文章目录
- 4. LinkedList的模拟实现
- 5. LinkedList的使用
- 5.1 什么是LinkedList
- 5.2 LinkedList的使用
- 5.2.1 LinkedList的构造
- 5.2.2 LinkedList的其他常用方法介绍
- 5.2.3 LinkedList的遍历
- 6. ArrayList和LinkedList的区别
4. LinkedList的模拟实现
java">public class MyLinkedList {static class ListNode{public int value;public ListNode prev;public ListNode next;public ListNode(int value) {this.value = value;}}public ListNode head;public ListNode last;public boolean contains(int key){if (head == null){return false;}ListNode cur = head;while (cur != null){if (cur.value == key){return true;}cur = cur.next;}return false;}public int size(){ListNode cur = head;int size = 0;while (cur != null){size++;cur = cur.next;}return size;}public void display(){ListNode cur = head;while (cur != null){System.out.print(cur.value + " ");cur = cur.next;}System.out.println();}public void addFirst(int data){ListNode node = new ListNode(data);if (head == null){head = node;last = node;return;}head.prev = node;node.next = head;head = node;}public void addLast(int data){ListNode node = new ListNode(data);if (head == null){head = node;last = node;}else {last.next = node;node.prev = last;last = node;}}public void addIndex(int index, int data){if (index < 0 || index > size()){System.out.println("输入的下标不合理");return;}if (index == 0){addFirst(data);return;}if (index == size()){addLast(data);return;}ListNode cur = searchIndex(index);ListNode node = new ListNode(data);node.next = cur;node.prev = cur.prev;cur.prev.next = node;cur.prev = node;}private ListNode searchIndex(int index){ListNode cur = head;for (int i = 0; i < index; i++) {cur = cur.next;}return cur;}public void remove(int key){if (head == null){return;}ListNode cur = head;while (cur != null){if (cur.value == key){if (head.value == key){head = head.next;if (head != null){head.prev = null;}else {last = null;}}else {cur.prev.next = cur.next;if (cur.next == null){last = cur.prev;}else {cur.next.prev = cur.prev;}}return;}else {cur = cur.next;}}}public void removeAllKey(int key){if (head == null){return;}ListNode cur = head;while (cur != null){if (cur.value == key){if (head.value == key){head = head.next;if (head != null){head.prev = null;}else {last = null;}}else {cur.prev.next = cur.next;if (cur.next == null){last = cur.prev;}else {cur.next.prev = cur.prev;}}}cur = cur.next;}}public void clear(){ListNode cur = head;while (cur != null){ListNode curN = cur.next;cur.prev = null;cur.next = null;cur = curN;}head = null;last = null;}
}
5. LinkedList的使用
5.1 什么是LinkedList
LinkedList的底层是双向链表结构(链表后面介绍),由于链表没有将元素存储在连续的空间中,元素存储在单独的节点中,然后通过引用将节点连接起来了,因此在在任意位置插入或者删除元素时,不需要搬移元素,效率比较高。
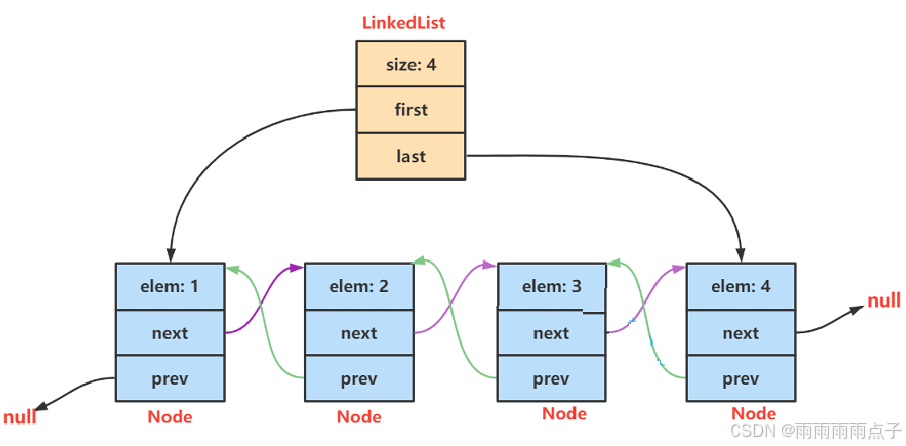
在集合框架中,LinkedList也实现了List接口,具体如下:
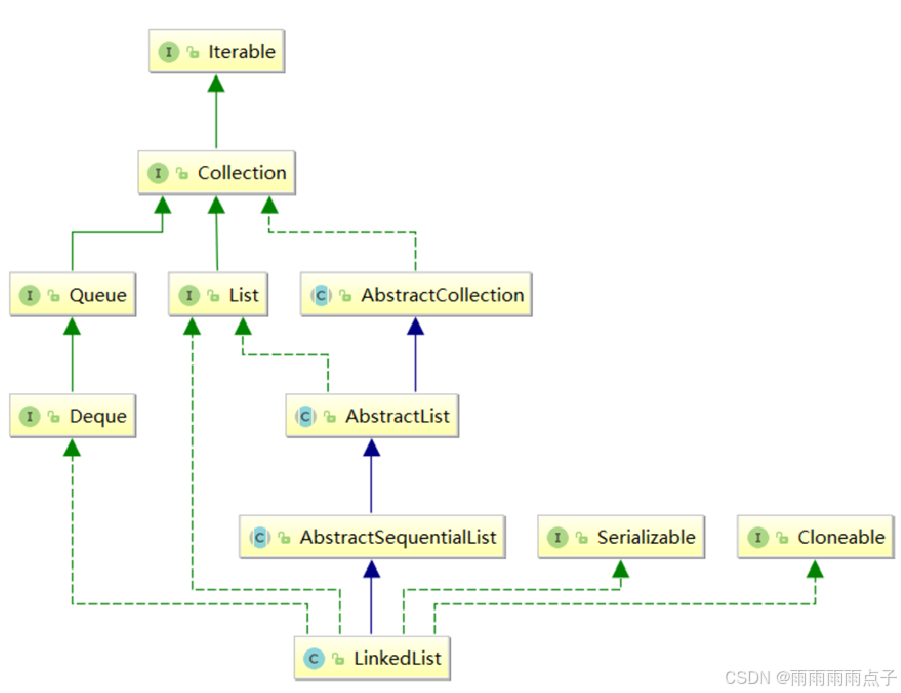
【说明】
- LinkedList实现了List接口
- LinkedList的底层使用了双向链表
- LinkedList没有实现RandomAccess接口,因此LinkedList不支持随机访问
- LinkedList的任意位置插入和删除元素时效率比较高,时间复杂度为O(1)
- LinkedList比较适合任意位置插入的场景
5.2 LinkedList的使用
5.2.1 LinkedList的构造
方法 | 解释 |
---|
LinkedList() | 无参构造 |
public LinkedList(Collection<?extends E>C) | 使用其他集合容器中元素构造List |
java"> public static void main(String[] args) {List<Integer> list1 = new LinkedList<>();List<String> list2 = new java.util.ArrayList<>();list2.add("JavaSE");list2.add("JavaWeb");list2.add("JavaEE");List<String> list3 = new LinkedList<>(list2);}
5.2.2 LinkedList的其他常用方法介绍
方法 | 解释 |
---|
boolean add(E e) | 尾插 e |
void add(int index, E element) | 将 e 插入到 index 位置 |
boolean addAll(Collection<? extends E> c) | 尾插 c 中的元素 |
E remove(int index) | 删除 index 位置元素 |
boolean remove(Object o) | 删除遇到的第一个 o |
E get(int index) | 获取下标 index 位置元素 |
E set(int index, E element) | 将下标 index 位置元素设置为 element |
void clear() | 清空 |
boolean contains(Object o) | 判断 o 是否在线性表中 |
int indexOf(Object o) | 返回第一个 o 所在下标 |
int lastIndexOf(Object o) | 返回最后一个 o 的下标 |
List subList(int fromIndex, int toIndex) | 截取部分List |
java">public static void main(String[] args) {LinkedList<Integer> list = new LinkedList<>();list.add(1); list.add(2);list.add(3);list.add(4);list.add(5);list.add(6);list.add(7);System.out.println(list.size());System.out.println(list);list.add(0, 0); System.out.println(list);list.remove();list.removeFirst(); list.removeLast(); list.remove(1); System.out.println(list);if(!list.contains(1)){list.add(0, 1);}list.add(1);System.out.println(list);System.out.println(list.indexOf(1)); System.out.println(list.lastIndexOf(1)); int elem = list.get(0); list.set(0, 100); System.out.println(list);List<Integer> copy = list.subList(0, 3);System.out.println(list);System.out.println(copy);list.clear(); System.out.println(list.size());}
5.2.3 LinkedList的遍历
java"> public static void main(String[] args) {LinkedList<Integer> list = new LinkedList<>();list.add(1); list.add(2);list.add(3);list.add(4);list.add(5);list.add(6);list.add(7);System.out.println(list.size());for (int e:list) {System.out.print(e + " ");}System.out.println();ListIterator<Integer> it = list.listIterator();while(it.hasNext()){System.out.print(it.next()+ " ");}System.out.println();ListIterator<Integer> rit = list.listIterator(list.size());while (rit.hasPrevious()){System.out.print(rit.previous() +" ");}System.out.println();}
6. ArrayList和LinkedList的区别
不同点 | ArrayList | LinkedList |
---|
存储空间上 | 物理上一定连续 | 逻辑上连续,但物理上不一定连续 |
随机访问 | 支持O(1) | 不支持:O(N) |
头插 | 需要搬移元素,效率低O(N) | 只需修改引用的指向,时间复杂度为O(1) |
插入 | 空间不够时需要扩容 | 没有容量的概念 |
应用场景 | 元素高效存储+频繁访问 | 任意位置插入和删除频繁 |