &a);s.insert(a);};s.clear();printf("%d", (int)s.size());return 0;
}
6.3string的常见用法详解
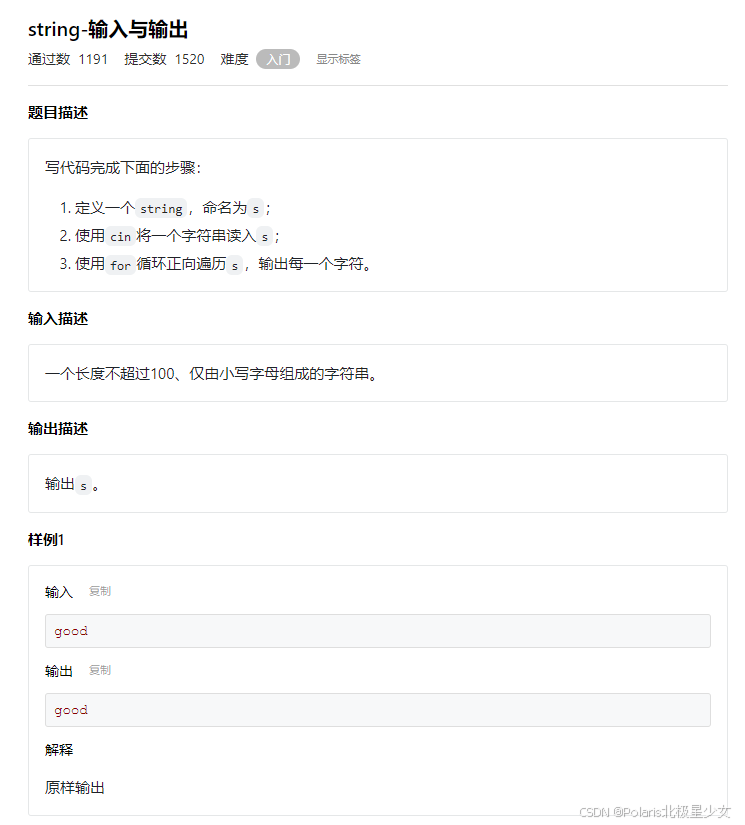
#include <iostream>
#include <string>
using namespace std;int main () {string s;cin >> s;for (int i = 0; i < s.length(); i++) {cout << s[i];}return 0;
}
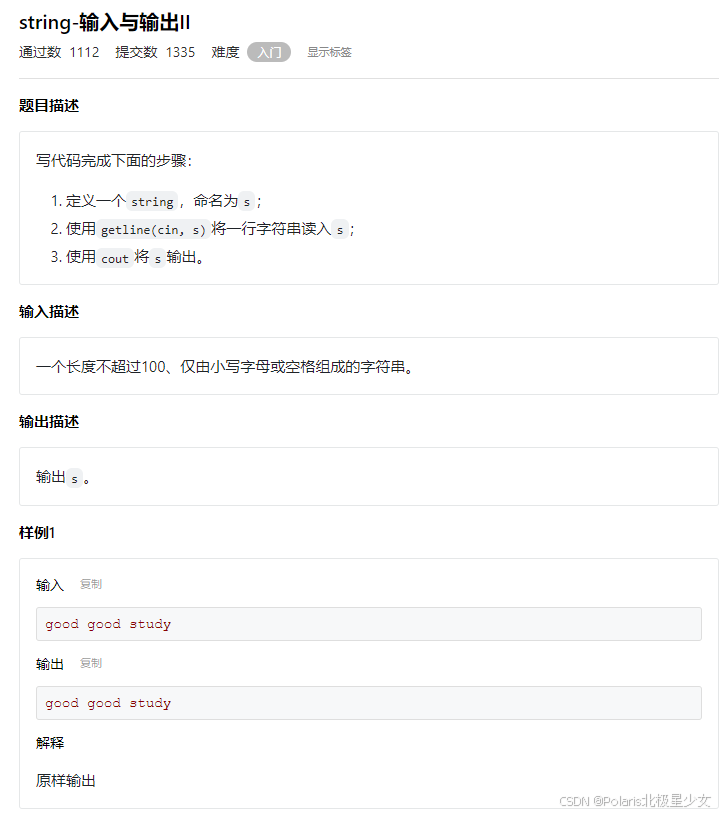
#include <iostream>
#include <string>
using namespace std;int main () {string s;getline(cin, s);for (int i = 0; i < s.length(); i++) {cout << s[i];}return 0;
}
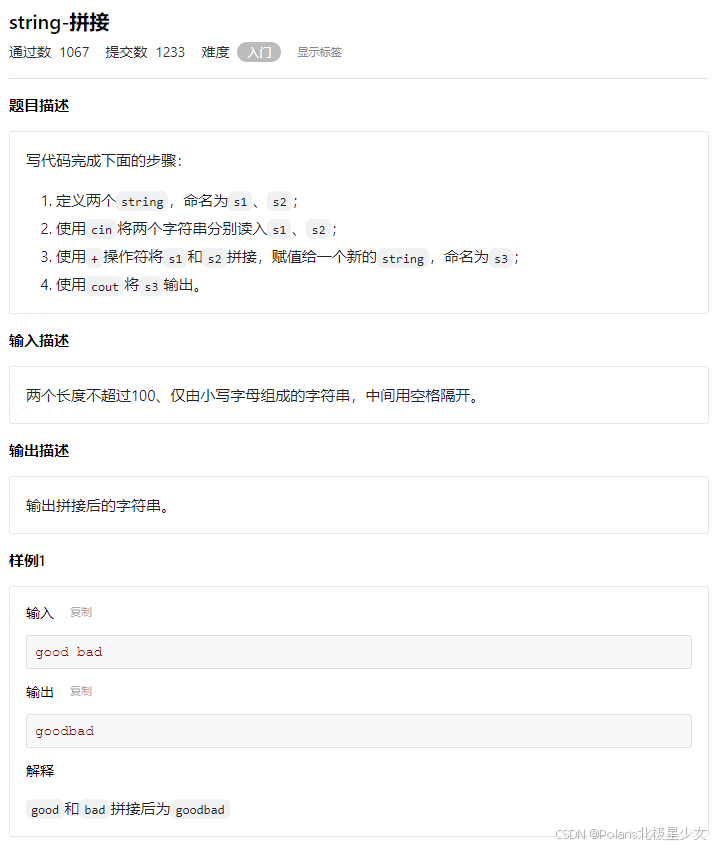
#include <iostream>
#include <string>
using namespace std;int main () {string s1,s2;cin>>s1>>s2;string s3=s1+s2;for (int i = 0; i < s3.length(); i++) {cout << s3[i];}return 0;
}
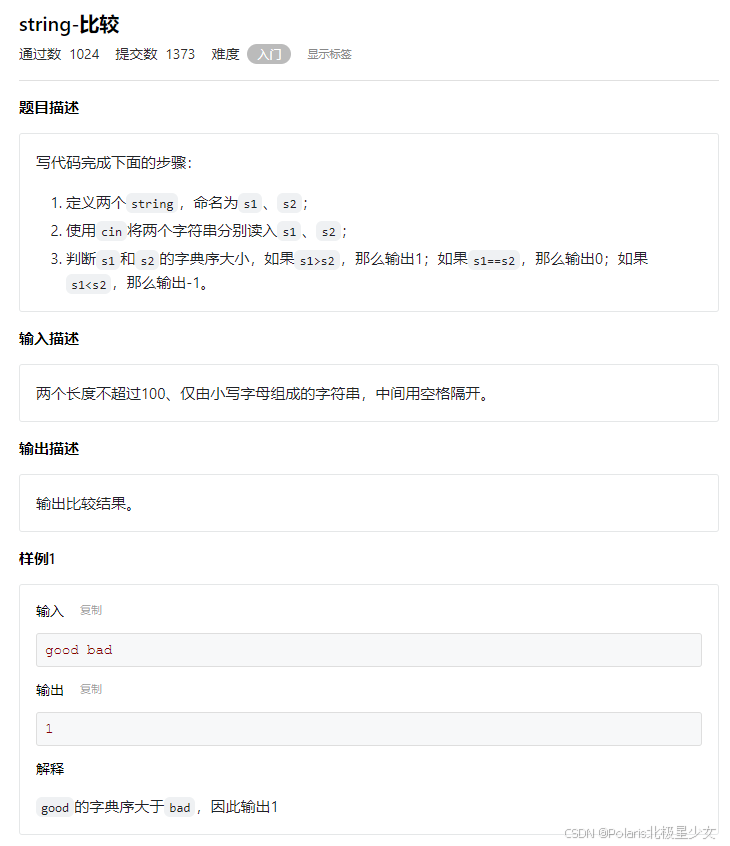
#include <iostream>
#include <string>
using namespace std;int main () {string s1,s2;cin>>s1>>s2;if(s1>s2)printf("1");else if(s1==s2)printf("0");else printf("-1");return 0;
}
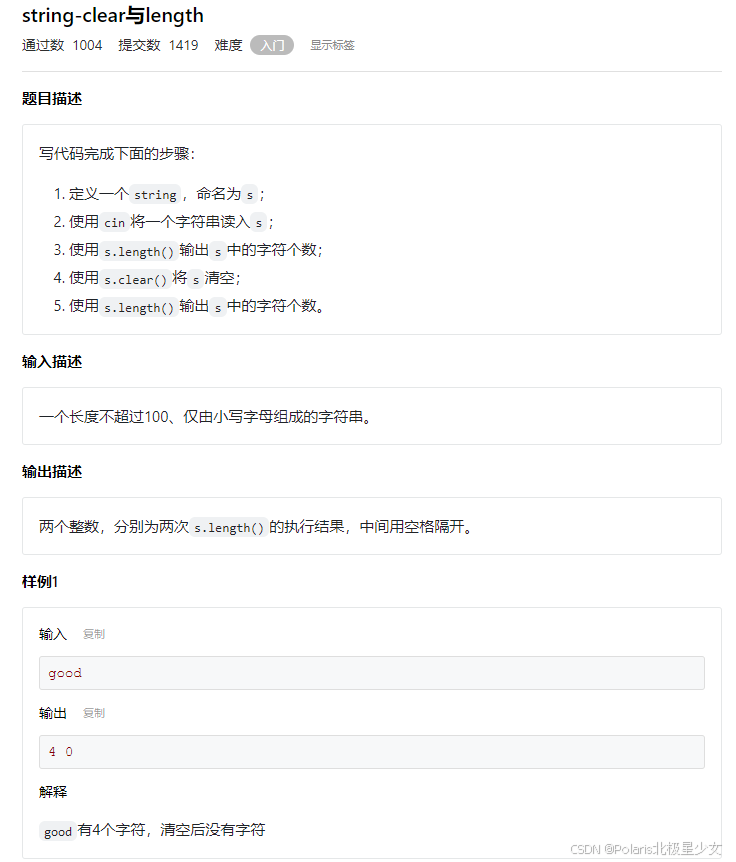
#include <iostream>
#include <string>
using namespace std;int main () {string s;cin>>s;printf("%d ",s.length());s.clear();printf("%d",s.length());return 0;
}
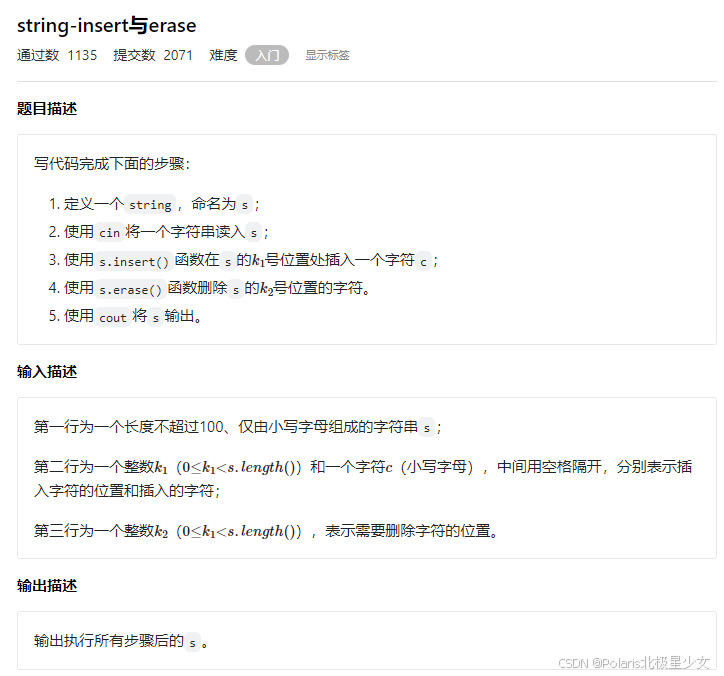
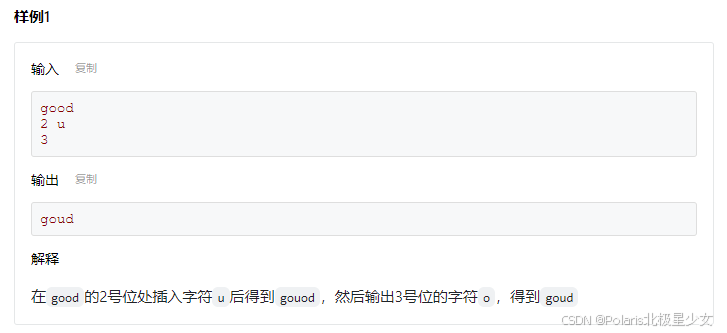
#include <iostream>
#include <string>
using namespace std;int main () {string s;cin >> s;int k1, k2;char c;cin >> k1 >> c >> k2;s.insert(s.begin() + k1, c);s.erase(s.begin() + k2);cout << s;return 0;
}
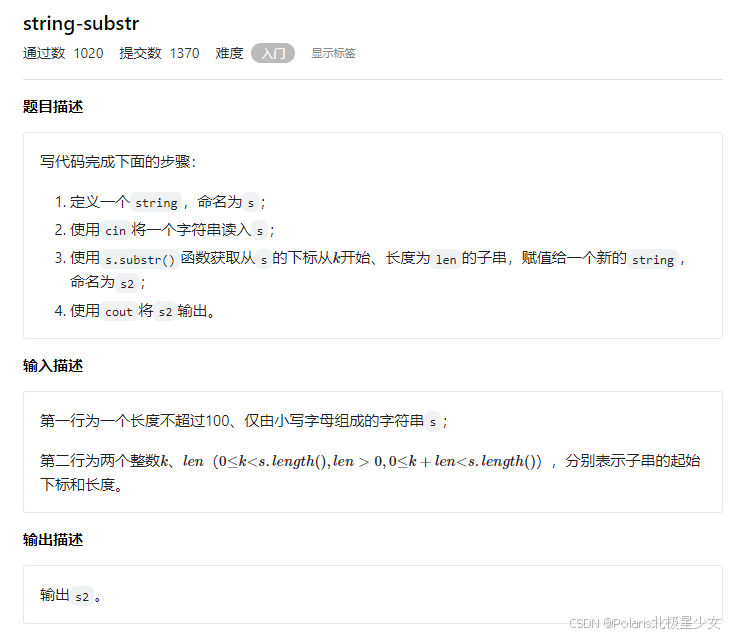
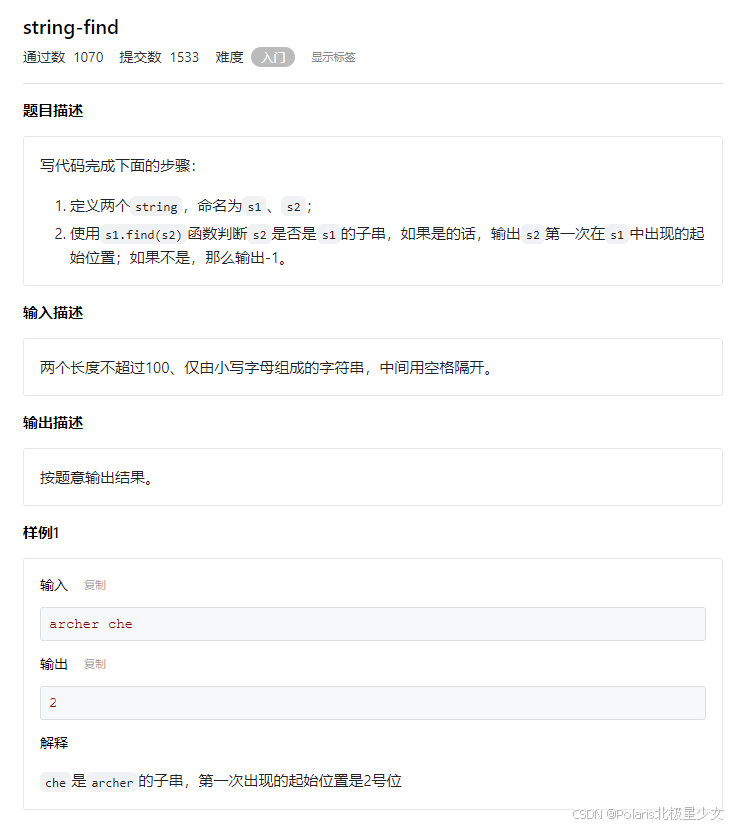
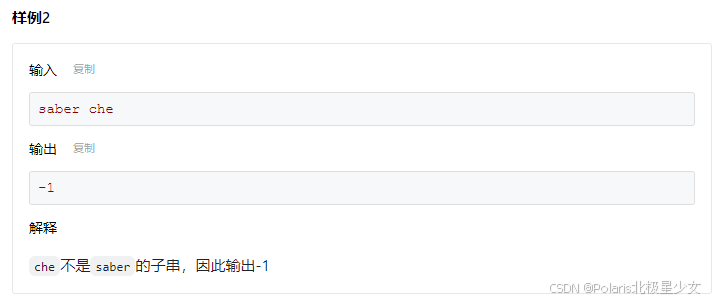
#include <iostream>
#include <string>
using namespace std;int main () {string s1,s2;cin >> s1>>s2;printf("%d",s1.find(s2));return 0;
}
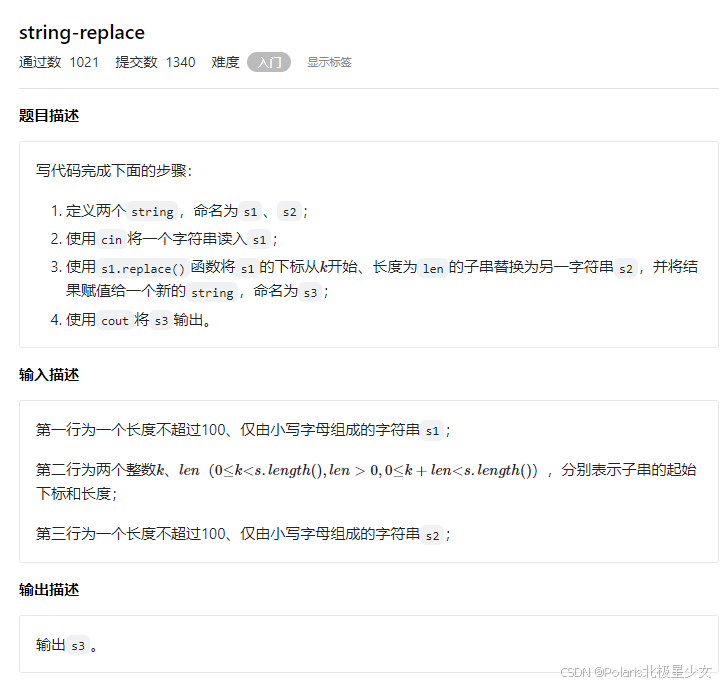
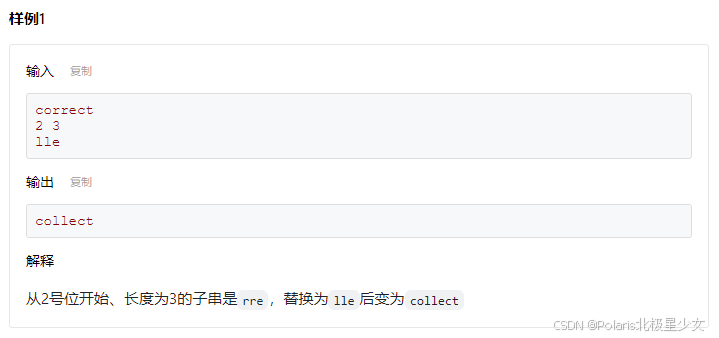
#include <iostream>
#include <string>
using namespace std;int main () {string s1,s2;cin >> s1;int k,len;cin>>k>>len;cin>>s2;string s3=s1.replace(k,len,s2);cout<<s3;return 0;
}
6.4map的常见用法详解
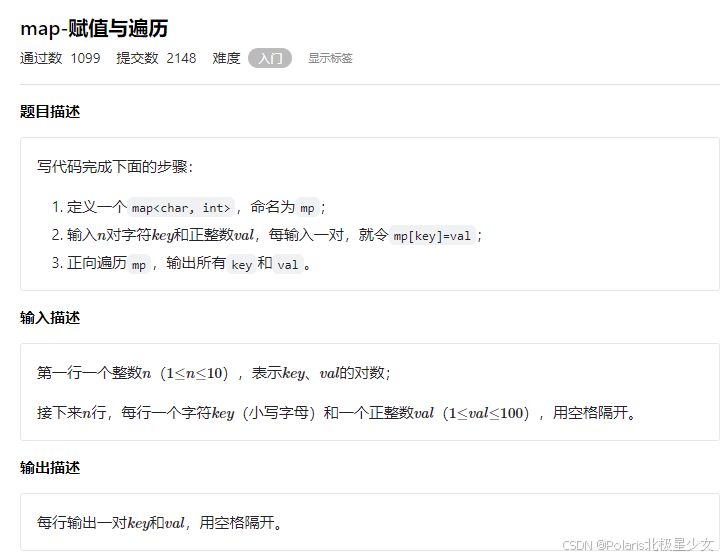
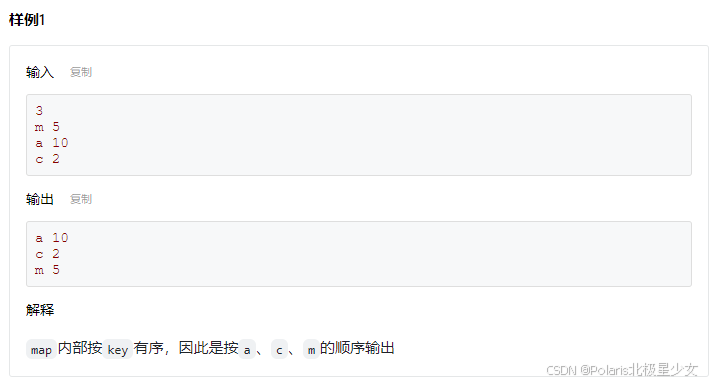
#include <iostream>
#include <map>
using namespace std;int main() {int n, x;char c;cin >> n;map<char, int> mp;for (int i = 0; i < n; i++) {cin >> c >> x;mp[c] = x;}for (map<char, int>::iterator it = mp.begin(); it != mp.end(); it++) {cout << it -> first << " " << it -> second << endl;}return 0;
}
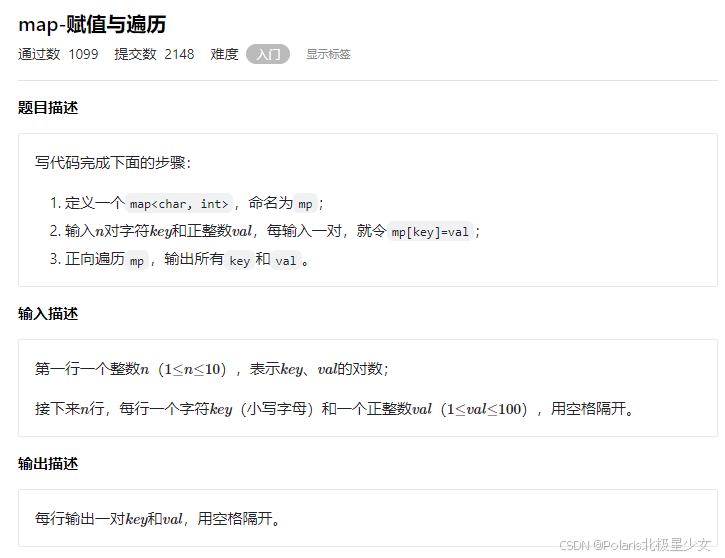
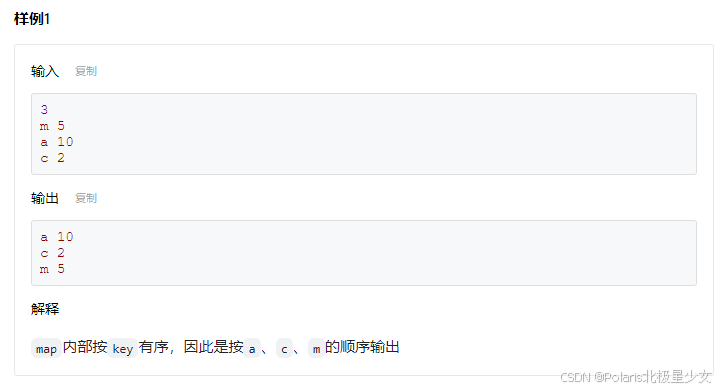
#include <iostream>
#include <map>
using namespace std;int main() {int n, x;char c;cin >> n;map<char, int> mp;for (int i = 0; i < n; i++) {cin >> c >> x;mp[c] = x;}for (map<char, int>::iterator it = mp.begin(); it != mp.end(); it++) {cout << it -> first << " " << it -> second << endl;}return 0;
}
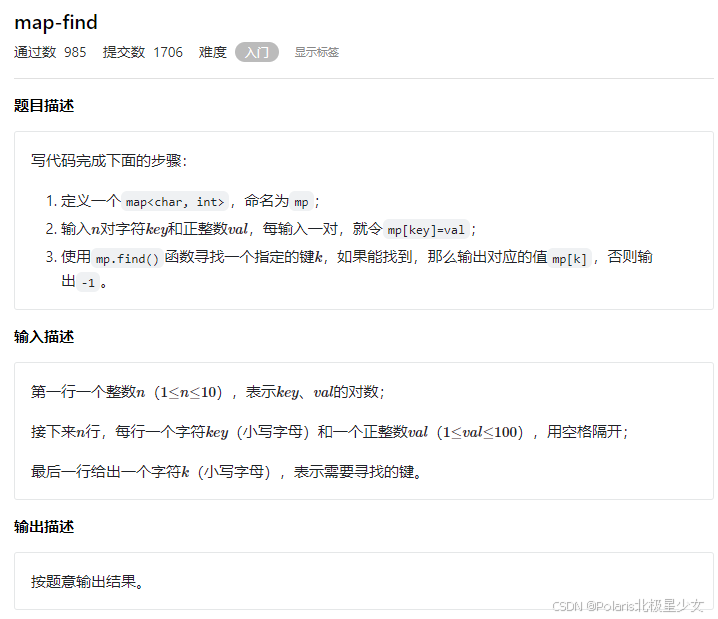
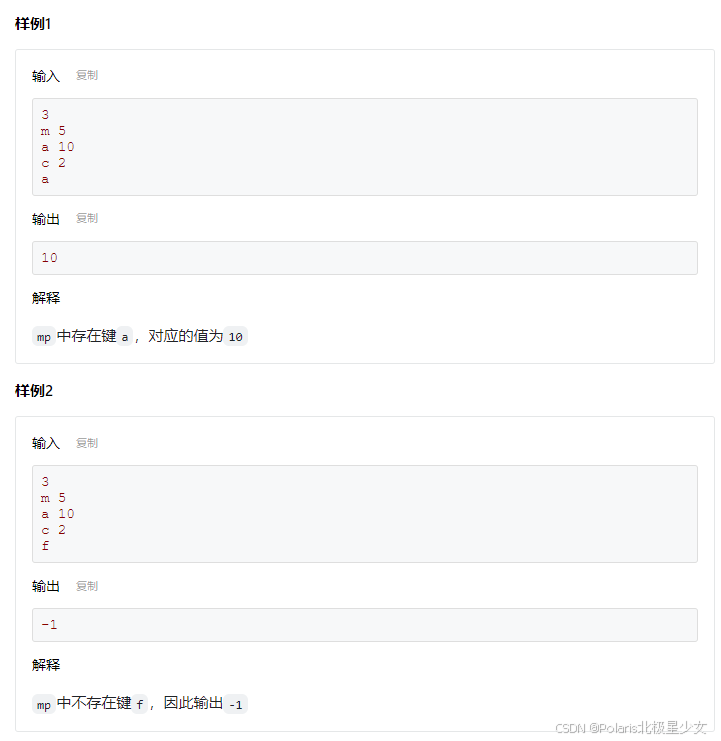
#include <iostream>
#include <map>
using namespace std;int main() {int n, x;char c;cin >> n;map<char, int> mp;for (int i = 0; i < n; i++) {cin >> c >> x;mp[c] = x;}char k;cin>>k;if (mp.find(k) != mp.end()) {cout << mp[k];} else {cout << -1;}return 0;
}
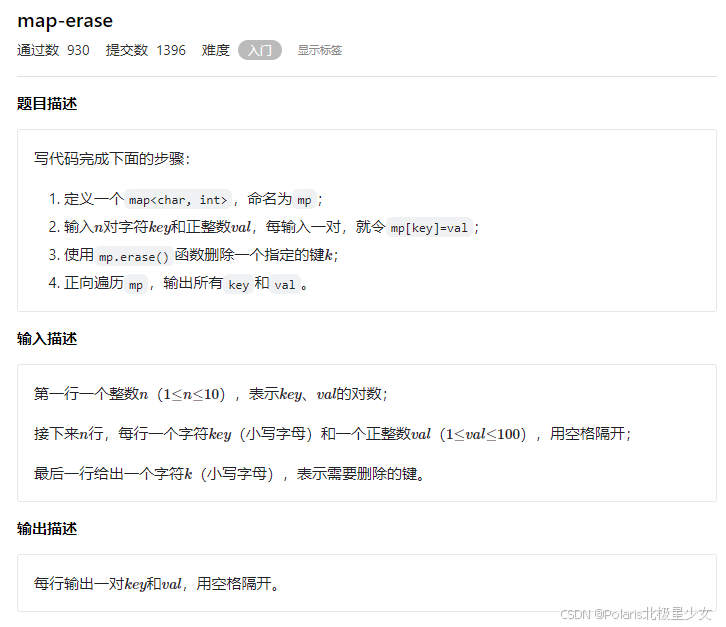
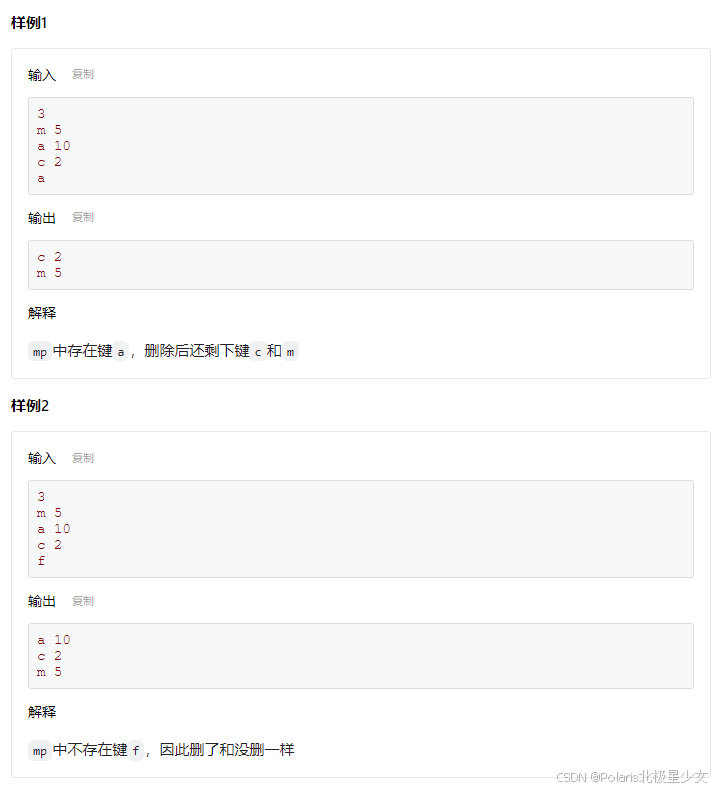
#include <iostream>
#include <map>
using namespace std;int main() {int n, x;char c;cin >> n;map<char, int> mp;for (int i = 0; i < n; i++) {cin >> c >> x;mp[c] = x;}char k;cin>>k;mp.erase(k); for (map<char, int>::iterator it = mp.begin(); it != mp.end(); it++) {cout << it -> first << " " << it -> second << endl;}return 0;
}
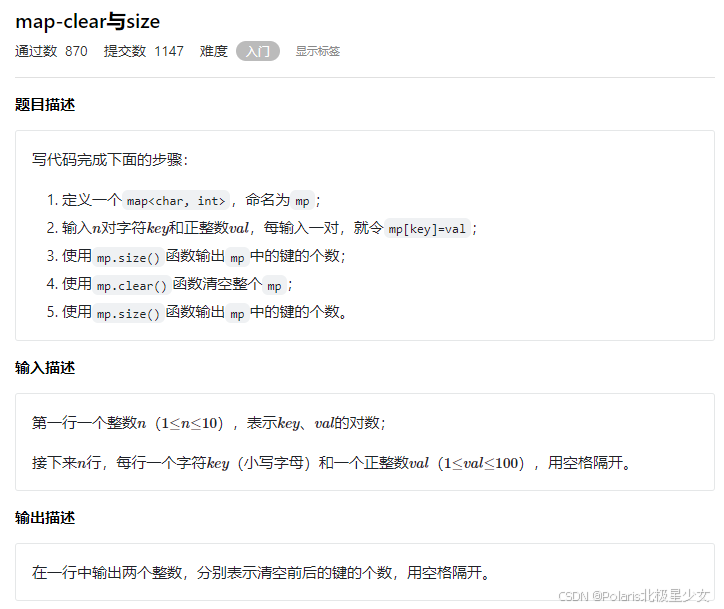
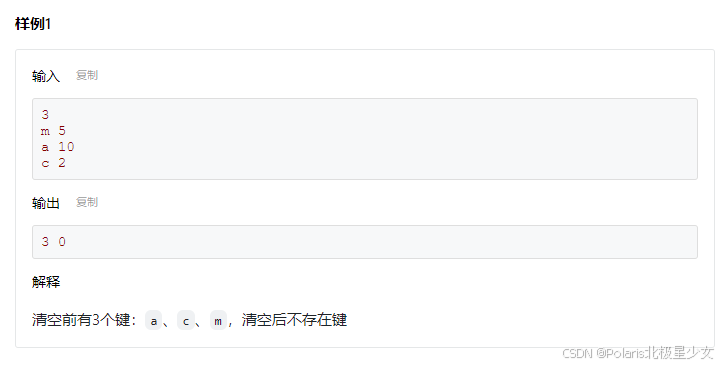
#include <iostream>
#include <map>
using namespace std;int main() {int n, x;char c;cin >> n;map<char, int> mp;for (int i = 0; i < n; i++) {cin >> c >> x;mp[c] = x;}cout<<mp.size()<<" ";mp.clear();cout<<mp.size();return 0;
}
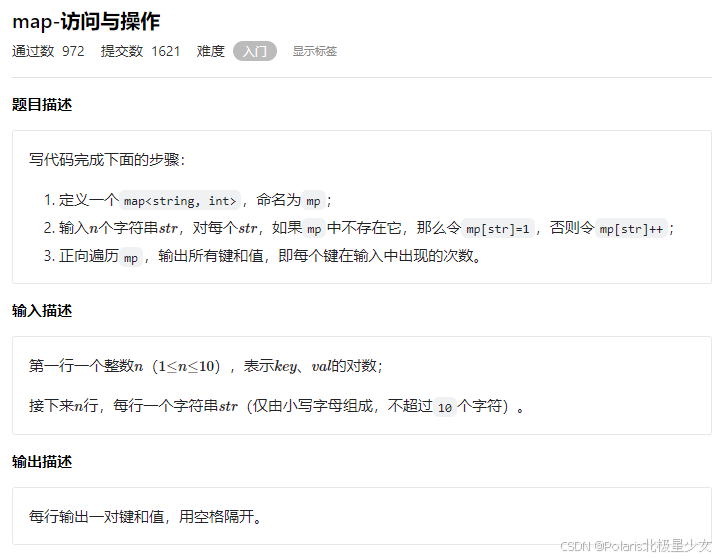
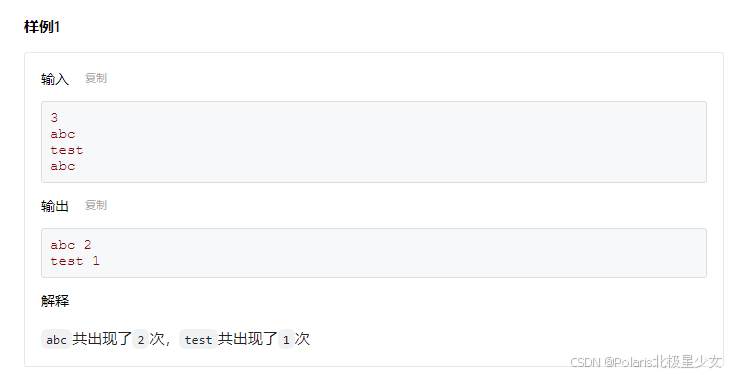
#include <iostream>
#include <map>
using namespace std;int main() {int n;string str;cin >> n;map<string, int> mp;for (int i = 0; i < n; i++) {cin >> str;if (mp.find(str) == mp.end()) {mp[str] = 1;} else {mp[str]++;}}for (map<string, int>::iterator it = mp.begin(); it != mp.end(); it++) {cout << it -> first << " " << it -> second << endl;}return 0;
}
6.5queue的常见用法详解
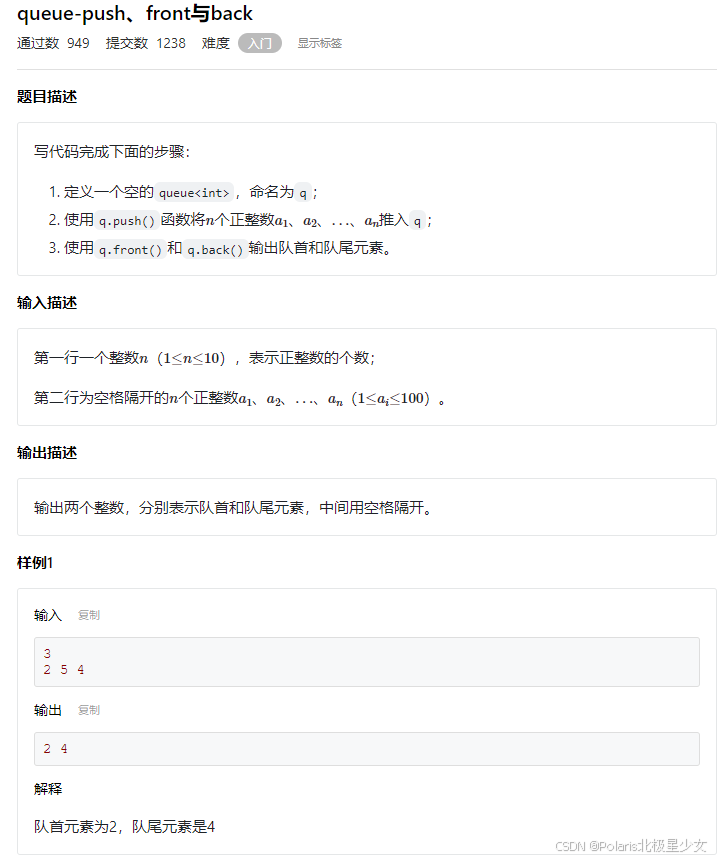
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x;cin >> n;queue<int> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}cout << q.front() << " " << q.back();return 0;
}
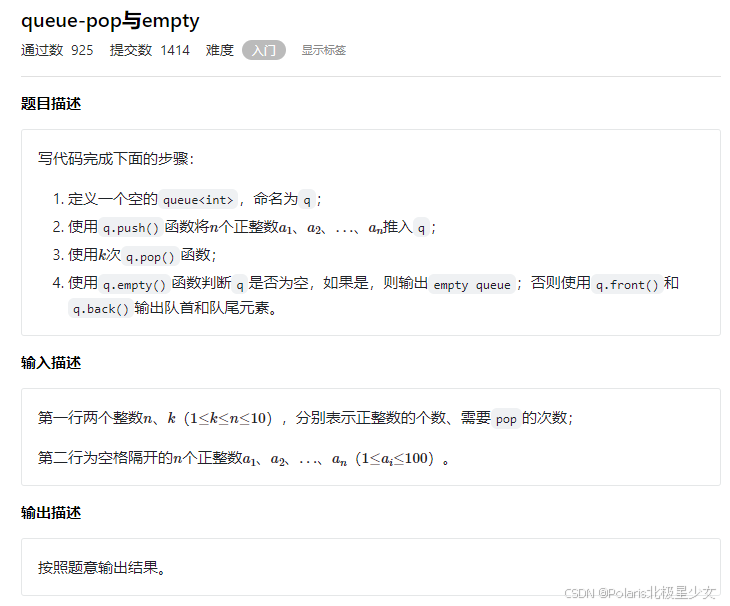
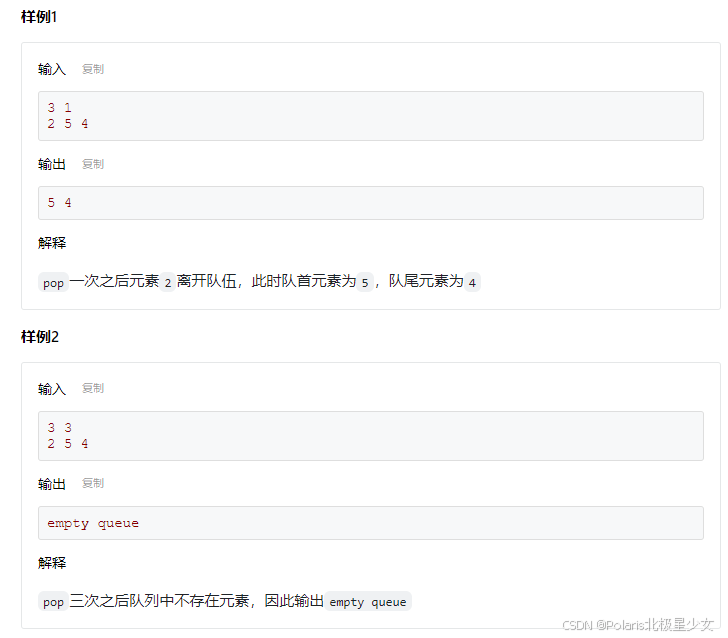
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x,k;cin >> n;cin>>k;queue<int> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}for (int i = 0; i < k; i++) {q.pop();}if (q.empty()) {cout << "empty queue";} else {cout << q.front() << " " << q.back();}return 0;
}
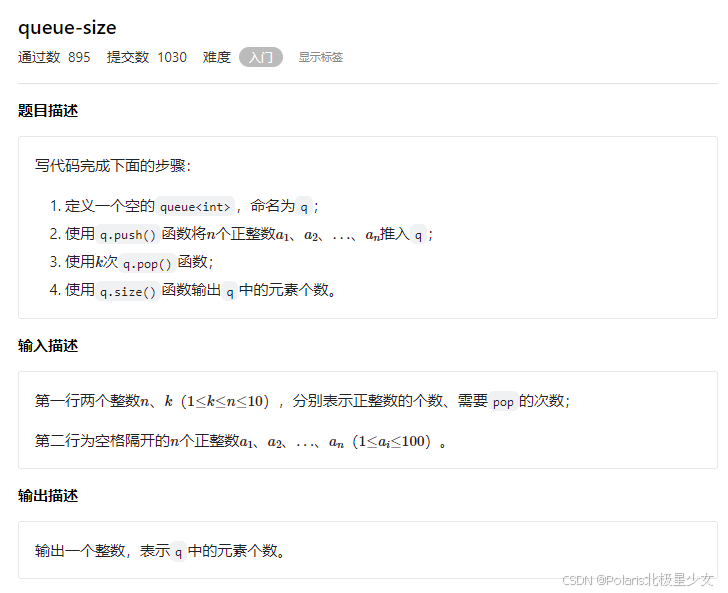
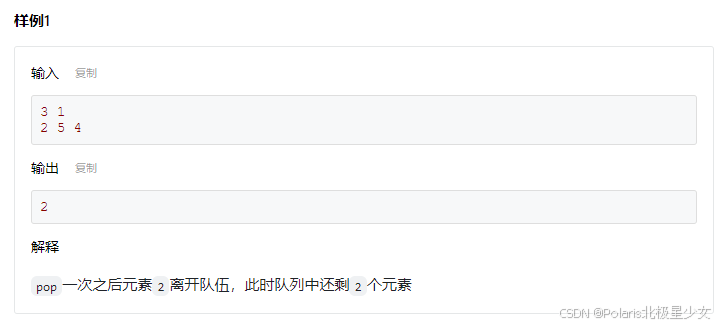
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x,k;cin >> n;cin>>k;queue<int> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}for (int i = 0; i < k; i++) {q.pop();}cout<<q.size();return 0;
}
6.6priority_queue的常见用法详解
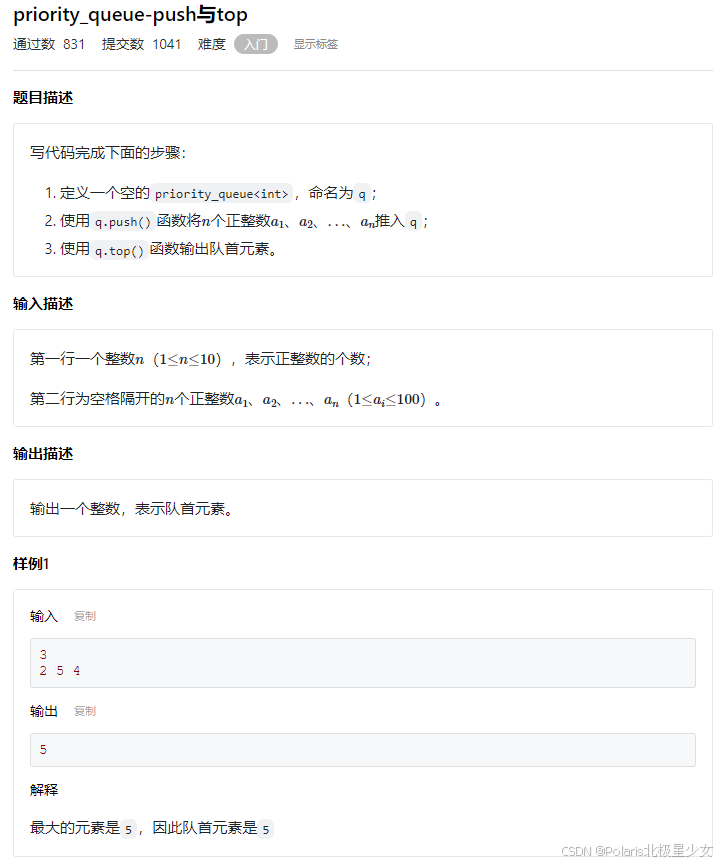
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x;cin >> n;priority_queue<int> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}cout << q.top();return 0;
}
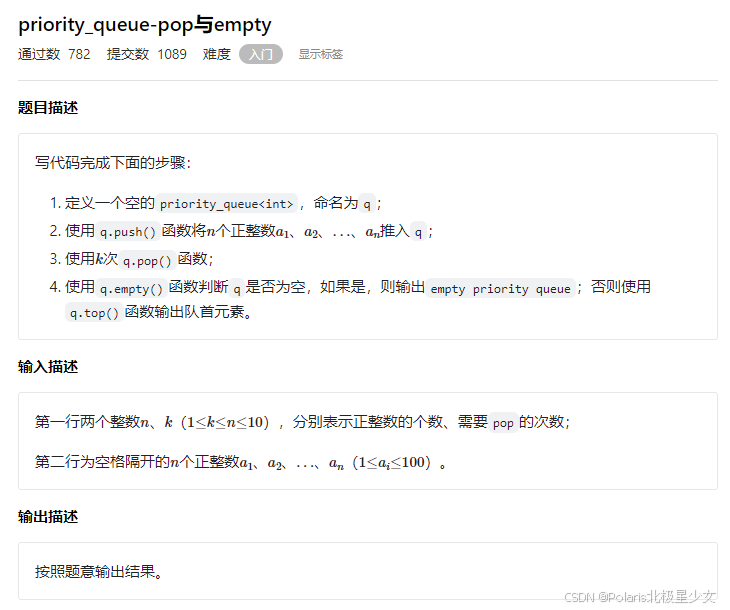
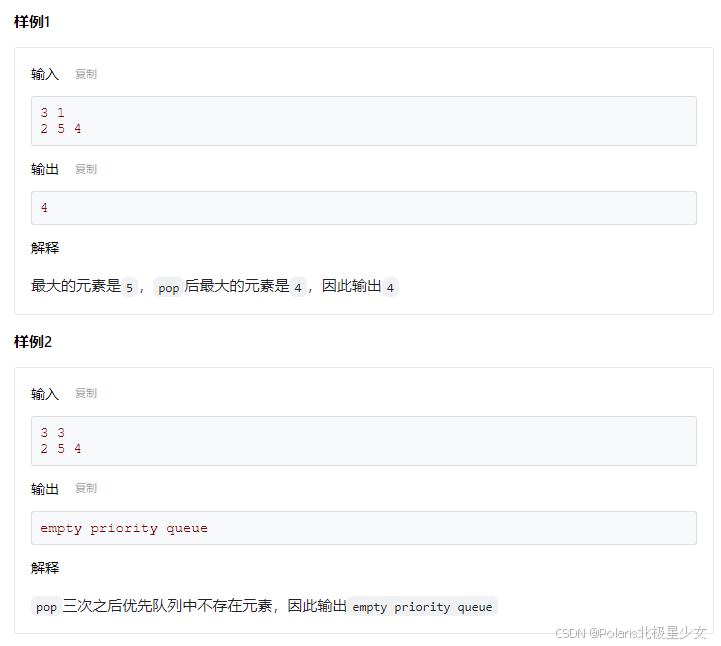
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x,k;cin >> n>>k;priority_queue<int> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}for (int i = 0; i < k; i++) {q.pop();}if(q.empty()){cout<<"empty priority queue";}else{cout<<q.top();}return 0;
}
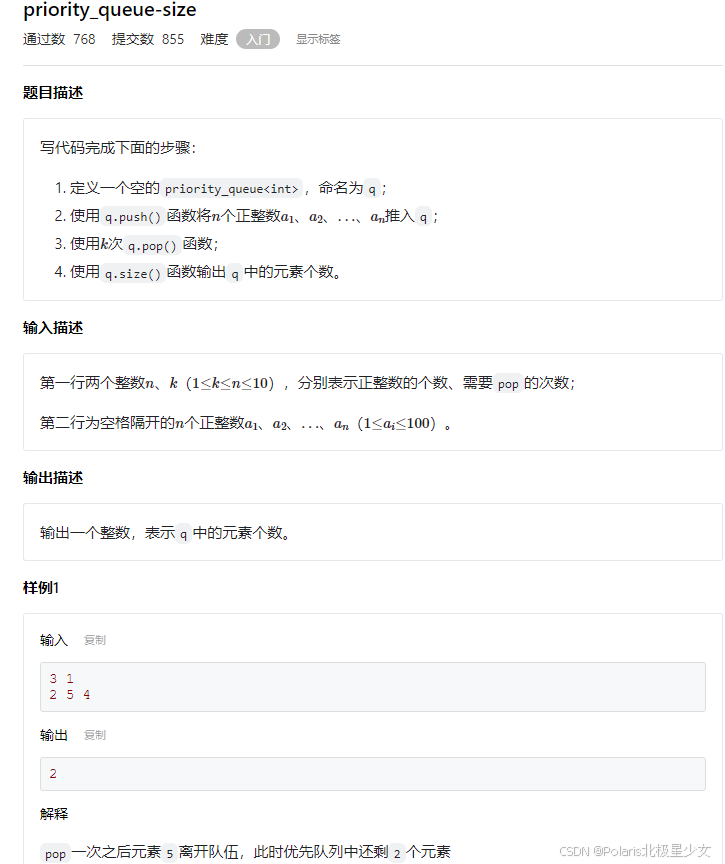
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x,k;cin >> n>>k;priority_queue<int> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}for (int i = 0; i < k; i++) {q.pop();}cout<<q.size();return 0;
}
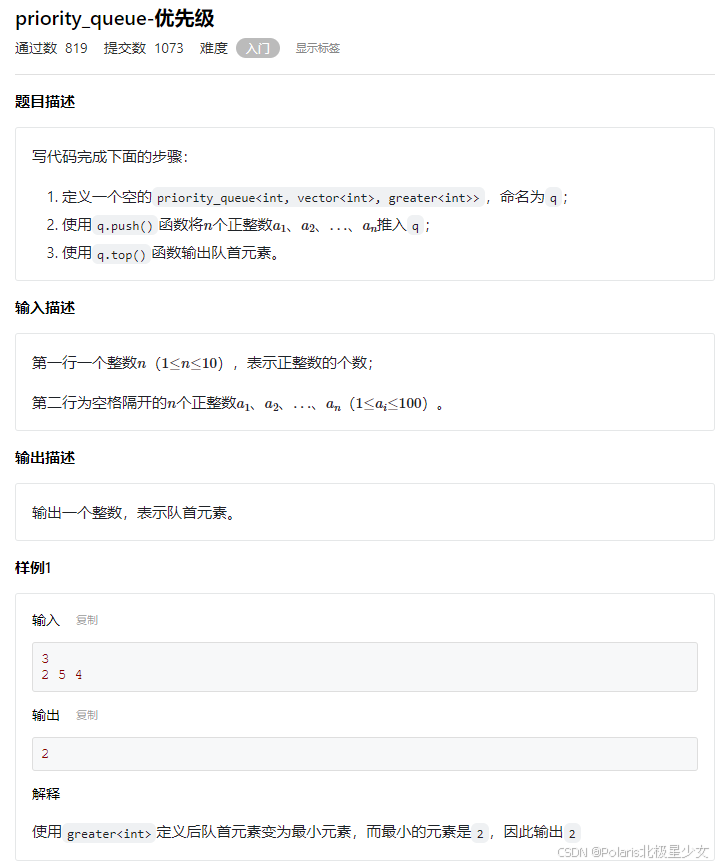
#include <iostream>
#include <queue>
using namespace std;int main() {int n, x;cin >> n;priority_queue<int, vector<int>, greater<int>> q;for (int i = 0; i < n; i++) {cin >> x;q.push(x);}cout<<q.top();return 0;
}
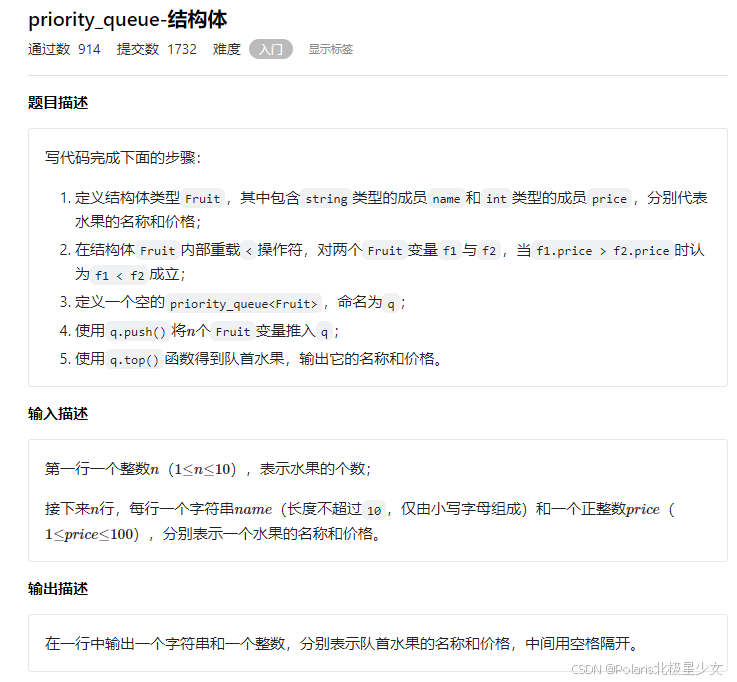
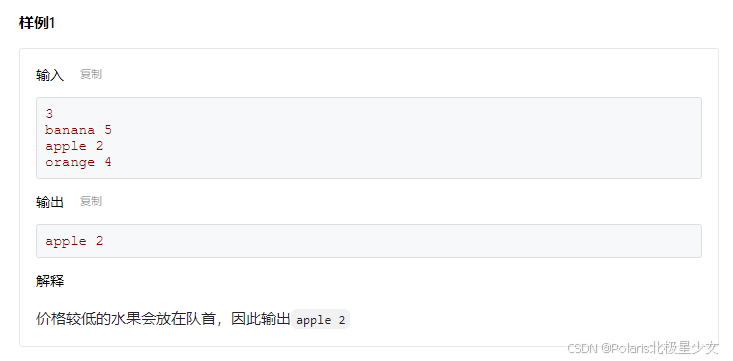
#include <iostream>
#include <queue>
using namespace std;
struct Fruit {string name;int price;Fruit(string _name, int _price) {name = _name;price = _price;}bool operator<(const Fruit& other) const {return price > other.price;}
};int main() {int n, price;string name;cin >> n;priority_queue<Fruit> q;for (int i = 0; i < n; i++) {cin >> name >> price;q.push(Fruit(name, price));}Fruit topFruit = q.top();cout << topFruit.name << " " << topFruit.price;return 0;
}
6.7stack的常见用法详解
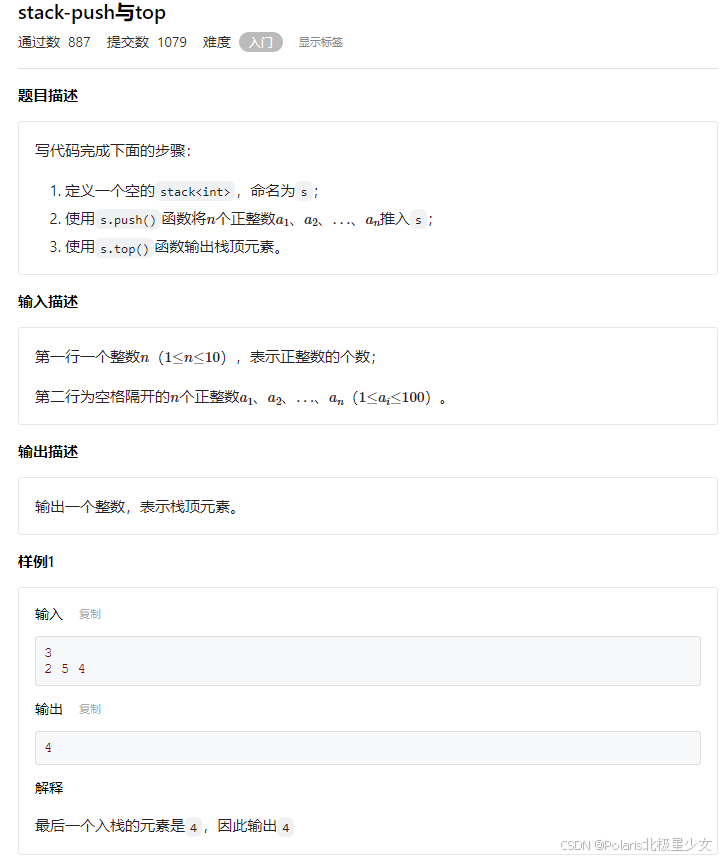
#include <iostream>
#include <stack>
using namespace std;int main() {int n, x;cin >> n;stack<int> s;for (int i = 0; i < n; i++) {cin >> x;s.push(x);}cout << s.top();return 0;
}
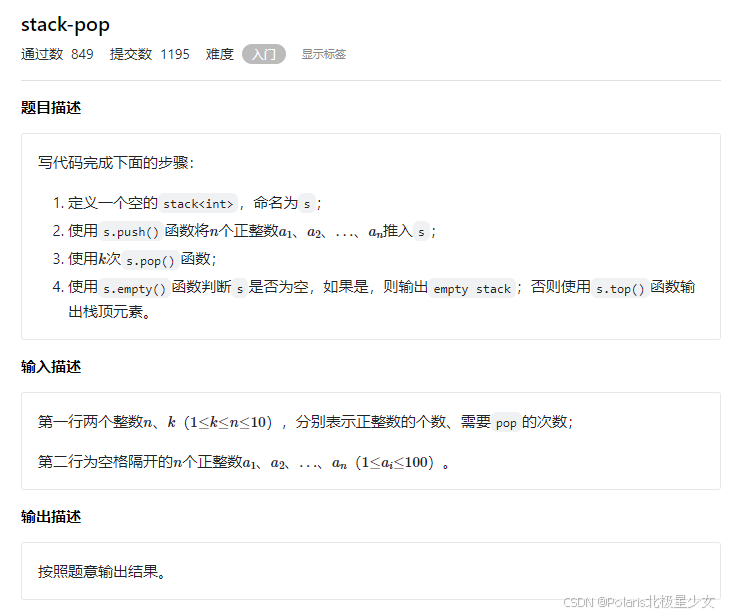
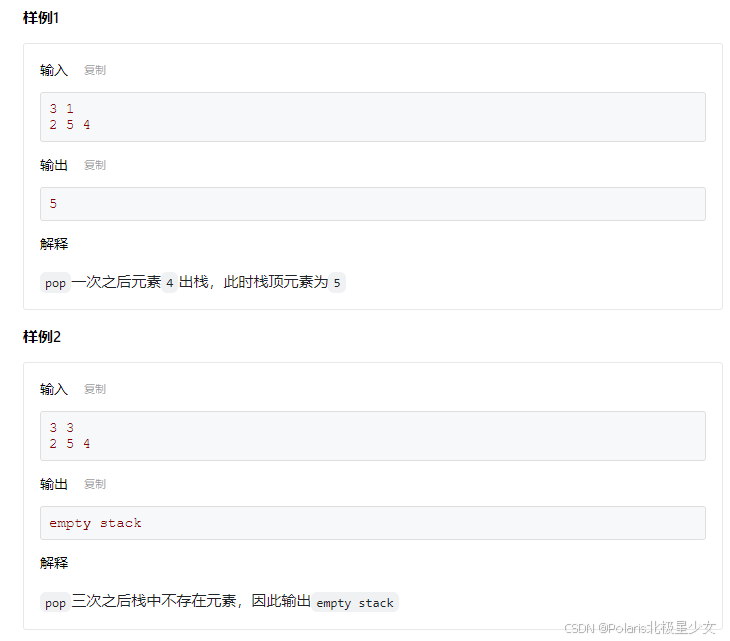
#include <iostream>
#include <stack>
using namespace std;int main() {int n, x,k;cin >> n>>k;stack<int> s;for (int i = 0; i < n; i++) {cin >> x;s.push(x);}for (int i = 0; i < k; i++) {s.pop();}if(s.empty()){cout<<"empty stack";}else{cout << s.top();}return 0;
}
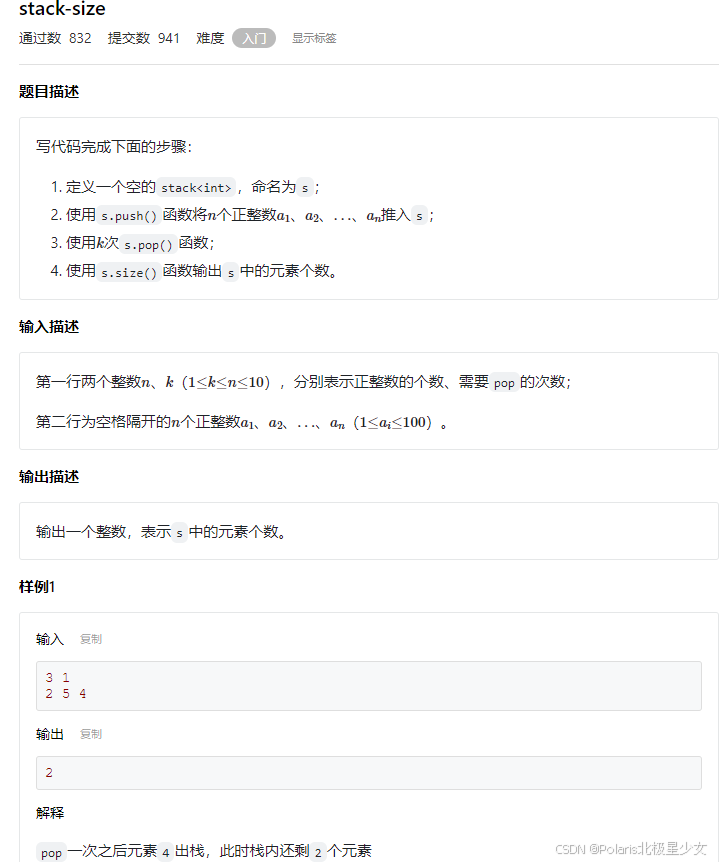
#include <iostream>
#include <stack>
using namespace std;int main() {int n, x,k;cin >> n>>k;stack<int> s;for (int i = 0; i < n; i++) {cin >> x;s.push(x);}for (int i = 0; i < k; i++) {s.pop();}cout<<s.size();return 0;
}
6.8pair的常见用法详解
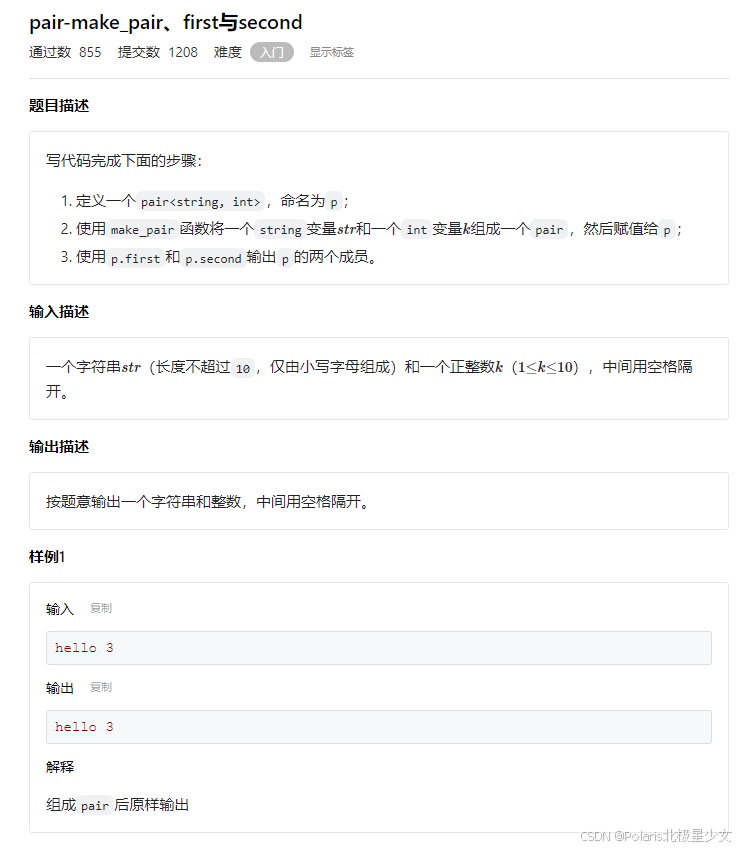
#include <iostream>
#include <utility>
using namespace std;int main() {string str;int k;cin >> str >> k;pair<string, int> p = make_pair(str, k);cout << p.first << " " << p.second;return 0;
}
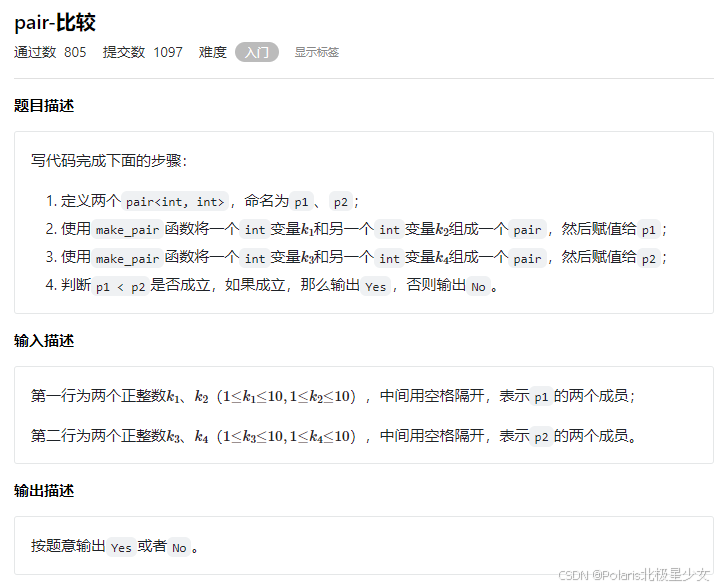
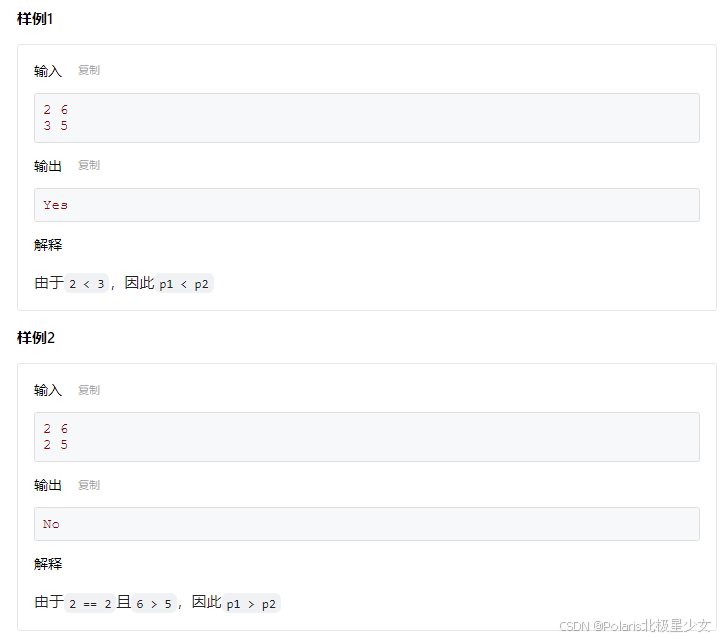
#include <iostream>
#include <utility>
using namespace std;int main() {string str;int k1,k2,k3,k4;cin>>k1>>k2>>k3>>k4;pair<int, int> p1 = make_pair(k1, k2);pair<int, int> p2 = make_pair(k3, k4);if(p1<p2){cout<<"Yes";}else{cout<<"No";}return 0;
}
6.9algorithm头文件下的常用函数
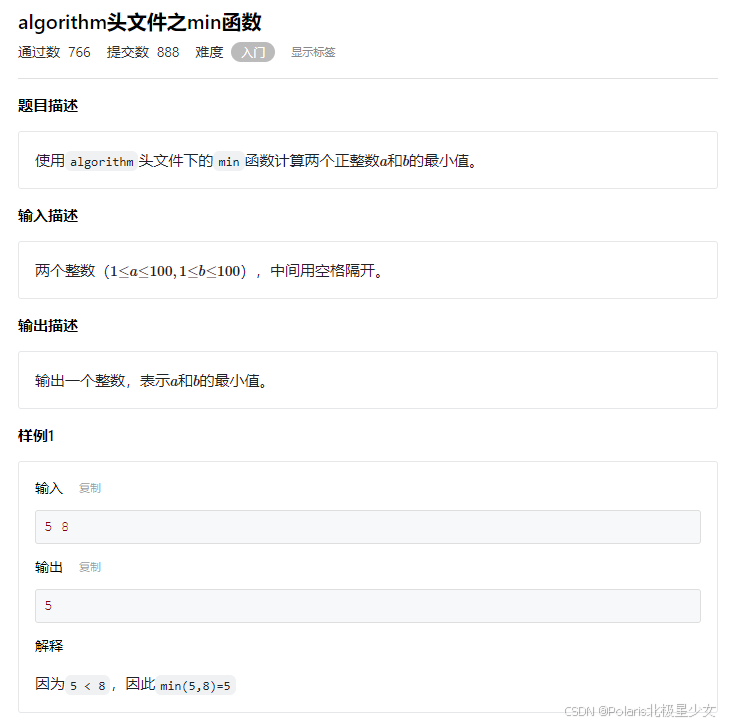
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int a, b;scanf("%d%d", &a, &b);printf("%d", min(a, b));return 0;
}
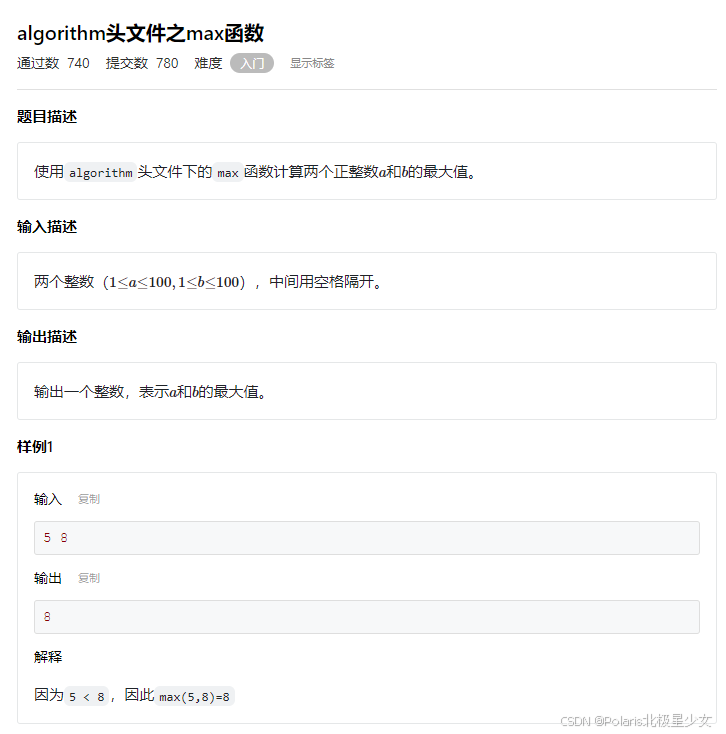
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int a, b;scanf("%d%d", &a, &b);printf("%d", max(a, b));return 0;
}
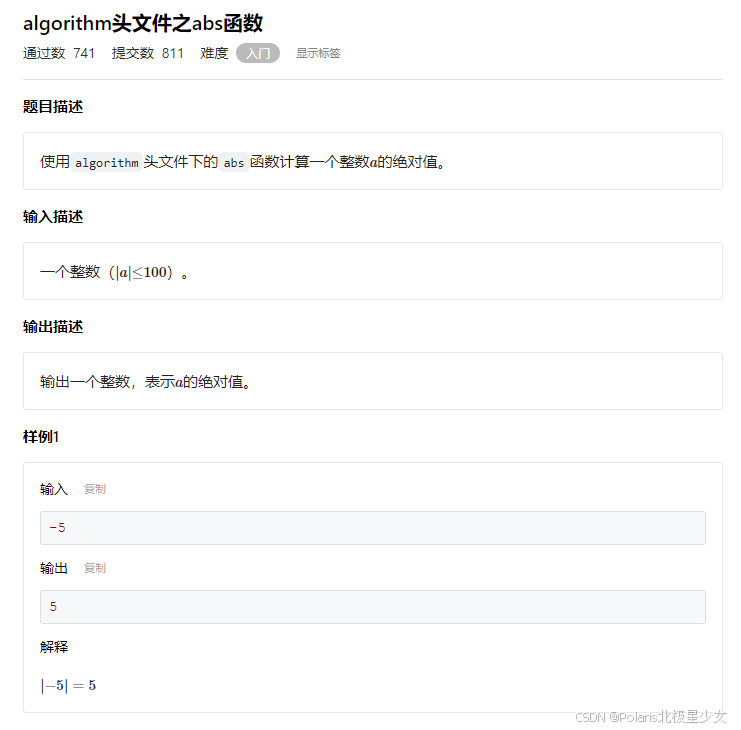
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int a;scanf("%d", &a);printf("%d", abs(a));return 0;
}
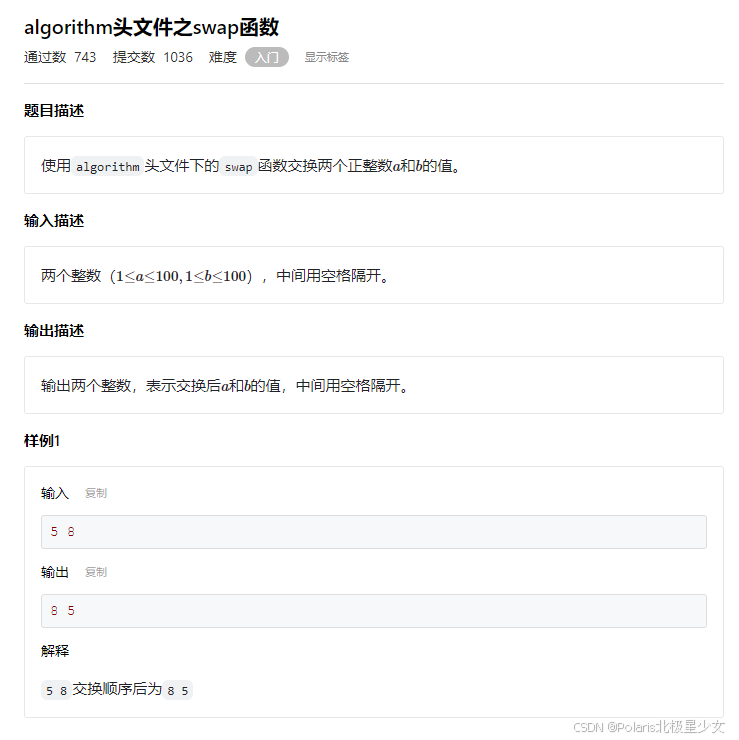
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int a, b;scanf("%d%d", &a, &b);swap(a,b);printf("%d %d", a, b);return 0;
}
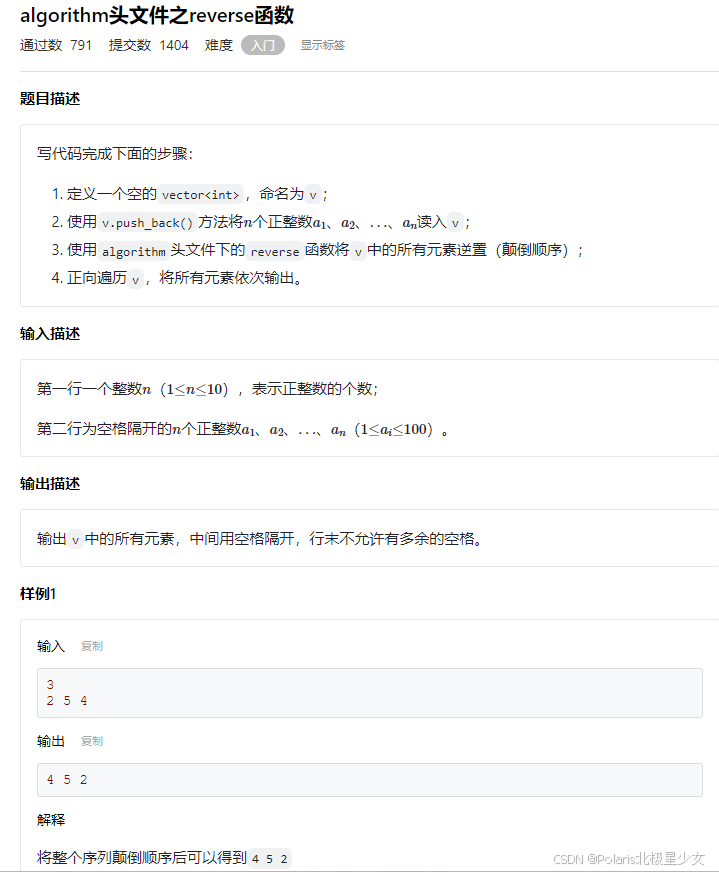
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int n,x;vector<int> v;scanf("%d",&n);for(int i=0;i<n;i++){scanf("%d",&x);v.push_back(x);}reverse(v.begin(), v.end());for (int i = 0; i < (int)v.size(); i++) {printf("%d", v[i]);if (i < (int)v.size() - 1) {printf(" ");}}return 0;
}
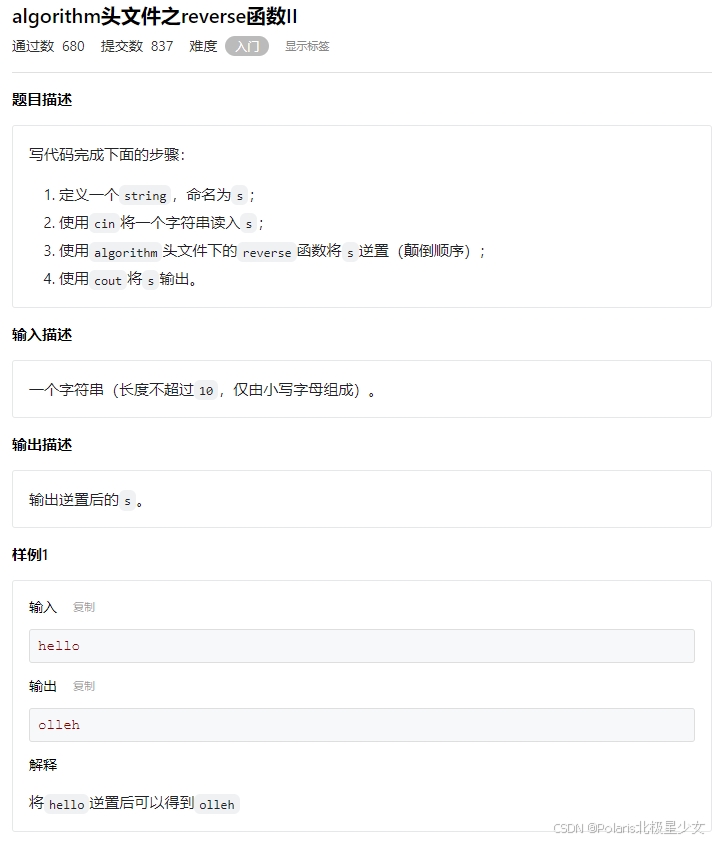
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;int main() {string str;cin >> str;reverse(str.begin(), str.end());cout << str;return 0;
}
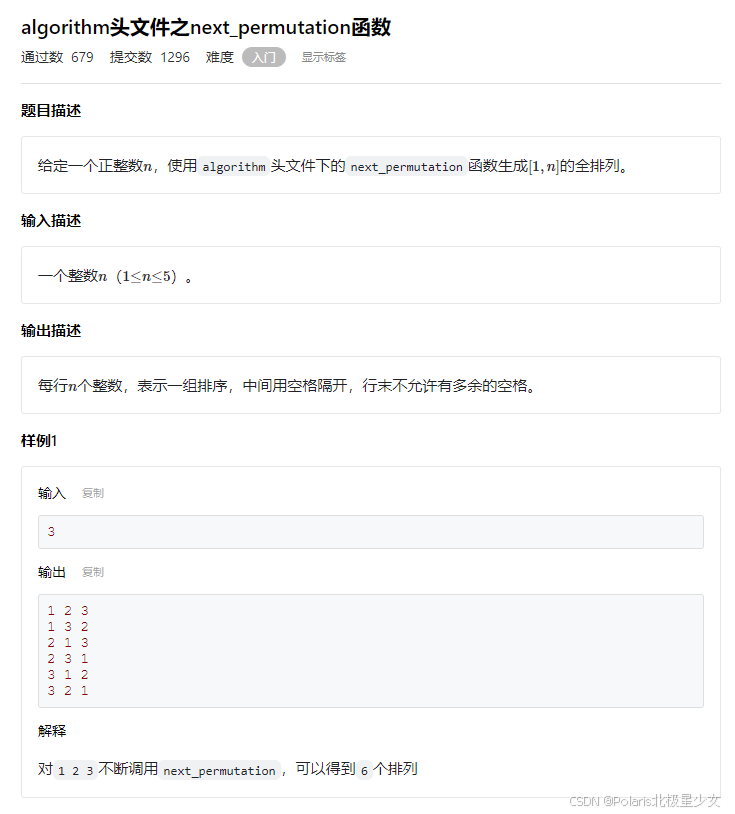
#include <cstdio>
#include <vector>
#include <algorithm>
using namespace std;int main() {int n, x;scanf("%d", &n);vector<int> v;for (int i = 1; i <= n; i++) {v.push_back(i);}do {for (int i = 0; i < n; i++) {printf("%d", v[i]);if (i < n - 1) {printf(" ");} else {printf("\n");}}} while (next_permutation(v.begin(), v.end()));return 0;
}
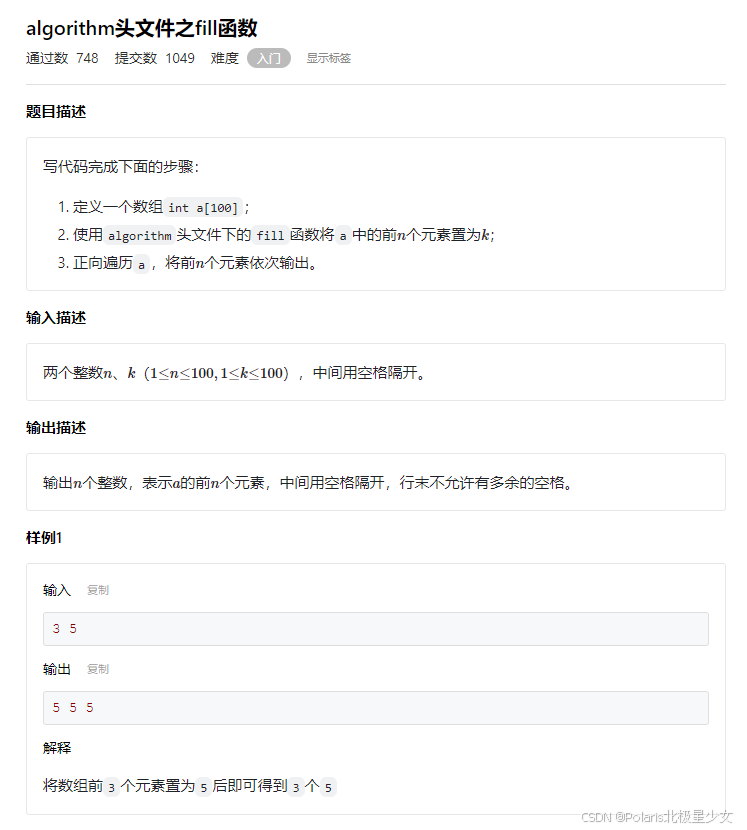
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int n, k, a[100];scanf("%d%d", &n, &k);fill(a, a + n, k);for (int i = 0; i < n; i++) {printf("%d", a[i]);if (i < n - 1) {printf(" ");}}return 0;
}
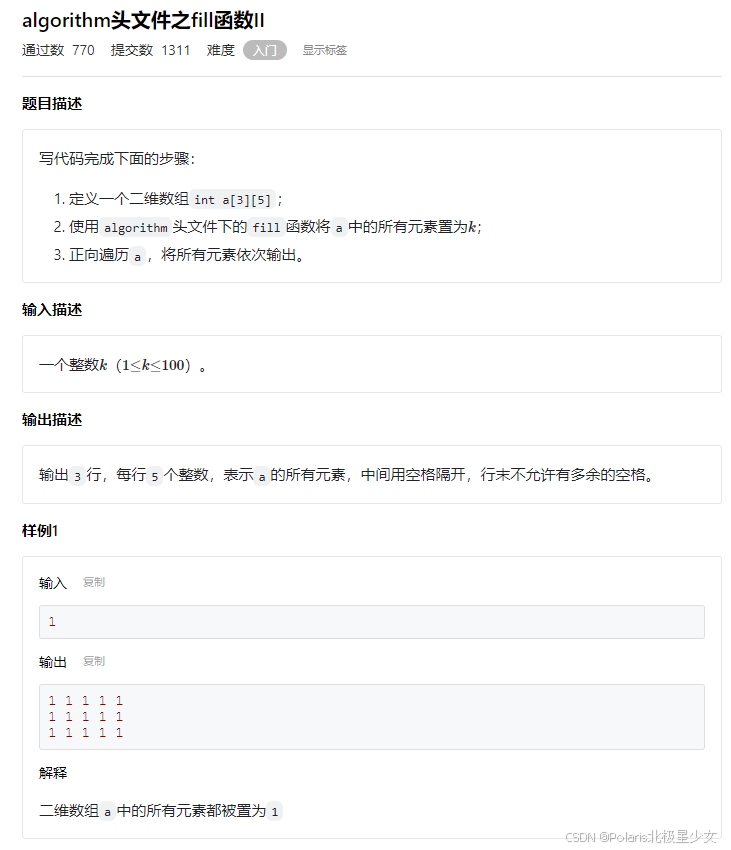
#include <cstdio>
#include <algorithm>
using namespace std;int main() {int n = 3, m = 5, k, a[3][5];scanf("%d", &k);fill(&a[0][0], &a[0][0] + n * m, k);for (int i = 0; i < n; i++) {for (int j = 0; j < m; j++) {printf("%d", a[i][j]);if (j < m - 1) {printf(" ");} else {printf("\n");}}}return 0;
}
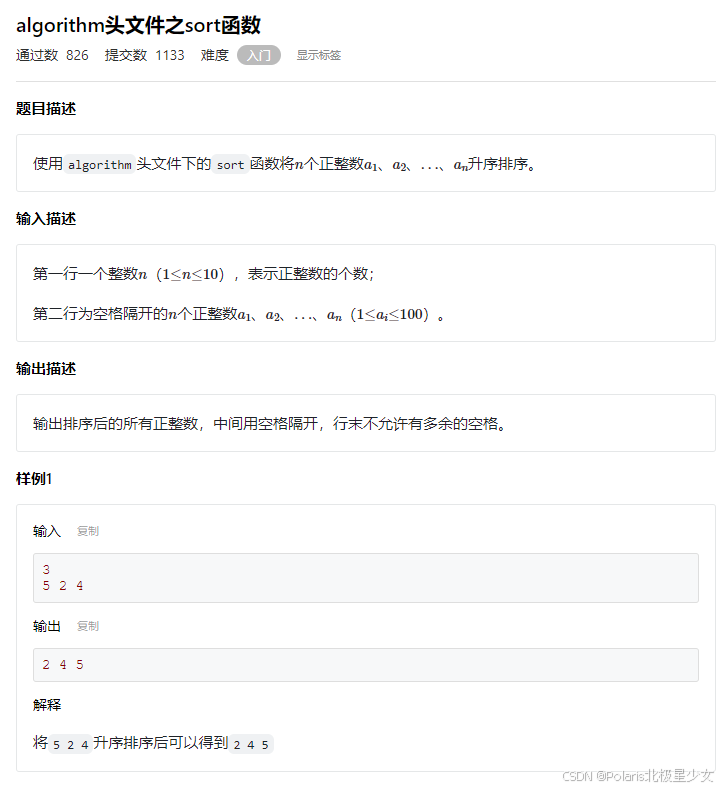
#include <cstdio>
#include <algorithm>
using namespace std;const int MAXN = 10;
int a[MAXN];int main() {int n;scanf("%d", &n);for (int i = 0; i < n; i++) {scanf("%d", &a[i]);}sort(a, a + n);for (int i = 0; i < n; i++) {printf("%d", a[i]);if (i < n - 1) {printf(" ");}}return 0;
}
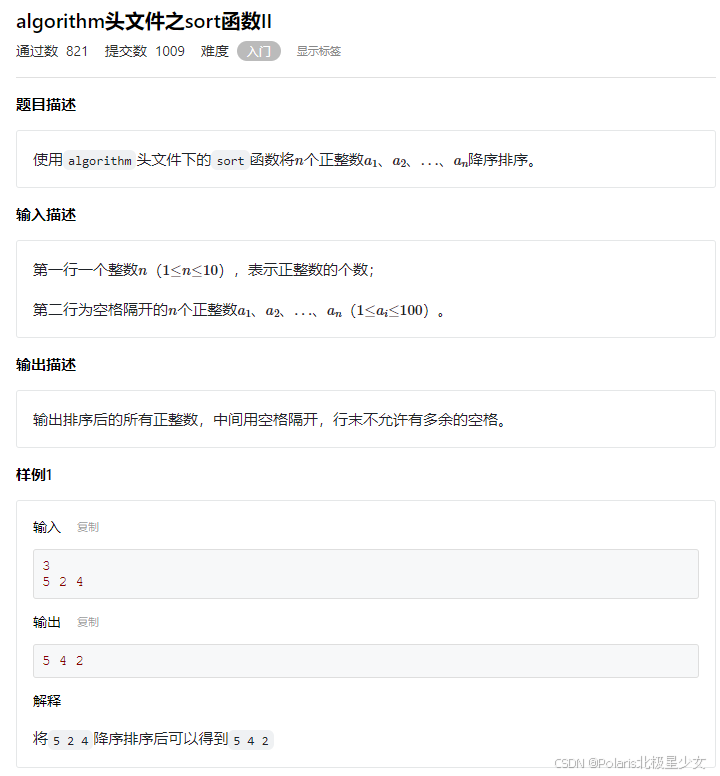
#include <cstdio>
#include <algorithm>
using namespace std;const int MAXN = 10;
int a[MAXN];bool cmp(int a, int b) {return a > b;
}int main() {int n;scanf("%d", &n);for (int i = 0; i < n; i++) {scanf("%d", &a[i]);}sort(a, a + n, cmp);for (int i = 0; i < n; i++) {printf("%d", a[i]);if (i < n - 1) {printf(" ");}}return 0;
}
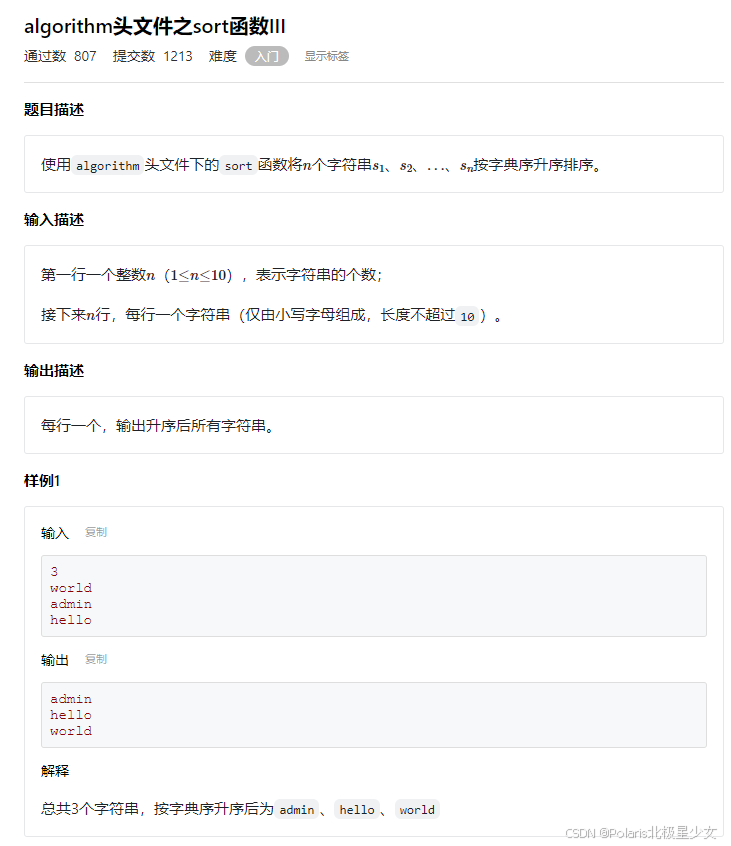
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;const int MAXN = 10;
string str[MAXN];int main() {int n;cin >> n;for (int i = 0; i < n; i++) {cin >> str[i];}sort(str, str + n);for (int i = 0; i < n; i++) {cout << str[i] << endl;}return 0;
}
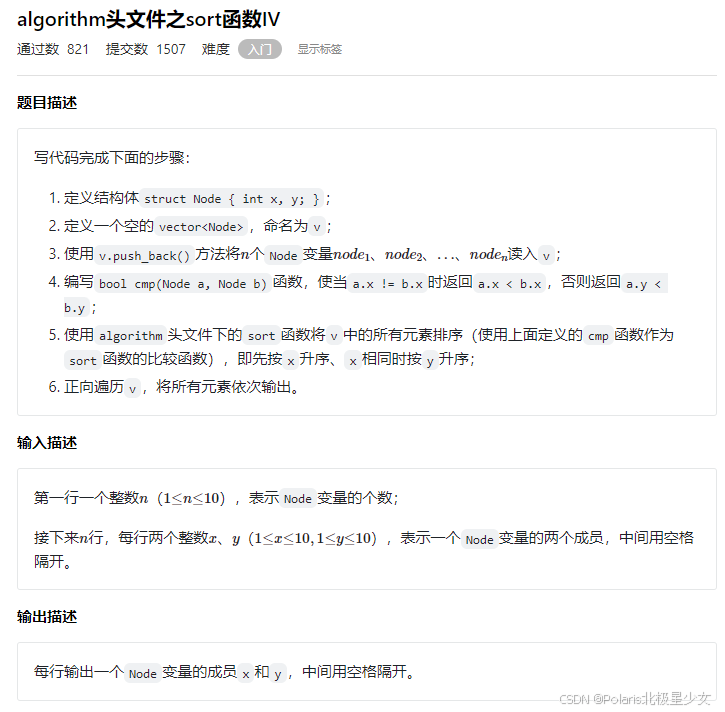
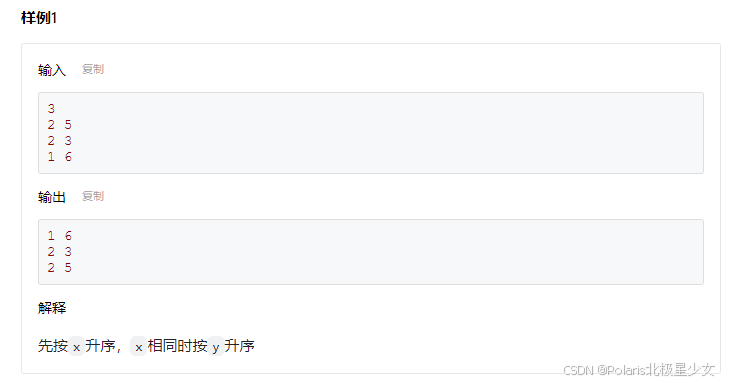
#include <cstdio>
#include <vector>
#include <algorithm>
using namespace std;struct Node {int x, y;Node(int _x, int _y) {x = _x;y = _y;}
};bool cmp(Node a, Node b) {if (a.x != b.x) {return a.x < b.x;} else {return a.y < b.y;}
}int main() {int n, x, y;scanf("%d", &n);vector<Node> v;for (int i = 0; i < n; i++) {scanf("%d%d", &x, &y);v.push_back(Node(x, y));}sort(v.begin(), v.end(), cmp);for (int i = 0; i < n; i++) {printf("%d %d\n", v[i].x, v[i].y);}return 0;
}
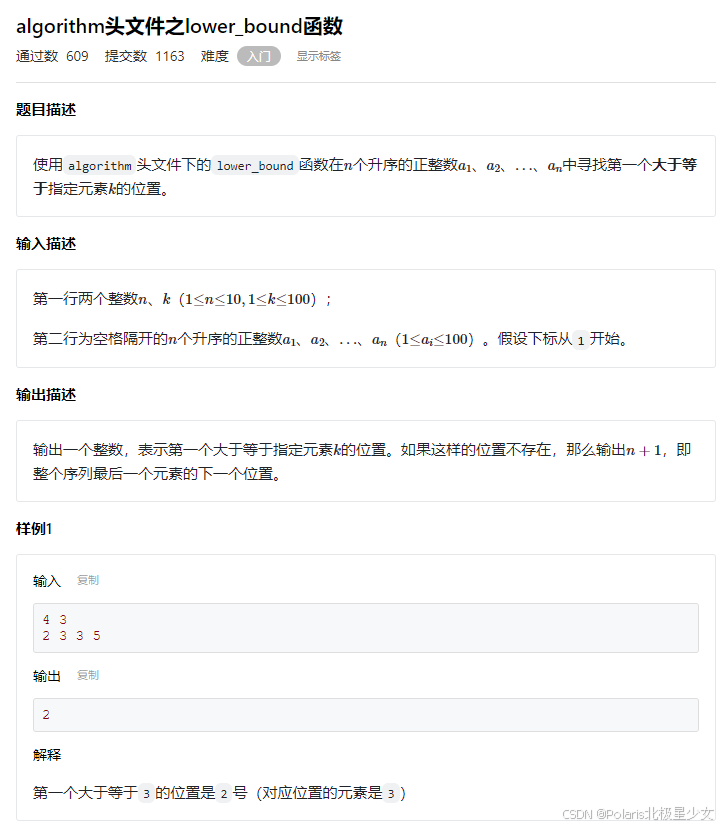
#include <cstdio>
#include <algorithm>
using namespace std;const int MAXN = 10;
int a[MAXN];int main() {int n, k;scanf("%d%d", &n, &k);for (int i = 0; i < n; i++) {scanf("%d", &a[i]);}int pos = lower_bound(a, a + n, k) - a;printf("%d", pos + 1);return 0;
}
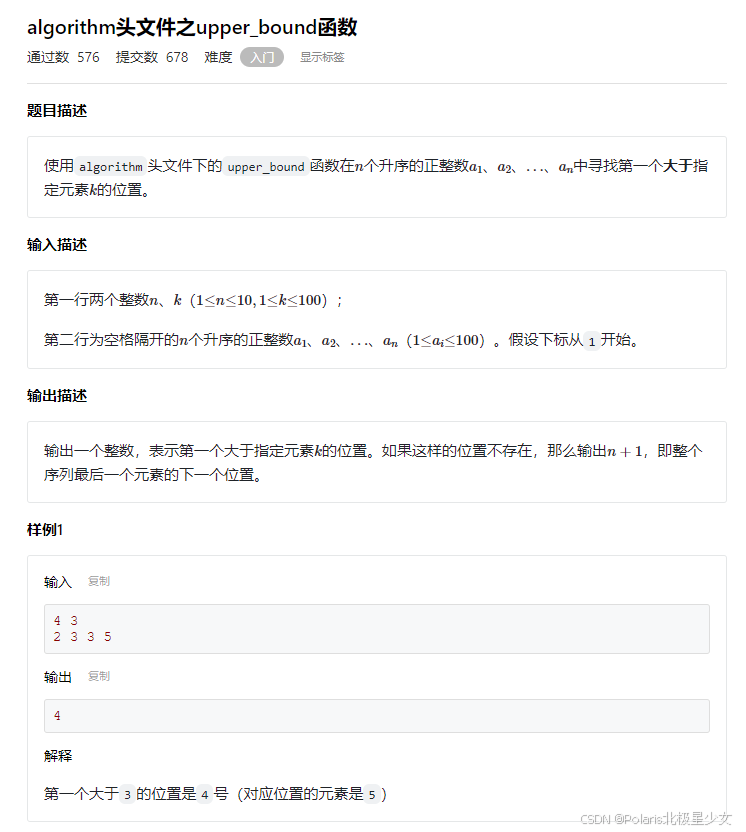
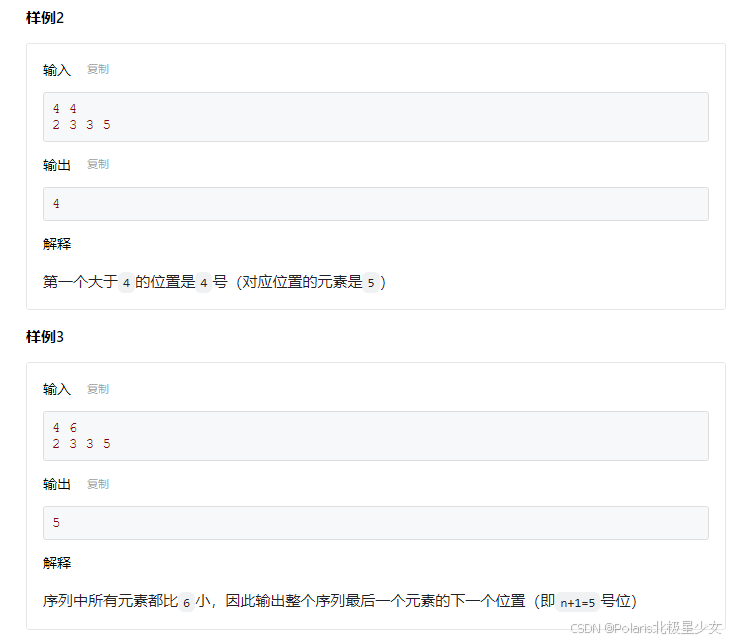
#include <cstdio>
#include <algorithm>
using namespace std;const int MAXN = 10;
int a[MAXN];int main() {int n, k;scanf("%d%d", &n, &k);for (int i = 0; i < n; i++) {scanf("%d", &a[i]);}int pos = upper_bound(a, a + n, k) - a;printf("%d", pos + 1);return 0;
}