运行出的游戏界面如下: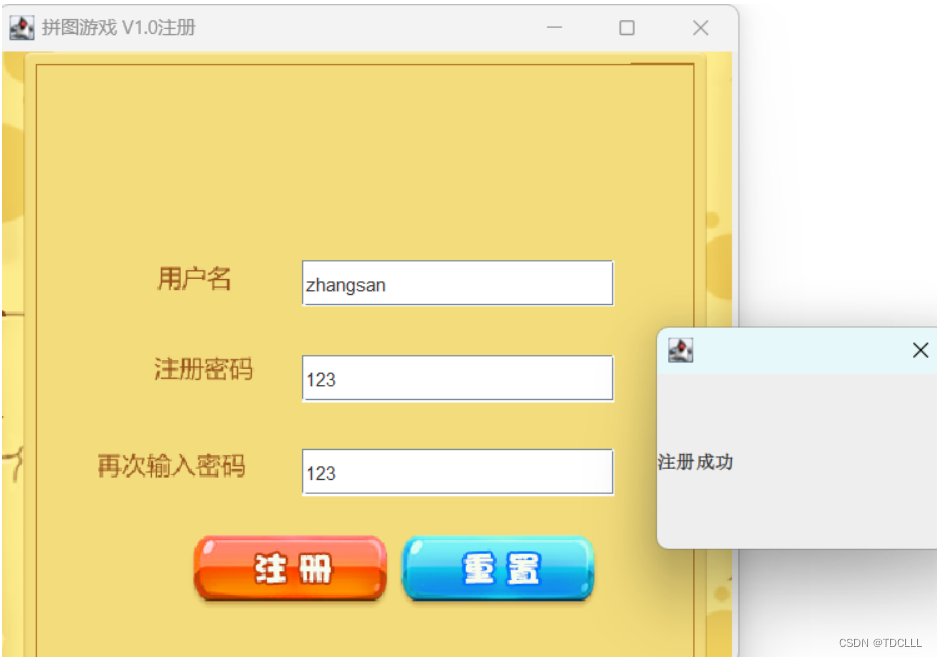
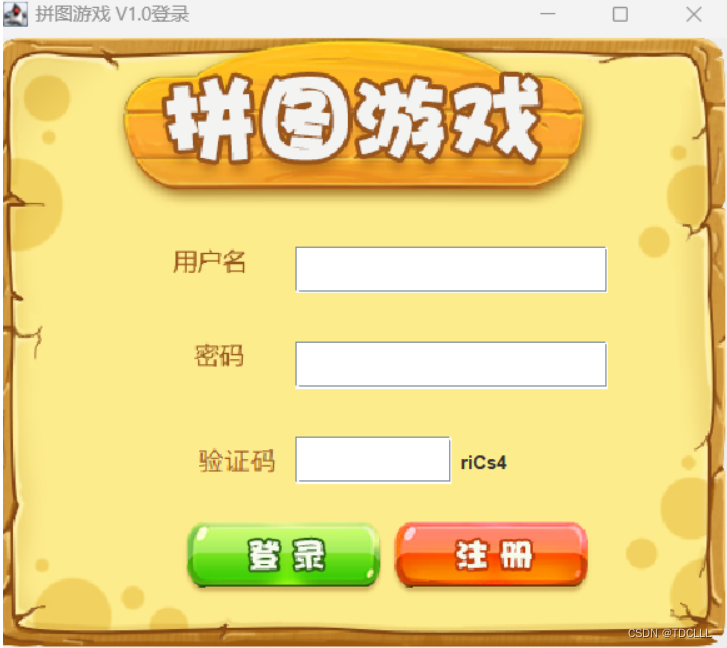
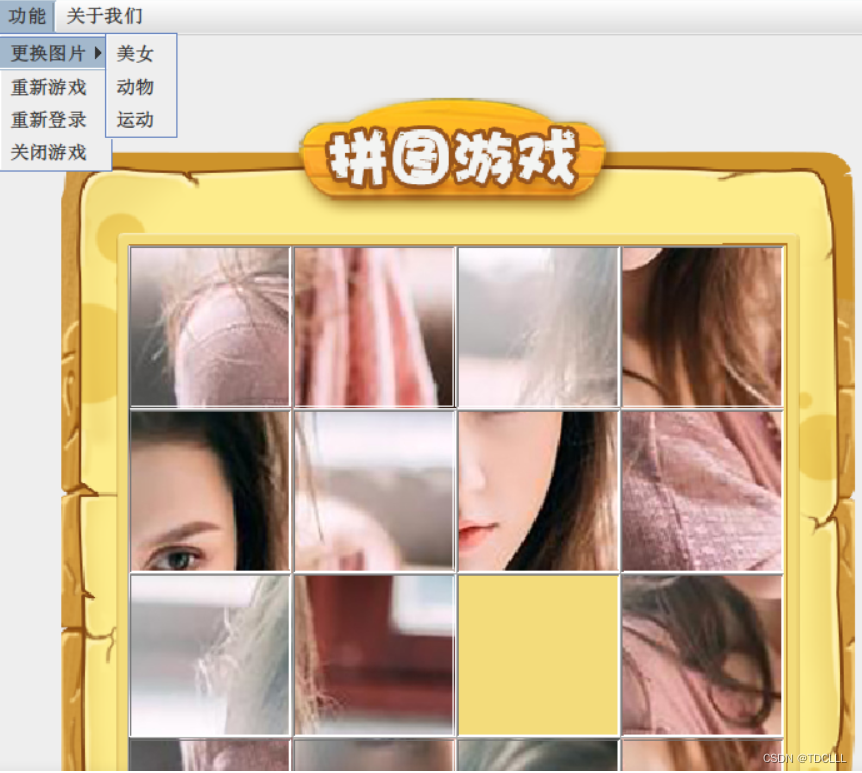
按住A不松开,显示完整图片;松开A显示随机打乱的图片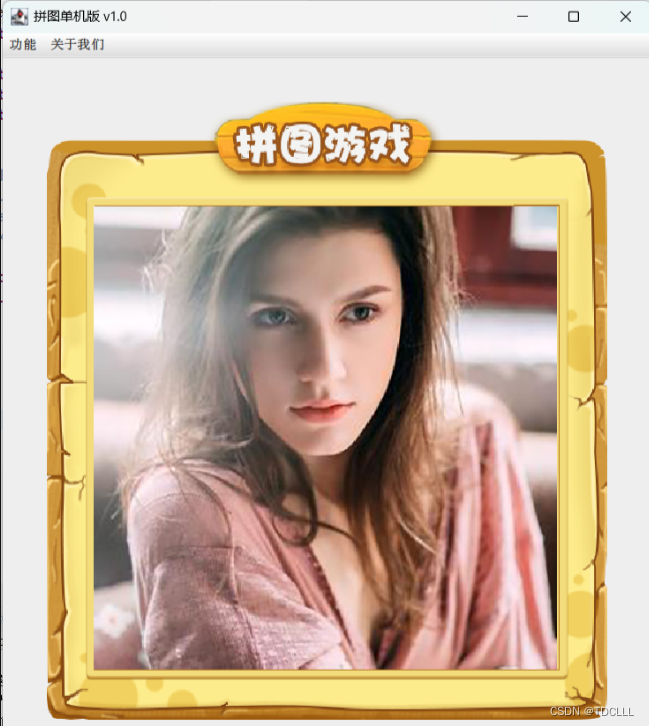
User类
package domain;/*** @ClassName: User* @Author: Kox* @Data: 2023/2/2* @Sketch:*/
public class User {private String username;private String password;public User() {}public User(String username, String password) {this.username = username;this.password = password;}/*** 获取* @return username*/public String getUsername() {return username;}/*** 设置* @param username*/public void setUsername(String username) {this.username = username;}/*** 获取* @return password*/public String getPassword() {return password;}/*** 设置* @param password*/public void setPassword(String password) {this.password = password;}}
CodeUtil类
package util;import java.util.ArrayList;
import java.util.Random;public class CodeUtil {public static String getCode(){//1.创建一个集合ArrayList<Character> list = new ArrayList<>();//52 索引的范围:0 ~ 51//2.添加字母 a - z A - Zfor (int i = 0; i < 26; i++) {list.add((char)('a' + i));//a - zlist.add((char)('A' + i));//A - Z}//3.打印集合//System.out.println(list);//4.生成4个随机字母String result = "";Random r = new Random();for (int i = 0; i < 4; i++) {//获取随机索引int randomIndex = r.nextInt(list.size());char c = list.get(randomIndex);result = result + c;}//System.out.println(result);//长度为4的随机字符串//5.在后面拼接数字 0~9int number = r.nextInt(10);//6.把随机数字拼接到result的后面result = result + number;//System.out.println(result);//ABCD5//7.把字符串变成字符数组char[] chars = result.toCharArray();//[A,B,C,D,5]//8.在字符数组中生成一个随机索引int index = r.nextInt(chars.length);//9.拿着4索引上的数字,跟随机索引上的数字进行交换char temp = chars[4];chars[4] = chars[index];chars[index] = temp;//10.把字符数组再变回字符串String code = new String(chars);//System.out.println(code);return code;}
}
游戏设置
package ui;import javax.swing.*;
import javax.swing.border.BevelBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;/*** @ClassName: GameJFrame* @Author: Kox* @Data: 2023/1/30* @Sketch:*/
public class GameJFrame extends JFrame implements KeyListener, ActionListener {// 管理数据int[][] data = new int[4][4];// 记录空白方块在二维数组的位置int x = 0;int y = 0;// 展示当前图片的路径String path = "拼图小游戏_image\\image\\girl\\girl7\\";// 存储正确的数据int[][] win = {{1, 2, 3, 4},{5, 6, 7, 8},{9, 10, 11, 12},{13, 14, 15, 0}};// 定义变量用来统计步数int step = 0;// 选项下面的条目对象JMenuItem replayItem = new JMenuItem("重新游戏");JMenuItem reLoginItem = new JMenuItem("重新登录");JMenuItem closeItem = new JMenuItem("关闭游戏");JMenuItem accountItem = new JMenuItem("公众号");JMenuItem beautiful = new JMenuItem("美女");JMenuItem animal = new JMenuItem("动物");JMenuItem exercise = new JMenuItem("运动");// 游戏界面public GameJFrame() {// 初始化界面initJFrame();// 初始化菜单initJMenuBar();// 初始化数据initDate();// 初始化图片initImage();// 显示this.setVisible(true);}// 数据private void initDate() {int[] tempArr = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15};Random r = new Random();for (int i = 0; i < tempArr.length; i++) {int index = r.nextInt(tempArr.length);int temp = tempArr[i];tempArr[i] = tempArr[index];tempArr[index] = temp;}for (int i = 0; i < tempArr.length; i++) {if (tempArr[i] == 0) {x = i / 4;y = i % 4;}data[i / 4][i % 4] = tempArr[i];}}// 图片private void initImage() {// 清空原本已经出现的所有图片this.getContentPane().removeAll();if (victory()) {JLabel winJLabel = new JLabel(new ImageIcon("拼图小游戏_image\\image\\win.png"));winJLabel.setBounds(203, 283, 197, 73);this.getContentPane().add(winJLabel);}JLabel stepCount = new JLabel("步数:" + step);stepCount.setBounds(50, 30, 100, 20);this.getContentPane().add(stepCount);for (int i = 0; i < 4; i++) {for (int j = 0; j < 4; j++) {int num = data[i][j];// 创建一个图片ImageIcon对象ImageIcon icon = new ImageIcon(path + num + ".jpg");// 创建一个JLabel的对象JLabel jLabel1 = new JLabel(icon);// 指定图片位置jLabel1.setBounds(105 * j + 83, 105 * i + 134, 105, 105);// 给图片添加边框jLabel1.setBorder(new BevelBorder(BevelBorder.LOWERED));// 管理容器添加到界面中this.getContentPane().add(jLabel1);}}// 添加背景图片JLabel background = new JLabel(new ImageIcon("拼图小游戏_image\\image\\background.png"));background.setBounds(40, 40, 508, 560);this.getContentPane().add(background);// 刷新一下界面this.getContentPane().repaint();}// 菜单private void initJMenuBar() {// 菜单对象JMenuBar jMenuBar = new JMenuBar();// 选项-功能JMenu functionJMenu = new JMenu("功能");// 选项-关于我们JMenu aboutJMenu = new JMenu("关于我们");// 选项-换图JMenu changePicture = new JMenu("更换图片");// 选项下面的条目添加到选项中functionJMenu.add(changePicture);functionJMenu.add(replayItem);functionJMenu.add(reLoginItem);functionJMenu.add(closeItem);changePicture.add(beautiful);changePicture.add(animal);changePicture.add(exercise);aboutJMenu.add(accountItem);// 给条目绑定事件replayItem.addActionListener(this);reLoginItem.addActionListener(this);closeItem.addActionListener(this);accountItem.addActionListener(this);beautiful.addActionListener(this);animal.addActionListener(this);exercise.addActionListener(this);// 将菜单里面的两个选项添加到菜单当中jMenuBar.add(functionJMenu);jMenuBar.add(aboutJMenu);// 给整个界面设置菜单this.setJMenuBar(jMenuBar);}// 界面private void initJFrame() {// 设置界面的宽高this.setSize(603, 680);// 设置界面的标题this.setTitle("拼图单机版 v1.0");// 设置界面置顶this.setAlwaysOnTop(true);// 设置界面居中this.setLocationRelativeTo(null);// 设置关闭模式this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);// 取消默认的居中放置this.setLayout(null);// 添加键盘监听事件this.addKeyListener(this);}@Overridepublic void keyTyped(KeyEvent e) {}// 按下@Overridepublic void keyPressed(KeyEvent e) {int code = e.getKeyCode();if (code == 65) {// 删除界面中的素有图片this.getContentPane().removeAll();// 加载第一张完整的图片JLabel all = new JLabel(new ImageIcon(path + "all.jpg"));all.setBounds(83, 134, 420, 420);this.getContentPane().add(all);// 添加背景图片JLabel background = new JLabel(new ImageIcon("拼图小游戏_image\\image\\background.png"));background.setBounds(40, 40, 508, 560);this.getContentPane().add(background);// 刷新界面this.getContentPane().repaint();}}// 松下@Overridepublic void keyReleased(KeyEvent e) {// 判断游戏是否胜利if (victory()) {return;}int code = e.getKeyCode();if (code == 37) {System.out.println("向左移动");if (y == 3) {return;}data[x][y] = data[x][y + 1];data[x][y + 1] = 0;y++;// 计算器step++;initImage();} else if(code == 38) {System.out.println("向上移动");if (x == 3) {return;}data[x][y] = data[x + 1][y];data[x + 1][y] = 0;x++;// 计算器step++;initImage();} else if(code == 39) {System.out.println("向右移动");if (y == 0) {return;}data[x][y] = data[x][y - 1];data[x][y - 1] = 0;y--;// 计算器step++;initImage();} else if(code == 40) {System.out.println("向下移动");if (x == 0) {return;}data[x][y] = data[x - 1][y];data[x - 1][y] = 0;x--;// 计算器step++;initImage();} else if (code == 65) {initImage();} else if (code == 87) {initImage();data = new int[][] {{1, 2, 3, 4},{5, 6, 7, 8},{9, 10, 11, 12},{13, 14, 15, 0}};initImage();}}// 胜利public boolean victory() {for (int i = 0; i < data.length; i++) {for (int j = 0; j < data[i].length; j++) {if (data[i][j] != win[i][j]) {return false;}}}return true;}@Overridepublic void actionPerformed(ActionEvent e) {// 获取当前被点击的条目对象Object obj = e.getSource();Random r = new Random();// 判断if (obj == beautiful) {System.out.println("换美女");int index = r.nextInt(11) + 1;path = "拼图小游戏_image\\image\\girl\\girl" + index +"\\";step = 0;initDate();initImage();} else if (obj == animal) {System.out.println("换动物");int index = r.nextInt(8) + 1;path = "拼图小游戏_image\\image\\animal\\animal" + index +"\\";step = 0;initDate();initImage();} else if (obj == exercise) {System.out.println("换运动");int index = r.nextInt(10) + 1;path = "拼图小游戏_image\\image\\sport\\sport" + index +"\\";step = 0;initDate();initImage();}if (obj == replayItem) {System.out.println("重新游戏");step = 0;initDate();initImage();} else if(obj == reLoginItem) {System.out.println("重新登录");this.setVisible(false);new LoginJFrame();} else if(obj == closeItem) {System.out.println("关闭游戏");System.exit(0);} else if(obj == accountItem) {System.out.println("公众号");JDialog jDialog = new JDialog();JLabel jLabel = new JLabel(new ImageIcon("拼图小游戏_image\\image\\about.png"));jLabel.setBounds(0, 0, 150, 150);jDialog.getContentPane().add(jLabel);jDialog.setSize(344, 344);jDialog.setAlwaysOnTop(true);jDialog.setLocationRelativeTo(null);jDialog.setModal(true);jDialog.setVisible(true);}}
}
登陆代码
package ui;import domain.User;
import util.CodeUtil;import javax.swing.*;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.util.ArrayList;public class LoginJFrame extends JFrame implements MouseListener {static ArrayList<User> allUsers = new ArrayList<>();static {allUsers.add(new User("zhangsan","123"));allUsers.add(new User("lisi","1234"));}JButton login = new JButton();JButton register = new JButton();JTextField username = new JTextField();//JTextField password = new JTextField();JPasswordField password = new JPasswordField();JTextField code = new JTextField();//正确的验证码JLabel rightCode = new JLabel();public LoginJFrame() {//初始化界面initJFrame();//在这个界面中添加内容initView();//让当前界面显示出来this.setVisible(true);}public void initView() {//1. 添加用户名文字JLabel usernameText = new JLabel(new ImageIcon("拼图小游戏_image\\image\\login\\用户名.png"));usernameText.setBounds(116, 135, 47, 17);this.getContentPane().add(usernameText);//2.添加用户名输入框username.setBounds(195, 134, 200, 30);this.getContentPane().add(username);//3.添加密码文字JLabel passwordText = new JLabel(new ImageIcon("拼图小游戏_image\\image\\login\\密码.png"));passwordText.setBounds(130, 195, 32, 16);this.getContentPane().add(passwordText);//4.密码输入框password.setBounds(195, 195, 200, 30);this.getContentPane().add(password);//验证码提示JLabel codeText = new JLabel(new ImageIcon("拼图小游戏_image\\image\\login\\验证码.png"));codeText.setBounds(133, 256, 50, 30);this.getContentPane().add(codeText);//验证码的输入框code.setBounds(195, 256, 100, 30);this.getContentPane().add(code);String codeStr = CodeUtil.getCode();//设置内容rightCode.setText(codeStr);//绑定鼠标事件rightCode.addMouseListener(this);//位置和宽高rightCode.setBounds(300, 256, 50, 30);//添加到界面this.getContentPane().add(rightCode);//5.添加登录按钮login.setBounds(123, 310, 128, 47);login.setIcon(new ImageIcon("拼图小游戏_image\\image\\login\\登录按钮.png"));//去除按钮的边框login.setBorderPainted(false);//去除按钮的背景login.setContentAreaFilled(false);//给登录按钮绑定鼠标事件login.addMouseListener(this);this.getContentPane().add(login);//6.添加注册按钮register.setBounds(256, 310, 128, 47);register.setIcon(new ImageIcon("拼图小游戏_image\\image\\login\\注册按钮.png"));//去除按钮的边框register.setBorderPainted(false);//去除按钮的背景register.setContentAreaFilled(false);//给注册按钮绑定鼠标事件register.addMouseListener(this);this.getContentPane().add(register);//7.添加背景图片JLabel background = new JLabel(new ImageIcon("拼图小游戏_image\\image\\login\\background.png"));background.setBounds(0, 0, 470, 390);this.getContentPane().add(background);}public void initJFrame() {this.setSize(488, 430);//设置宽高this.setTitle("拼图游戏 V1.0登录");//设置标题this.setDefaultCloseOperation(3);//设置关闭模式this.setLocationRelativeTo(null);//居中this.setAlwaysOnTop(true);//置顶this.setLayout(null);//取消内部默认布局}//点击@Overridepublic void mouseClicked(MouseEvent e) {if (e.getSource() == login) {System.out.println("点击了登录按钮");//获取两个文本输入框中的内容String usernameInput = username.getText();String passwordInput = password.getText();//获取用户输入的验证码String codeInput = code.getText();//创建一个User对象User userInfo = new User(usernameInput, passwordInput);System.out.println("用户输入的用户名为" + usernameInput);System.out.println("用户输入的密码为" + passwordInput);if (codeInput.length() == 0) {showJDialog("验证码不能为空");} else if (usernameInput.length() == 0 || passwordInput.length() == 0) {//校验用户名和密码是否为空System.out.println("用户名或者密码为空");//调用showJDialog方法并展示弹框showJDialog("用户名或者密码为空");} else if (!codeInput.equalsIgnoreCase(rightCode.getText())) {showJDialog("验证码输入错误");} else if (contains(userInfo)) {System.out.println("用户名和密码正确可以开始玩游戏了");//关闭当前登录界面this.setVisible(false);//打开游戏的主界面//需要把当前登录的用户名传递给游戏界面new GameJFrame();} else {System.out.println("用户名或密码错误");showJDialog("用户名或密码错误");}} else if (e.getSource() == register) {System.out.println("点击了注册按钮");} else if (e.getSource() == rightCode) {System.out.println("更换验证码");//获取一个新的验证码String code = CodeUtil.getCode();rightCode.setText(code);}}public void showJDialog(String content) {//创建一个弹框对象JDialog jDialog = new JDialog();//给弹框设置大小jDialog.setSize(200, 150);//让弹框置顶jDialog.setAlwaysOnTop(true);//让弹框居中jDialog.setLocationRelativeTo(null);//弹框不关闭永远无法操作下面的界面jDialog.setModal(true);//创建Jlabel对象管理文字并添加到弹框当中JLabel warning = new JLabel(content);warning.setBounds(0, 0, 200, 150);jDialog.getContentPane().add(warning);//让弹框展示出来jDialog.setVisible(true);}//按下不松@Overridepublic void mousePressed(MouseEvent e) {if (e.getSource() == login) {login.setIcon(new ImageIcon("拼图小游戏_image\\image\\login\\登录按下.png"));} else if (e.getSource() == register) {register.setIcon(new ImageIcon("拼图小游戏_image\\image\\login\\注册按下.png"));}}//松开按钮@Overridepublic void mouseReleased(MouseEvent e) {if (e.getSource() == login) {login.setIcon(new ImageIcon("jigsawgame\\image\\login\\登录按钮.png"));} else if (e.getSource() == register) {register.setIcon(new ImageIcon("jigsawgame\\image\\login\\注册按钮.png"));}}//鼠标划入@Overridepublic void mouseEntered(MouseEvent e) {}//鼠标划出@Overridepublic void mouseExited(MouseEvent e) {}//判断用户在集合中是否存在public boolean contains(User userInput){for (int i = 0; i < allUsers.size(); i++) {User rightUser = allUsers.get(i);if(userInput.getUsername().equals(rightUser.getUsername()) && userInput.getPassword().equals(rightUser.getPassword())){//有相同的代表存在,返回true,后面的不需要再比了return true;}}//循环结束之后还没有找到就表示不存在return false;}}
注册代码
package ui;import domain.User;
import util.CodeUtil;import javax.swing.*;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.io.*;
import java.util.ArrayList;
import java.util.List;public class RegisterJFrame extends JFrame implements MouseListener {ArrayList<User> list = new ArrayList<>();//提升三个输入框的变量的作用范围,让这三个变量可以在本类中所有方法里面可以使用。JTextField username = new JTextField();JTextField password = new JTextField();JTextField rePassword = new JTextField();//提升两个按钮变量的作用范围,让这两个变量可以在本类中所有方法里面可以使用。JButton submit = new JButton();JButton reset = new JButton();public RegisterJFrame() throws IOException {user();initFrame();initView();setVisible(true);}public void user() throws IOException {File file = new File("user.txt");file.createNewFile();BufferedReader br = new BufferedReader(new FileReader("user.txt"));String str;while ((str = br.readLine()) != null) {String[] user = str.split("&");//nameString name = user[0].split("=")[1];//passwordString password = user[1].split("=")[1];list.add(new User(name, password));}}@Overridepublic void mouseClicked(MouseEvent e) {//获取输入框中的内容String userNameStr = username.getText();String passWordStr = password.getText();String rePasswordText = rePassword.getText();if (e.getSource() == submit){//注册System.out.println("注册");//判断输入框是否有空if ((userNameStr.length() == 0) || (passWordStr.length() == 0) || (rePasswordText.length() == 0)){showDialog("账号或密码不能为空");//清空密码password.setText("");rePassword.setText("");} else if (!passWordStr.equals(rePasswordText)) {showDialog("密码不一致");//清空密码rePassword.setText("");} else if (!tfUsername(userNameStr)) { //账户已存在showDialog("账号已存在");} else {try {//将数据存入本地文件中User(userNameStr,passWordStr);showDialog("注册成功");this.setVisible(false);new LoginJFrame();} catch (IOException ex) {throw new RuntimeException(ex);}}//}else if(e.getSource() == reset){//重置System.out.println("重置");password.setText("");rePassword.setText("");username.setText("");}}/** 将数据账号数据存入本地文件中* 参数1:账号 参数2:密码* */private void User(String name , String password) throws IOException {String user = "name="+name+"&password="+password;BufferedWriter bw = new BufferedWriter(new FileWriter("user.txt",true));bw.write(user);bw.newLine();bw.close();}/** 检测账号是否存在* 返回值 boolean* 传入 username* */private boolean tfUsername(String userName){for (User user : list) {if(user.getUsername().equals(userName))return false;}return true;}@Overridepublic void mousePressed(MouseEvent e) {if (e.getSource() == submit) {submit.setIcon(new ImageIcon("拼图小游戏_image\\image\\register\\注册按下.png"));} else if (e.getSource() == reset) {reset.setIcon(new ImageIcon("拼图小游戏_image\\image\\register\\重置按下.png"));}}@Overridepublic void mouseReleased(MouseEvent e) {if (e.getSource() == submit) {submit.setIcon(new ImageIcon("拼图小游戏_image\\image\\register\\注册按钮.png"));} else if (e.getSource() == reset) {reset.setIcon(new ImageIcon("拼图小游戏_image\\image\\register\\重置按钮.png"));}}@Overridepublic void mouseEntered(MouseEvent e) {}@Overridepublic void mouseExited(MouseEvent e) {}private void initView() {//添加注册用户名的文本JLabel usernameText = new JLabel(new ImageIcon("拼图小游戏_image\\image\\login\\用户名.png"));usernameText.setBounds(85, 135, 80, 20);//添加注册用户名的输入框username.setBounds(195, 134, 200, 30);//添加注册密码的文本JLabel passwordText = new JLabel(new ImageIcon("拼图小游戏_image\\image\\register\\注册密码.png"));passwordText.setBounds(97, 193, 70, 20);//添加密码输入框password.setBounds(195, 195, 200, 30);//添加再次输入密码的文本JLabel rePasswordText = new JLabel(new ImageIcon("拼图小游戏_image\\image\\register\\再次输入密码.png"));rePasswordText.setBounds(64, 255, 95, 20);//添加再次输入密码的输入框rePassword.setBounds(195, 255, 200, 30);//注册的按钮submit.setIcon(new ImageIcon("拼图小游戏_image\\image\\register\\注册按钮.png"));submit.setBounds(123, 310, 128, 47);submit.setBorderPainted(false);submit.setContentAreaFilled(false);submit.addMouseListener(this);//重置的按钮reset.setIcon(new ImageIcon("拼图小游戏_image\\image\\register\\重置按钮.png"));reset.setBounds(256, 310, 128, 47);reset.setBorderPainted(false);reset.setContentAreaFilled(false);reset.addMouseListener(this);//背景图片JLabel background = new JLabel(new ImageIcon("拼图小游戏_image\\image\\background.png"));background.setBounds(0, 0, 470, 390);this.getContentPane().add(usernameText);this.getContentPane().add(passwordText);this.getContentPane().add(rePasswordText);this.getContentPane().add(username);this.getContentPane().add(password);this.getContentPane().add(rePassword);this.getContentPane().add(submit);this.getContentPane().add(reset);this.getContentPane().add(background);}private void initFrame() {//对自己的界面做一些设置。//设置宽高setSize(488, 430);//设置标题setTitle("拼图游戏 V1.0注册");//取消内部默认布局setLayout(null);//设置关闭模式setDefaultCloseOperation(3);//设置居中setLocationRelativeTo(null);//设置置顶setAlwaysOnTop(true);}//只创建一个弹框对象JDialog jDialog = new JDialog();//因为展示弹框的代码,会被运行多次//所以,我们把展示弹框的代码,抽取到一个方法中。以后用到的时候,就不需要写了//直接调用就可以了。public void showDialog(String content){if(!jDialog.isVisible()){//把弹框中原来的文字给清空掉。jDialog.getContentPane().removeAll();JLabel jLabel = new JLabel(content);jLabel.setBounds(0,0,200,150);jDialog.add(jLabel);//给弹框设置大小jDialog.setSize(200, 150);//要把弹框在设置为顶层 -- 置顶效果jDialog.setAlwaysOnTop(true);//要让jDialog居中jDialog.setLocationRelativeTo(null);//让弹框jDialog.setModal(true);//让jDialog显示出来jDialog.setVisible(true);}}
}
游戏代码
import java.io.IOException;import ui.GameJFrame;
import ui.LoginJFrame;
import ui.RegisterJFrame;/*** @ClassName: App* @Author: Kox* @Data: 2023/1/30* @Sketch:*/
public class App {public static void main(String[] args) throws IOException {// 登录界面new LoginJFrame();// 注册界面new RegisterJFrame();// 游戏界面new GameJFrame();}
}