LRUCache
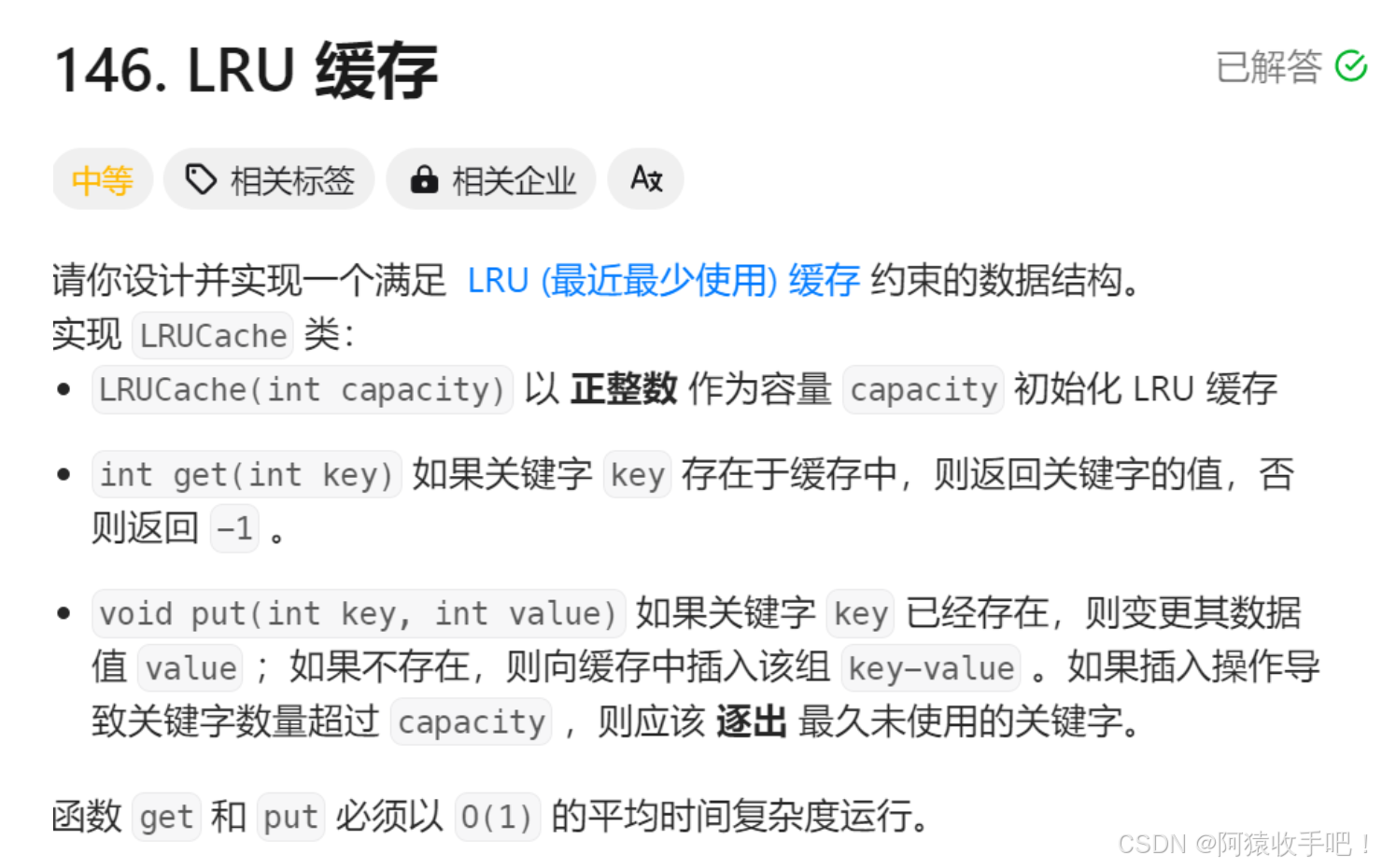
class LRUCache {using ListIt = list<pair<int, int>>::iterator;list<pair<int, int>> _LRUlist;int _capacity;unordered_map<int, ListIt> _hashmap;public:LRUCache(int capacity) : _capacity(capacity) {}int get(int key) {auto it = _hashmap.find(key);if (it != _hashmap.end()) {ListIt listit = it->second;_LRUlist.splice(_LRUlist.begin(), _LRUlist, listit);return listit->second;} elsereturn -1;}void put(int key, int value) {auto it = _hashmap.find(key);if (it == _hashmap.end()) {if (_hashmap.size() == _capacity) {pair<int, int> back = _LRUlist.back();_hashmap.erase(back.first);_LRUlist.pop_back();}_LRUlist.push_front(make_pair(key, value));_hashmap.insert(make_pair(key, _LRUlist.begin()));}else {ListIt listit = it->second;listit->second = value;_LRUlist.splice(_LRUlist.begin(), _LRUlist, listit);}}
};
字典树
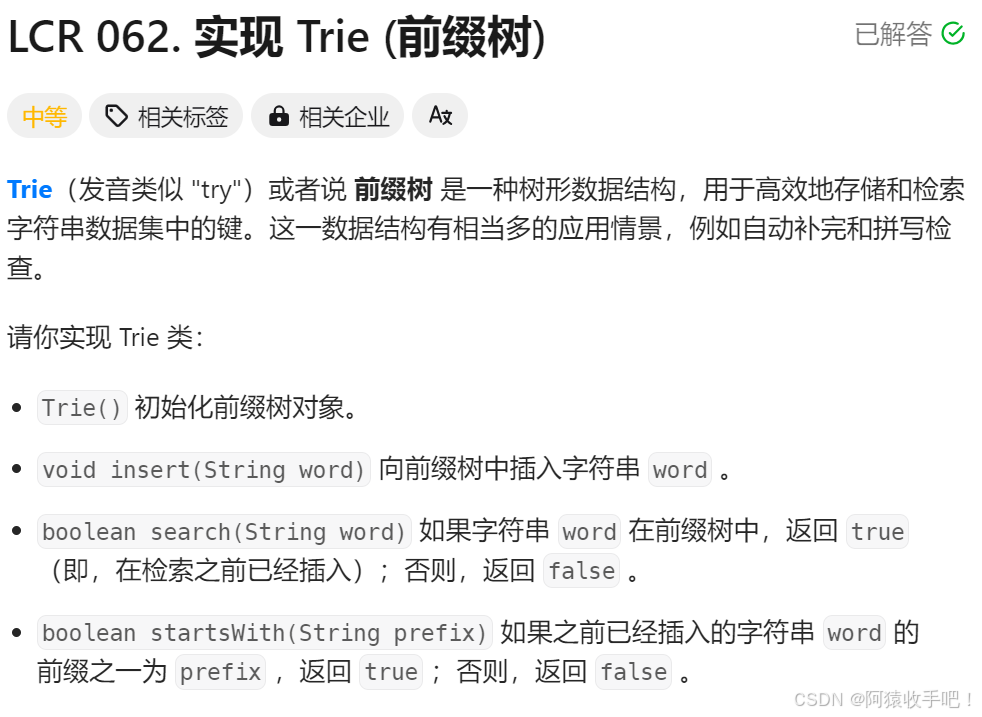
class Trie {
private:struct TreeNode {vector<TreeNode*> next;bool isEnd;TreeNode() {isEnd = false;next.resize(26, nullptr);}};void deleteTree(TreeNode* node) {if (node == nullptr)return;for (TreeNode* nextNode : node->next)deleteTree(nextNode);delete node;}public:TreeNode* root;Trie() { root = new TreeNode(); }~Trie() { deleteTree(root); }void insert(const std::string& word) {TreeNode* cur = root;for (char ch : word) {int index = ch - 'a';if (cur->next[index] == nullptr)cur->next[index] = new TreeNode();cur = cur->next[index];}cur->isEnd = true;}bool search(const std::string& word) {TreeNode* cur = root;for (char ch : word) {int index = ch - 'a';if (cur->next[index] == nullptr)return false;cur = cur->next[index];}return cur->isEnd;}bool startsWith(const std::string& prefix) {TreeNode* cur = root;for (char ch : prefix) {int index = ch - 'a';if (cur->next[index] == nullptr)return false;cur = cur->next[index];}return true;}bool query(const string& s) {TreeNode* cur = root;for (char c : s) {int u = c - 'a';cur = cur->next[u]; if (!cur->isEnd) return false;}return true; }
};
布隆过滤器
#pragma once#include <iostream>
#include <list>
#include <vector>
#include <algorithm>
#include <array>
#include <time.h>
#include <queue>
#include <stack>
#include <string>
#include <set>
#include <map>
#include <unordered_set>
#include <unordered_map>
#include <functional>
#include <assert.h>
using namespace std;
template <size_t N>
class bitmap
{
private:vector<char> _bits;public:bitmap(){_bits.resize(N / 8 + 1, 0);}void insert_setone(size_t x){size_t i = x / 8;size_t j = x % 8;_bits[i] |= (1 << j); }void erase_setzero(size_t x){size_t i = x / 8;size_t j = x % 8;_bits[i] &= ~(1 << j);}bool judge(size_t x){size_t i = x / 8;size_t j = x % 8;return _bits[i] & (1 << j);}
};void test_bitmap1()
{bitmap<100> bm;bm.insert_setone(10);bm.insert_setone(11);bm.insert_setone(15);cout << bm.judge(10) << endl;cout << bm.judge(15) << endl;bm.erase_setzero(10);cout << bm.judge(10) << endl;cout << bm.judge(15) << endl;bm.erase_setzero(10);bm.erase_setzero(15);cout << bm.judge(10) << endl;cout << bm.judge(15) << endl;
}void test_bitmap2()
{bitmap<0xFFFFFFFF> bm;
}
struct BKDR_Hash
{size_t operator()(const string &s){size_t value = 0;for (auto &ch : s){value = value * 31 + ch;}return value;}
};
struct AP_Hash
{size_t operator()(const string &s){size_t value = 0;for (long i = 0; i < s.size(); i++){size_t ch = s[i];if ((i & 1) == 0)value ^= ((value << 7) ^ ch ^ (value >> 3));elsevalue ^= (~((value << 11) ^ ch ^ (value >> 5)));}return value;}
};
struct DJB_Hash
{size_t operator()(const string &s){size_t value = 5381;for (auto ch : s){value += (value << 5) + ch;}return value;}
};template <size_t N, class K = string, class Hash1 = BKDR_Hash,class Hash2 = AP_Hash,class Hash3 = DJB_Hash>
class BloomFilter
{private:static const size_t num = 4; bitmap<num * N> _bm;
public:void insert_setone(const K &key){size_t len = num * N;size_t hash1 = Hash1()(key) % len;_bm.insert_setone(hash1);size_t hash2 = Hash2()(key) % len;_bm.insert_setone(hash2);size_t hash3 = Hash3()(key) % len;_bm.insert_setone(hash3);}bool judge(const K &key){size_t len = N * num;size_t hash1 = Hash1()(key) % len;if (!_bm.judge(hash1)){return false;}size_t hash2 = Hash2()(key) % len;if (!_bm.judge(hash2)){return false;}size_t hash3 = Hash3()(key) % len;if (!_bm.judge(hash3)){return false;}return true;}
};
void test_bloomfilter1()
{BloomFilter<100> bf;bf.insert_setone("sort");bf.insert_setone("bloom");bf.insert_setone("hello world hello bit");bf.insert_setone("test");bf.insert_setone("etst");bf.insert_setone("estt");cout << bf.judge("sort") << endl;cout << bf.judge("bloom") << endl;cout << bf.judge("hello world hello bit") << endl;cout << bf.judge("etst") << endl;cout << bf.judge("test") << endl;cout << bf.judge("estt") << endl;cout << bf.judge("ssort") << endl;cout << bf.judge("tors") << endl;cout << bf.judge("ttes") << endl;
}
void test_bloomfilter2()
{srand(time(0));const size_t N = 10000;BloomFilter<N> bf; vector<string> v1;string url = "https://www.gitee.com/Ape-LHR/apes-warehouse/547993.html";for (size_t i = 0; i < N; ++i){v1.push_back(url + to_string(i));}for (auto &str : v1){bf.insert_setone(str);}vector<string> v2;for (size_t i = N; i < 2 * N; ++i){v2.push_back(url + to_string(i));}size_t count1 = 0;for (auto &str : v2){if (bf.judge(str))++count1;}double rate1 = (double)count1 / (double)N;cout << "相似字符串误判率 :" << rate1 * 100 << "%" << endl;vector<string> v3;string url2 = "https://www.csdn.net/?spm=1001.2014.3001.4476";for (size_t i = 0; i < N; ++i){v3.push_back(url2 + to_string(i + rand()));}size_t count2 = 0;for (auto &str : v3){if (bf.judge(str))++count2;}double rate2 = (double)count2 / (double)N;cout << "不相似字符串误判率:" << rate2 * 100 << "%" << endl;
}