react如何获取真实DOM:useRef, React.CreateRef()
access DOM nodes directly within React:ref.current
Let’s say you want to change the value of an <input> element, but without using props or re-rendering the whole component. They’re an excellent way to update part of a component without the need to re-render the entire thing.
1. useRef
const {useRef} = React
const App=()=>{
const buttonRef=useRef();
return(
<button
onClick={()=>{console.log(buttonRef.current);}}
ref={buttonRef} >
Special Button
</button>
);
}
2. React.createRef()
create a ref by calling React.createRef() and attaching a React element to it using the ref attribute on the element.
class Example extends React.Component {
constructor(props) {
super(props)
this.exampleRef = React.createRef() // Create the ref
}
render() {
return (
<div>
//这里是uncontrolled form
<input type="text" ref={this.exampleRef} /> // Call the ref with the `ref` attribute
</div>
)
}}
3. Passing a callback function to ref
create a ref by passing a callback function to the ref attribute of a component.
<input type="text" ref={element=>this.textInput=element}/>
The callback is used to store a reference to the DOM node in an instance property. When we want to make use of this reference, we access it using: this.textInput.value
When you make use of callback like we did above, React will call the ref callback with the DOM node when the component mounts, when the component un-mounts, it will call it with null.
class App extends React.Component {
state = { value: '' }
handleSubmit = e => {
e.preventDefault();
this.setState({ value: this.textInput.value})
};
render() {
return (
<div>
<h3>Value: {this.state.value}</h3>
<form onSubmit={this.handleSubmit}>
<input type="text" ref={element => this.textInput = element} />
<button>Submit</button>
</form>
</div>
); }}
4. pass ref from a parent component to a child component using callbacks.
child:
const Input = props =>{
return(
//This component is expecting inputRef props from its parent component which is then used to create a ref to the DOM node.
<inputtype="text" ref={ props.inputRef }/>
);
};
parent:
In the App component, we want to obtain the text that is entered in the input field (which is in the child component) so we can render it. The ref is created using a callback like we did in the first example of this section. The key lies in how we access the DOM of the input element in the Input component from the App component. If you look closely, we access it using this.inputElement. So, when updating the state of value in the App component, we get the text that was entered in the input field using this.inputElement.value.
class App extends React.Component{
state={
value:""
};
handleSubmit=event=>{
this.setState({ value:this.inputElement.value });
};
render() {
return(
<div>
<h3>Value: {this.state.value}</h3>
<Input inputRef={el=>(this.inputElement=el)}/>
<button onClick={this.handleSubmit}>Submit</button>
</div>
);}}
5. Forwarding a ref from one component to another
Ref forwarding is the technique of passing a ref from a component to a child component by making use of the React.forwardRef() method.
child:
const Input=React.forwardRef((props,ref)=>(
<inputtype="text"ref={ref}/>
));
parent:
class App extends React.Component{
constructor(props) {
super(props)
this.inputRef=React.createRef();
this.state={value:''}
}
handleSubmit=e=>{
e.preventDefault();
this.setState({value:this.inputRef.current.value})
};
render() {
return(
<div>
<h3>Value: {this.state.value}</h3>
<form onSubmit={this.handleSubmit}>
<Input ref={this.inputRef}/>
<button>Submit</button>
</form>
</div>
);}}
uncontrolled form example: Using ref for form validation
class App extends React.Component {
constructor(props) {
super(props);
this.username = React.createRef();
this.password = React.createRef();
this.state = {
errors: []
};
}
handleSubmit = (event) => {
event.preventDefault();
const username = this.username.current.value;
const password = this.password.current.value;
const errors = this.handleValidation(username, password);
if (errors.length > 0) {
this.setState({ errors });
return;
}
// Submit data
};
handleValidation = (username,password) => {
const errors = [];
if (username.length === 0) { errors.push("Username cannot be empty"); }
if (password.length < 6) { errors.push("Password should be at least 6 characters long");}
return errors;
};
render() {
const { errors } = this.state;
return (
<form onSubmit={this.handleSubmit}>
{errors.map(error => <p key={error}>{error}</p>)}
<input type="text" ref={this.username} />
<input type="text" ref={this.password} />
<button>Submit</button>
</form>
);}}
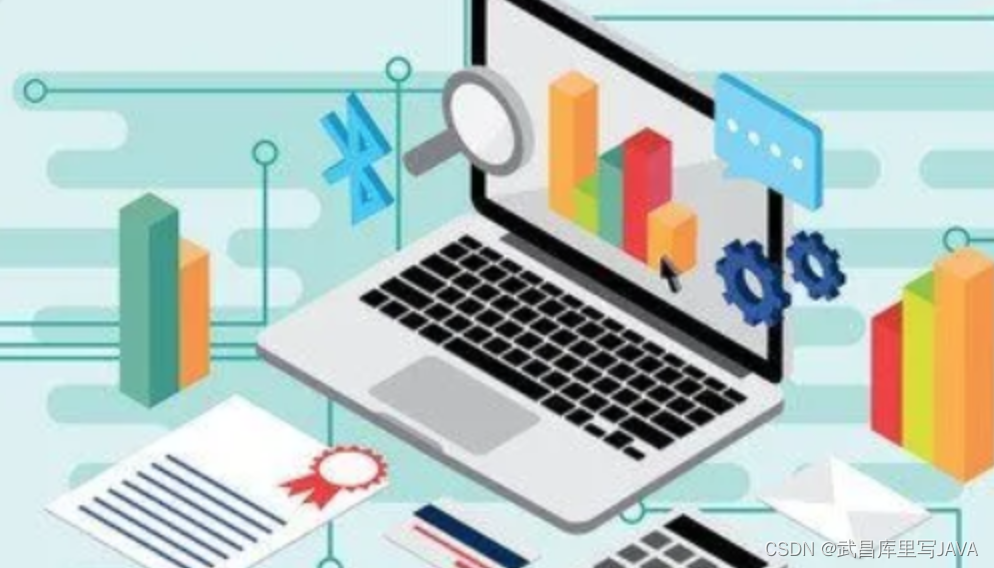
喜欢的朋友记得点赞、收藏、关注哦!!!