面向对象程序设计基本特点
特点:
- 抽象(数据抽象,行为抽象)
数据抽象:int hour,int minute.....,车:长,宽,高....
功能抽象:showTime(),setTime() .....车:刹车,加速....- 封装
private , public , protect- 继承(将已有的代码拉来编写新的类,使其变得更加具体更详细的说明,一般与特殊的关系)
- 多态
类
定义:
class 类名称
{
public :
外部接口
protected:
保护型成员
private:
私有成员
} ;
访问权限:private , public , protected
对象
类名 对象名 :
Clock myClock ; // 声明了一个时钟类型的myClock
调用时
对象名 . 函数成员名
myClock . showTime () ; // 在类的外部只能访问共有成员,在类的成员函数中,可以访问类的全部成员
类的成员函数
- 成员函数的实现
与普通函数不同,类的成员函数名需要用类名来限制 Clock :: showTime()- 带默认形参值的成员函数
- 内联成员函数
内联函数的声明有两种:隐式声明和显式声明
隐式声明: 直接将函数放在结构体里
显式声明:采用关键字inline,在函数的返回值类型前添加inline
🙋♂️实例(时钟类):
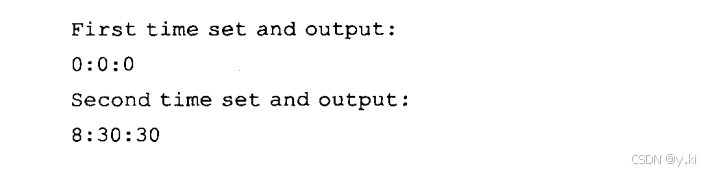
构造函数
构造函数的作用:在对象创建时利用特定的值构建对象,将对象初始化为一个特定的状态
将其封装出去
主函数部分:
int main()
{
Clock c(0,0,0); // 声明变量并赋值
c.showTime();
c.setTime(3,29,20);}
初始化赋值,hour在开空间的同时将h赋值,minute在开空间的同时传参m
🙋♂️实例(α+βi):
#include <iostream> #define P 3.1415926 #include "circle.h" using namespace std;class Complex { public:Complex(double r = 0,double i =0);void setReal(double r);void setImage(double i);void show(){cout<<real<<"+"<<image<<"i"<<endl;}private:double real;double image; }; void Complex::setReal(double r) {real= r; } void Complex::setImage(double i) {image = i; } Complex ::Complex(double r, double i):real(r),image(i) {} int main() {Complex c(10,20);c.show(); }
复制构造函数
复制构造函数定义与声明:class 类名
{
public :
类名(形参表); //构造函数
类名(类名 & 对象名); //复制构造函数
......
};
类名 ::类名(类名 & 对象名); //复制构造函数的实现
{
函数体
}
复制结构函数的实现:Point :: Point(Point &p)
{
x = p.x;
y = p.y;
cout << " Calling the copy constructor " << endl;
}
示例:
using namespace std; class Point { public:Point (int x1 = 0,int y1 = 0)//构造函数{x = x1;y = y1;}Point (Point &p);//复制构造函数int getX()//获取private中的数值{return x;}int getY(){return y;} private:int x,y; };
//成员函数的实现 Point ::Point(Point &p) {x = p.x;y = p.y;cout<<"Calling the constructor"<<endl; }
//形参为point类对象的函数 void fun1(Point p) {cout <<p.getX()<<endl; }
//返回值为point类对象的函数 Point fun2() {Point a(1,2);return a; }
//主函数 int main() {Point a(4,5);//第一个对象Point b =a; //情况1 用a初始化b,第一次调用复制构造函数cout<<b.getX()<<endl;fun1(b); //情况2 对象b作为fun1的实参 第二次调用复制构造函数b = fun2(); //情况3 函数的返回值是类对象,函数返回时,调用复制构造函数cout<<b.getX()<<endl;return 0;}
析构函数
析构函数的特点:
#include <iostream> class MyClass { public: MyClass() { std::cout << "Constructor called\n"; } ~MyClass() { std::cout << "Destructor called\n"; // 清理工作,比如释放资源 } }; int main() { MyClass obj; // 调用构造函数 // 当obj的生命周期结束时(main函数返回时),调用析构函数 return 0; }
- ~ 没有返回类型,也不接受任何参数
- 当一个对象的生命周期结束时,析构函数会自动被调用。这包括局部对象在离开其作用域时、动态分配的对象被
delete
删除时、全局对象在程序结束时、以及容器中的对象被销毁时等情况。- 如果没有定义析构函数编译器会自动默认生成,但如果定义了析构函数编辑器便不会生产
- 析构函数不能被重载,每个类只能有一个析构函数
- 析构函数通常被声明为
public
,因为即使你创建了类的对象,也需要外部代码(如delete
表达式)来销毁对象并调用析构函数。但是,在特定情况下(如基类的析构函数在派生类中被隐藏时),可能会将析构函数声明为protected
或private
🙋♂️实例(坐标显示,圆环面积)
#include <iostream>
#define P 3.1415926
#include "circle.h"
using namespace std;
class Point
{
public:Point(int x = 0,int y = 0);Point(const Point &other);void setX(int x);void setY(int y);int getX(){return m_x;}int getY(){return m_y;}void show();~Point(){cout << "~"<< endl;}
private:int m_x,m_y;
};void Point ::show()
{cout << "(" << m_x << "," << m_y << ")" << endl;
}
Point ::Point(int x, int y):m_x(x),m_y(y)
{cout <<" p "<<endl;
}
Point ::Point(const Point &other):m_x(other.m_x),m_y(other.m_y)
{cout << "p1 " << endl;
}int main()
{Point p(10,20);p.show();Point p1(p);p1.show();return 0;
}
求圆环的面积:
#include <iostream> #define P 3.1415926 //宏定义using namespace std;class Circle //声明定义类Circle及其数据和方法 { public: //外部接口double area() // 计算面积{return radius * radius * P;}void setRaius(double r) // 获取private的数据{radius = r;} private:double radius; };int main() {Circle c1;Circle c2;c1.setRaius(20);c2.setRaius(10);cout <<c1.area() - c2.area() <<endl;cout << "Hello World!" << endl;return 0; }
封装:
类的组合
Circle :: Circle (double r) : radius ( r ) { }radius 开空间的同时将 r 赋值
🙋♂️练习(线段长度):
Line 类包含p1 , p2作为其数据成员,Line类具有计算线段长度的功能
#include <iostream>using namespace std;
class Point //point 类定义
{
public:Point(int x = 0,int y = 0); //构造函数Point(const Point &other); void setX(int x);void setY(int y);int getX(){return m_x;}int getY(){return m_y;}void show();~Point(){cout << "~Point"<< endl;}
private:int m_x,m_y;
};void Point ::show()
{cout << "(" << m_x << "," << m_y << ")" << endl;
}
Point ::Point(int x, int y):m_x(x),m_y(y)
{cout <<" p(int,int) "<<endl;
}
//复制构造函数的实现
Point ::Point(const Point &other):m_x(other.m_x),m_y(other.m_y)
{cout << "point &other " << endl;
}//类的组合
class Line //line类的定义
{
public: //外部接口Line(int x1,int y1,int x2,int y2):m_p1(x1,y1),m_p2(x2,y2){cout << "line(int,int,int,int)" <<endl;}Line(const Point &p1,const Point &p2):m_p1(p1),m_p2(p2){cout<<"point &p"<<endl;}void show(){m_p1.show();cout<<"->";m_p2.show();cout<<endl;}~Line(){cout<<"~Line"<<endl;}
private: Point m_p1,m_p2; //point类的对象m_p1,m_p2
};
int main()
{Point p1(3,4); Point p2(9,10); //建立point类对象Line l(p1,p2); //建立line类的对象l.show();return 0;
}