电子地图应用广泛:
网约车 : 在网约车 场景中实现 准定位 、导航 、司乘同显 ,精准计费
智慧物流、生活服务等,本专题课程囊括各类应用场景
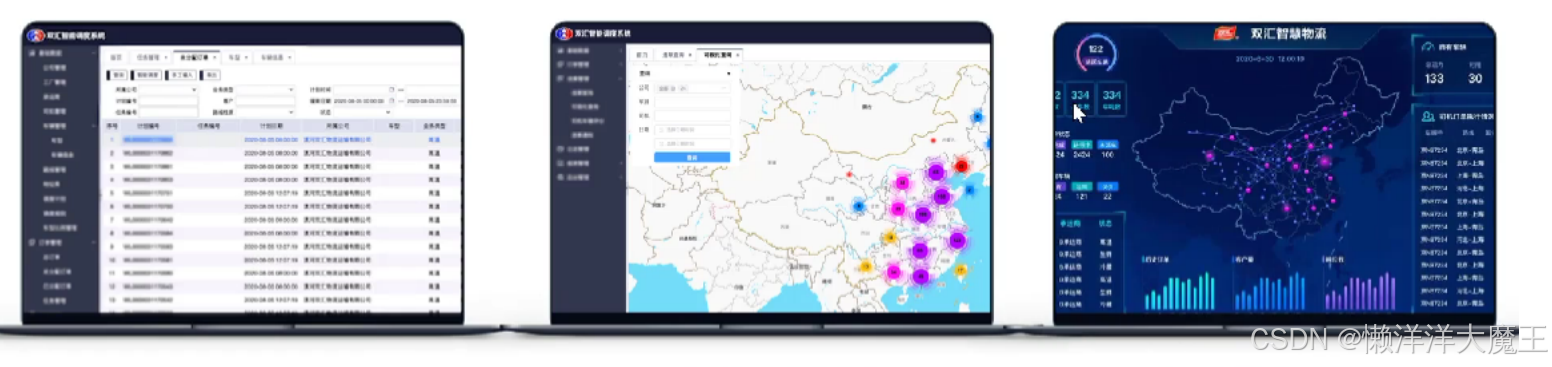
学习 电子地图解决方案,满足学员工作学习各类需求。
基础知识
学习 集成 地图之前需要掌握的知识 :
- 初步 掌握 Vue 基本使用
- 父子组件之间的传值
- 组件编写,
- html+ css
创建项目
- vue create [项目名]
- 选择我们需要的特性 : Babel 、 Router 、 VueX 注 : 自定义特性的原因是因为 以免小白 开启 ES-lint 检查
- 选择版本
- 确认选择
- 安装咱们需要的依赖
- npm i @amap/amap-jsapi-loader --savenpm i element-ui
开发依赖
npm install --save-dev less@4.0.0 less-loader@8.0.0
packjson.json
{
"name": "Auto Navi Map",
"version": "0.1.0",
"private": true,
"scripts": {
"serve": "vue-cli-service serve",
"build": "vue-cli-service build"
},
"dependencies": {
"@amap/amap-jsapi-loader": "^1.0.1",
"core-js": "^3.8.3",
"element-ui": "^2.15.8",
"vue": "^2.6.14",
"vue-router": "^3.5.1",
"vuex": "^3.6.2"
},
"devDependencies": {
"@vue/cli-plugin-babel": "~5.0.0",
"@vue/cli-plugin-router": "~5.0.0",
"@vue/cli-plugin-vuex": "~5.0.0",
"@vue/cli-service": "~5.0.0",
"less": "4.0.0",
"less-loader": "8.0.0",
"vue-template-compiler": "^2.6.14"
}
}
注册 高德地图API
官网地址 : https://lbs.amap.com
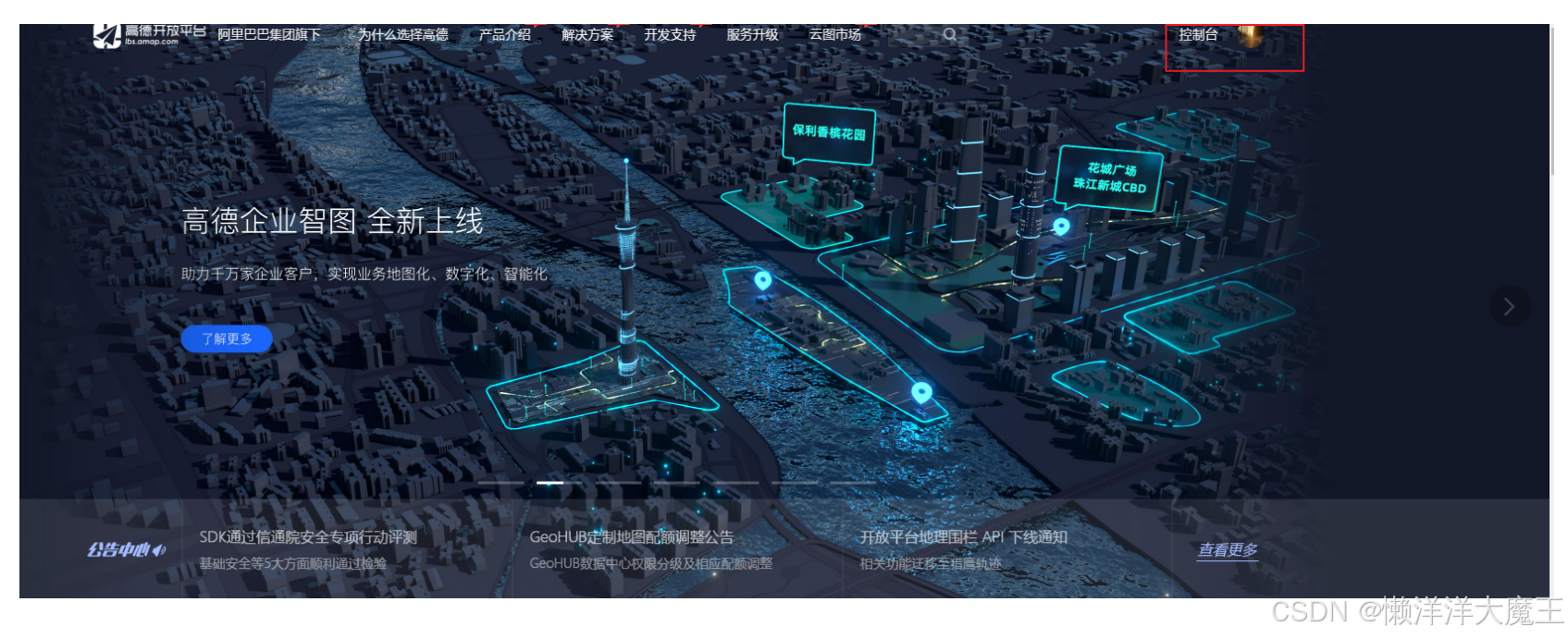
Key 名称:
官方使用 Vue教程 : https://lbs.amap.com/api/jsapi-v2/guide/webcli/map-vue1
构建 地图 区域展示
Home View
<template><div id="index_container"><div id="search_wrap"><search></search></div><div id="map_wrap"><map-container></map-container></div><div id="heatMap_wrap"><heat-map-search-box></heat-map-search-box></div>
<div id="shadow">
</div>
</div>
</template>
<script>
import MapContainer from
'@/components/MapContainer/MapContainer.vue'
import Search from '@/components/MapContainer/Search.vue'
import HeatMapSearchBox from
'@/components/heatMapSearchBox/heatMapSearchBox.vue'
export default {
components: { MapContainer, Search, HeatMapSearchBox }
}
</script>
<style lang="less" scoped>
#index_container {
overflow: hidden;
background-color: rgb(207, 229, 248);
height: 100%;
width: 100%;
position: relative;
#map_wrap {
z-index: 10;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
height: 60%;
width: 60%;
}
#search_wrap {
z-index: 10;
position: absolute;
top: 10%;
left: 50%;
height: 60px;
width: 400px;
transform: translate(-50%, -50%);
}
#shadow {
height: 80%;
width: 80%;
position: absolute;
top: 40%;
left: 50%;
transform: translate(-50%, -50%);
background-color: rgb(255, 255, 255);
}
#heatMap_wrap {
z-index: 12;
position: absolute;
top: 15%;
left: 50%;
transform: translate(-50%, -50%);
}
}
</style>
import MapContainer from '@/components/MapContainer/MapContainer.vue'
<template>
<div id="container"></div>
</template>
<script>
import AMapLoader from '@amap/amap-jsapi-loader'
import bus from '@/bus/bus'
window._AMapSecurityConfig = {
securityJsCode: '1dbf90857c868c4b99c98165da706acb'
}
export default {
data() {
return {
map: null,
autoOptions: {
input: ''
},
searchPlaceInput: '',
auto: null,
placeSearch: null,
district: null,
polygons: [],
showHeatOrNot: false,
heatmap: null,
heatmapList: null
}
},
methods: {
initMap() {
AMapLoader.load({
key: '02c854342b6ea9c8f1e85cb0a6f2882f', // 申请好的Web端开发
者Key,首次调用 load 时必填
version: '2.0', // 指定要加载的 JSAPI 的版本,缺省时默认为 1.4.15
plugins: ['AMap.ToolBar', 'AMap.Scale', 'AMap.HawkEye',
'AMap.MapType', 'AMap.Geolocation', 'AMap.AutoComplete',
'AMap.PlaceSearch'] // 需要使用的的插件列表,如比例尺'AMap.Scale'等
})
.then(AMap => {
this.map = new AMap.Map('container', {
//设置地图容器id
viewMode: '3D', //是否为3D地图模式
zoom: 10, //初始化地图级别
center: [121.473667, 31.230525] //初始化地图中心点位置
})
this.map.addControl(new AMap.Scale())
this.map.addControl(new AMap.ToolBar())
this.map.addControl(new AMap.HawkEye())
this.map.addControl(new AMap.MapType())
this.map.addControl(new AMap.Geolocation())
this.auto = new AMap.AutoComplete(this.autoOptions)
this.placeSearch = new AMap.PlaceSearch({
map: this.map
}) //构造地点查询类
this.auto.on('select', this.select)
})
.catch(e => {
console.log(e)
})
},
select(e) {
this.placeSearch.setCity(e.poi.adcode)
this.placeSearch.search(e.poi.name) //关键字查询查询
this.drawBounds(e.poi.name)
this.map.setZoom(16, true, 1)
},
// 行政区区域划分
drawBounds(newValue) {
//加载行政区划插件
if (!this.district) {
//实例化DistrictSearch
var opts = {
subdistrict: 1, //获取边界不需要返回下级行政区
extensions: 'all', //返回行政区边界坐标组等具体信息
level: 'district' //查询行政级别为 市
}
this.map.plugin(['AMap.DistrictSearch'], () => {
this.district = new AMap.DistrictSearch(opts)
})
// this.district = new AMap.DistrictSearch(opts)
}
//行政区查询
this.district.search(newValue, (status, result) => {
console.log(result)
if (result != null) {
this.feedBack('区域搜索成功', 'success')
if (this.polygons != null) {
this.map.remove(this.polygons) //清除上次结果
this.polygons = []
}
var bounds = result.districtList[0].boundaries
if (bounds) {
for (var i = 0, l = bounds.length; i < l; i++) {
//生成行政区划polygon
var polygon = new AMap.Polygon({
strokeWeight: 1,
path: bounds[i],
fillOpacity: 0.4,
fillColor: '#80d8ff',
strokeColor: '#0091ea'
})
this.polygons.push(polygon)
}
}
this.map.add(this.polygons)
this.map.setFitView(this.polygons) //视口自适应
} else {
this.feedBack('区域搜索失败', 'error')
}
})
},
feedBack(msg, feedBackType) {
this.$message({
showClose: true,
message: msg,
type: feedBackType
})
},
showHeatMap() {
this.map.plugin(['AMap.PlaceSearch'], () => {
//构造地点查询类
var placeSearch = new AMap.PlaceSearch({
pageSize: 50, // 单页显示结果条数
pageIndex: 1, // 页码
city: this.searchPlaceInput, // 兴趣点城市
citylimit: true //是否强制限制在设置的城市内搜索
//map: this.map, // 展现结果的地图实例
// panel: 'panel', // 结果列表将在此容器中进行展示。
// autoFitView: true // 是否自动调整地图视野使绘制的 Marker点都
处于视口的可见范围
})
//关键字查询
placeSearch.search('商场', (status, result) => {
// console.log(result)
this.getHotChartPos('商场', result)
})
})
this.$notify({
title: '成功',
message: '热力图获取成果,但是由于电脑性能,我们仅加载部分数据',
type: 'success'
})
},
getHotChartPos(detail, result) {
let lengthSize = result.poiList.pageSize
const count = result.poiList.count
// const lengthPage = count / lengthSize
if (lengthSize > count) {
lengthSize = count
}
for (var n = 0; n < lengthSize; n++) {
// this.map.plugin(['AMap.PlaceSearch'], () => {
//构造地点查询类
var realSearch = new AMap.PlaceSearch({
pageSize: 50, // 单页显示结果条数
pageIndex: n + 1, // 页码
city: this.searchPlaceInput, // 兴趣点城市
citylimit: true //是否强制限制在设置的城市内搜索
// map: this.map, // 展现结果的地图实例
// panel: 'panel', // 结果列表将在此容器中进行展示。
// autoFitView: true // 是否自动调整地图视野使绘制的 Marker点都
处于视口的可见范围
})
realSearch.search(detail, (status, result) => {
// for (var j = 0; j < 50; j++) {
// this.map.remove(this.map.getAllOverlays('marker'))
//var centerPoint = [result.poiList.pois[j].location.lng,
result.poiList.pois[j].location.lat]
// console.log(result)
//热力图
this.showHatChart(result)
// }
})
}
},
showHatChart(result) {
var info = []
for (let i = 0; i < 50; i++) {
info.push({
lng: result.poiList.pois[i].location.lng,
lat: result.poiList.pois[i].location.lat,
count: 3 * 50 * Math.round(Math.random() * (10 - 2) + 2)
})
}
this.map.plugin(['AMap.HeatMap'], () => {
//初始化heatmap对象
this.heatmap = new AMap.HeatMap(this.map, {
radius: 56, //给定半径
opacity: [0, 0.5]
/*,
gradient:{
0.5: 'blue',
0.65: 'rgb(117,211,248)',
0.7: 'rgb(0, 255, 0)',
0.9: '#ffea00',
1.0: 'red'
}
*/
})
//设置数据集
this.heatmap.setDataSet({
data: info,
max: 100
})
this.heatmapList.push(this.heatmap)
this.heatmap.show()
})
}
},
mounted() {
//DOM初始化完成进行地图初始化
this.initMap()
},
created() {
bus.$on('shareUserInput', val => {
this.autoOptions.input = val.inputId
this.searchPlaceInput = val.userInput
})
bus.$on('shareHeatMapShow', val => {
this.showHeatOrNot = val
})
},
watch: {
searchPlaceInput(newValue) {
if (newValue != null) {
console.log(newValue)
this.placeSearch.search(newValue)
this.drawBounds(newValue)
this.map.setZoom(16, true, 1)
}
},
showHeatOrNot(newValue) {
if (newValue) {
this.showHeatMap()
} else {
this.heatmap.hide()
}
}
}
}
</script>
<style lang="less" scoped>
#container {
padding: 0px;
margin: 0px;
width: 100%;
height: 100%;
}
</style>
官方 提供的输入 API : 输入提示 - 输入提示 - 示例中心 -JS API 示例 | 高德地图 API (amap.com)
本章主要介绍以下内容:
1. 输入提示插件 AMap.Autocomplete
2. POI 搜索插件 AMap.PlaceSearch
App.vue 主渲染页面 结果
<template><div id="app"><router-view/></div>
</template>
<style>
html,body{margin: 0px;padding: 0px;width: 100%;height: 100%;}
#app{width: 100%;height: 100%!important;margin: 0px;padding:
0px;position: absolute;background: #a0cbff;
background: url(assets/2.png) no-repeat center center;
background-size:100% 100%;
}#nprogress .bar {background: rgb(96, 47, 231) !important;
}
</style>
构建搜索结果
import Search from '@/components/MapContainer/Search.vue
<template><div id="search_container"><el-input v-model="inputObject.userInput"
:id="inputObject.inputId" placeholder="请输入你要查找的关键词"
type="text"></el-input><!-- <input type="text" placeholder="" > --><el-button type="primary" id="searchBtn"@click="send">search</el-button></div>
</template>
<script>
import bus from '@/bus/bus'
export default {data() {return {inputObject: {userInput: '',inputId: 'searchInput'}}},methods: {send() {bus.$emit('shareUserInput', this.inputObject)}},mounted() {this.send()}
}
</script>
<style lang="less" scoped>
#search_container {height: 100%;width: 100%;display: flex;justify-content: baseline;align-items: center;
#searchInput {height: 30px;width: 300px;
}
#searchBtn {// height: 30px;width: 100px;}
}
</style>
css 写法
#search_container {height: 100%;width: 100%;display: flex;justify-content: baseline;align-items: center;
}
#search_container #searchInput {height: 30px;width: 300px;
}
#search_container #searchBtn {width: 100px;
}
热力图展示
<template><div id="heat_map_search_box_container"><el-checkbox v-model="checked" @change="send">热力图显示</elcheckbox></div>
</template>
<script>
import bus from '@/bus/bus'
export default {data() {return {checked: false}},methods: {send() {bus.$emit('shareHeatMapShow', this.checked)}}
}
</script>
<style lang="less" scoped>
#heat_map_search_box_container {height: 100%;width: 100%;position: relative;
}
</style>
路由
import Vue from 'vue'
import VueRouter from 'vue-router'
import HomeView from '../views/HomeView.vue'
Vue.use(VueRouter)
const routes = [{path: '/',name: 'home',component: HomeView},{path: '/about',name: 'about',// route level code-splitting// this generates a separate chunk (about.[hash].js) for thisroute// which is lazy-loaded when the route is visited.component: () => import(/* webpackChunkName: "about" */
'../views/AboutView.vue')}
]
const router = new VueRouter({mode: 'history',base: process.env.BASE_URL,routes
})
export default router
主页面 App 官方封装组件使用
<template><div id="app"><router-view></router-view></div>
</template>
<script>
export default {}
</script>
<style lang="less" scoped>
#app {height: 100vh;width: 100vw;
}
</style>
官方封装组件使用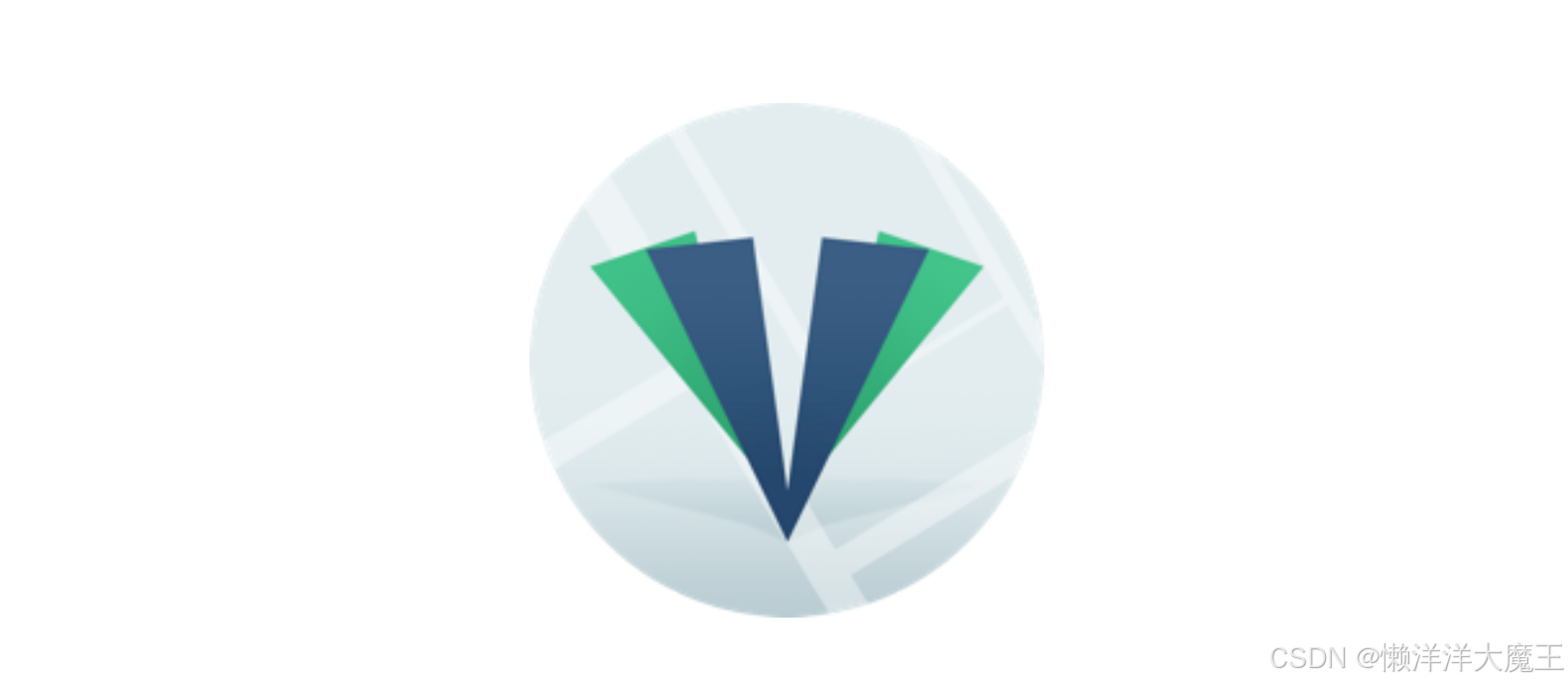
https://github.com/ElemeFE/vue-amap
Cesium
CesiumJS 是一款用于创建虚拟场景的 3D 地理信息平台。目标是用于创建以基于
Web 的地图动态数据可视化。目前尽力提升平台的性能、准确率、虚拟化能
力、易用性以及平台的各种支持。