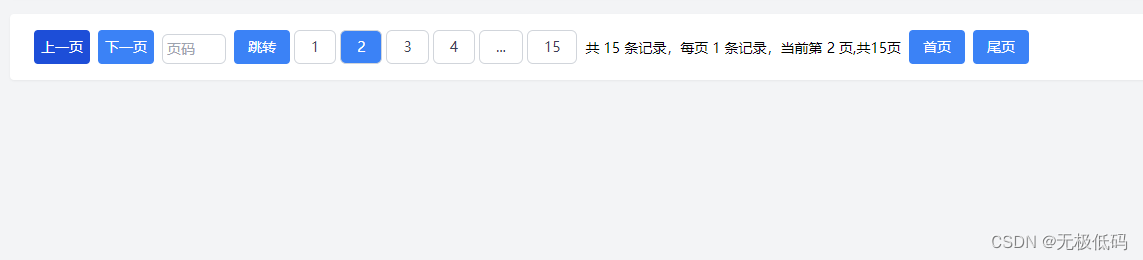
javascript">// 分页插件类
class PaginationPlugin {constructor(fetchDataURL, options = {}) {this.fetchDataURL = fetchDataURL;this.options = {containerId: options.containerId || 'paginationContainer',dataSizeAttr: options.dataSizeAttr || 'toatalsize', // 修改为实际API返回的数据属性名pageSizeAttr: options.pageSizeAttr || 'size',onPageChange: options.onPageChange || (() => {}),};this.paginationData = {};this.totalPages=0;}async init() {const initialData = await this.fetchData(1);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);}createPagination(containerId) {// ... 保留原有的创建分页组件逻辑 ..const container = document.getElementById(containerId);container.innerHTML=''// 创建上一页按钮const prevPageButton = document.createElement('button');prevPageButton.id = 'prevPage';prevPageButton.textContent = '上一页';prevPageButton.classList.add('bg-blue-200','w-14', 'hover:bg-blue-700', 'text-white','text-sm', 'py-1', 'px-1', 'rounded', 'ml-2');// 创建下一页按钮const nextPageButton = document.createElement('button');nextPageButton.id = 'nextPage';nextPageButton.textContent = '下一页';nextPageButton.classList.add('bg-blue-500', 'w-14','hover:bg-blue-700', 'text-white', 'text-sm','py-1', 'px-1', 'rounded', 'ml-2');// 创建跳转按钮const gotoPageButton = document.createElement('button');gotoPageButton.id = 'gotoPageButton';gotoPageButton.textContent = '跳转';gotoPageButton.classList.add('bg-blue-500','w-14', 'hover:bg-blue-700', 'text-white', 'text-sm', 'py-1', 'px-1', 'rounded', 'ml-2');// 创建输入框const gotoPageInput = document.createElement('input');gotoPageInput.id = 'gotoPageInput';gotoPageInput.type = 'number';gotoPageInput.placeholder = '页码';gotoPageInput.classList.add('w-16', 'p-1', 'mt-1', 'border','text-sm', 'border-gray-300', 'rounded-md', 'ml-2');// 创建分页按钮容器const paginationContainer = document.createElement('div');paginationContainer.id = 'pagination';paginationContainer.classList.add('flex', 'justify-center');// 创建分页按钮列表let pages = [];const visibleRange = 5; // 显示5个完整页码const halfVisibleRange = Math.floor(visibleRange / 2); // 对称显示,一半数量的完整页码if (this.totalPages > visibleRange) {// 开始页码let startPage = this.paginationData.data.page - halfVisibleRange;if (startPage <= 0) {startPage = 1;}// 结束页码let endPage = this.paginationData.data.page + halfVisibleRange;if (endPage > this.totalPages) {endPage = this.totalPages;startPage = Math.max(startPage - (endPage - this.totalPages), 1);}// 添加开始页码之前的页码if (startPage > 1) {pages.push(1);if (startPage > 2) {pages.push('...');}}// 添加中间页码for (let i = startPage; i <= endPage; i++) {pages.push(i);}// 添加结束页码之后的页码if (endPage < this.totalPages) {pages.push('...');pages.push(this.totalPages);}} else {for (let i = 1; i <= this.totalPages; i++) {pages.push(i);}}pages.forEach((page) => {const button = document.createElement('button');button.id = `page-${page}`;button.textContent = page;button.classList.add('px-4', 'py-1', 'border', 'border-gray-300','text-sm', 'rounded-md', 'text-gray-700','ml-1',);if (page === this.paginationData.data.page) {button.classList.remove('text-gray-700');button.classList.add('bg-blue-500', 'text-white');}paginationContainer.appendChild(button);});// 创建分页信息容器const pageInfoContainer = document.createElement('div');pageInfoContainer.id = 'pageInfoContainer';pageInfoContainer.classList.add('flex', 'text-sm','justify-end');// 创建分页信息const pageInfo = document.createElement('span');pageInfo.id = 'pageInfo';pageInfo.textContent = `共 ${paginationData.data.toatalsize} 条记录,每页 ${paginationData.data.size} 条记录,当前第 ${paginationData.data.page} 页,共${paginationData.data.totalpage}页`;pageInfoContainer.appendChild(pageInfo);pageInfo.classList.add('ml-2','mt-2');// 创建跳转到第一页按钮const firstPageButton = document.createElement('button');firstPageButton.id = 'firstPageButton';firstPageButton.textContent = '首页';firstPageButton.classList.add('bg-blue-500','w-14', 'hover:bg-blue-700', 'text-white','text-sm', 'py-1', 'px-1', 'rounded', 'ml-2');pageInfoContainer.appendChild(firstPageButton);// 创建跳转到最后一页按钮const lastPageButton = document.createElement('button');lastPageButton.id = 'lastPageButton';lastPageButton.textContent = '尾页';lastPageButton.classList.add('bg-blue-500','w-14', 'hover:bg-blue-700', 'text-white','text-sm', 'py-1', 'px-1', 'rounded', 'ml-2');pageInfoContainer.appendChild(lastPageButton);// 将所有元素添加到容器中container.appendChild(prevPageButton);container.appendChild(nextPageButton);container.appendChild(gotoPageInput);container.appendChild(gotoPageButton);container.appendChild(paginationContainer);container.appendChild(pageInfoContainer);}// 更新选中的分页按钮updateSelectedPage(page, containerId) {const container = document.getElementById(containerId);const buttons = container.querySelectorAll('#pagination button');buttons.forEach((button) => {if (button.id === `page-${page}`) {button.classList.remove('text-gray-700');button.classList.add('bg-blue-500', 'text-white');} else {button.classList.add('text-gray-700');button.classList.remove('bg-blue-500', 'text-white');}});}bindEventListeners(containerId) {const prevPageButton = document.getElementById('prevPage');const nextPageButton = document.getElementById('nextPage');const gotoPageButton = document.getElementById('gotoPageButton');const gotoPageInput = document.getElementById('gotoPageInput');const pageInfoContainer = document.getElementById('pageInfoContainer');const firstPageButton = document.getElementById('firstPageButton');const lastPageButton = document.getElementById('lastPageButton');prevPageButton.addEventListener('click',async () => {const currentPage = parseInt(this.paginationData.data.page);if(currentPage==2){prevPageButton.classList.add('bg-blue-200');prevPageButton.classList.remove('bg-blue-500');}if(currentPage==1){alert("已经是第一页了");return;}if (currentPage > 1) {this.paginationData.data.page = currentPage - 1;const initialData =await this.fetchData( this.paginationData.data.page);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);this.updateSelectedPage(this.paginationData.data.page,containerId)}});nextPageButton.addEventListener('click', async() => {const currentPage = parseInt(this.paginationData.data.page);if( currentPage==this.totalPages){alert("已经是最后一页了");return;}prevPageButton.classList.remove('bg-blue-200');prevPageButton.classList.add('bg-blue-500');if (currentPage < this.totalPages) {const initialData =await this.fetchData( this.paginationData.data.page);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.paginationData.data.page = currentPage + 1;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);this.updateSelectedPage(this.paginationData.data.page,containerId)}});gotoPageButton.addEventListener('click', async() => {const input = document.getElementById('gotoPageInput');const page = parseInt(input.value);if (!isNaN(page) && page > 0 && page <= this.totalPages) {const initialData =await this.fetchData(page);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.paginationData.data.page = page;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);}});firstPageButton.addEventListener('click', async() => {this.paginationData.data.page = 1;const initialData =await this.fetchData(this.paginationData.data.page);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);});lastPageButton.addEventListener('click',async () => {const initialData =await this.fetchData(this.totalPages);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.paginationData.data.page = this.totalPages;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);});// ... 保留原有的事件绑定逻辑 ...const paginationButtons = document.querySelectorAll(`#${containerId} button[id^="page-"]`);paginationButtons.forEach((button) => {button.addEventListener('click', async (event) => {const targetPage = parseInt(event.target.id.replace('page-', ''));const initialData = await this.fetchData(targetPage);this.paginationData = initialData;this.totalPages=initialData.data.totalpage;this.paginationData.data.page =targetPage;this.options.onPageChange(initialData);this.createPagination(this.options.containerId);this.bindEventListeners(this.options.containerId);});});}async fetchData(page) {const url = `${this.fetchDataURL}&page=${page}&size=10`;try {const response = await fetch(url);const jsonData = await response.json();return jsonData;} catch (error) {console.error('There has been a problem with your fetch operation:', error);return null;}
}
}