文章目录
- 序言
- Spring 框架的核心概念
- Spring 框架的主要模块
- Spring Boot:简化 Spring 开发
- Spring Cloud:构建微服务架构
- 实际案例分析
- 结论
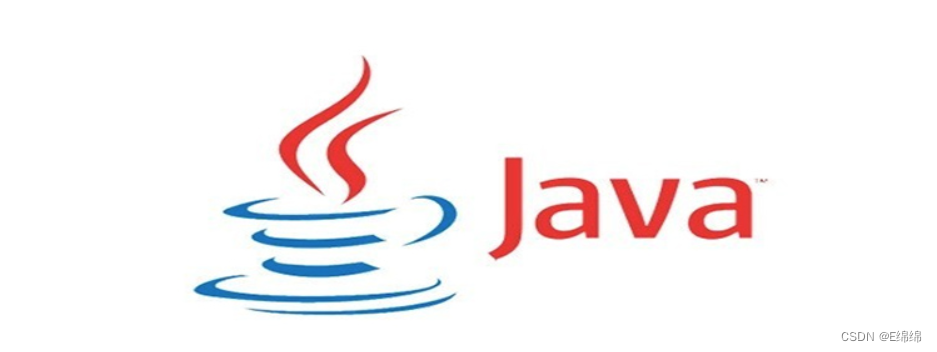
序言
Spring 框架自 2002 年发布以来,已经成为 Java 企业级开发的标准之一。它通过提供全面的基础设施支持,使开发者能够专注于应用程序的业务逻辑,实现更高效、更灵活的软件开发。本文将深入探讨 Spring 框架的核心概念、主要模块及其在实际开发中的应用,帮助读者全面了解这一强大的开发工具。
Spring 框架的核心概念
-
依赖注入 (Dependency Injection, DI)
依赖注入是 Spring 框架的核心理念之一,它通过控制反转 (Inversion of Control, IoC) 容器管理对象的生命周期和依赖关系。依赖注入使得组件之间的耦合度降低,增加了代码的可测试性和可维护性。
public class HelloWorld {private MessageService messageService;@Autowiredpublic void setMessageService(MessageService messageService) {this.messageService = messageService;}public void sayHello() {System.out.println(messageService.getMessage());} }
-
面向切面编程 (Aspect-Oriented Programming, AOP)
AOP 允许开发者将横切关注点 (cross-cutting concerns) 从业务逻辑中分离出来,如事务管理、安全检查、日志记录等。Spring 通过 AOP 模块,实现了切面与业务逻辑的无缝结合。
@Aspect public class LoggingAspect {@Before("execution(* com.example.service.*.*(..))")public void logBefore(JoinPoint joinPoint) {System.out.println("Before method: " + joinPoint.getSignature().getName());} }
Spring 框架的主要模块
-
Spring Core
Spring Core 是 Spring 框架的基础模块,提供了依赖注入和 IoC 容器的实现。它包括 BeanFactory、ApplicationContext 等核心组件,负责管理应用程序中的对象。
-
Spring Context
Spring Context 是 Spring Core 的扩展,提供了框架级别的支持,包括国际化 (i18n)、事件传播、资源加载等。它还支持注解配置和基于 Java 的配置。
@Configuration public class AppConfig {@Beanpublic HelloWorld helloWorld() {return new HelloWorld();} }
-
Spring AOP
Spring AOP 模块提供了 AOP 支持,使得开发者可以使用注解或 XML 配置来定义切面和切点 (pointcut),实现横切关注点的分离。
-
Spring JDBC
Spring JDBC 模块简化了数据库访问,提供了一致的异常处理机制和简单的模板类(如 JdbcTemplate),减少了重复代码和复杂性。
@Repository public class UserDao {@Autowiredprivate JdbcTemplate jdbcTemplate;public List<User> findAll() {return jdbcTemplate.query("SELECT * FROM users", new UserRowMapper());} }
-
Spring ORM
Spring ORM 模块集成了流行的 ORM 框架,如 Hibernate、JPA 和 MyBatis。它简化了 ORM 的配置和使用,并提供了统一的事务管理机制。
@Entity public class User {@Id@GeneratedValue(strategy = GenerationType.IDENTITY)private Long id;private String name;// getters and setters }
-
Spring MVC
Spring MVC 是一个全功能的 MVC 框架,提供了丰富的注解支持,使得开发者可以轻松地构建 Web 应用程序。它包括 DispatcherServlet、Controller、ViewResolver 等核心组件。
@Controller public class HomeController {@GetMapping("/home")public String home(Model model) {model.addAttribute("message", "Welcome to Spring MVC");return "home";} }
-
Spring Security
Spring Security 是一个强大的安全框架,提供了全面的认证和授权功能。它支持基于 URL、方法和表达式的安全控制,并可以与多种身份验证机制集成。
@EnableWebSecurity public class WebSecurityConfig extends WebSecurityConfigurerAdapter {@Overrideprotected void configure(HttpSecurity http) throws Exception {http.authorizeRequests().antMatchers("/admin/**").hasRole("ADMIN").anyRequest().authenticated().and().formLogin().loginPage("/login").permitAll().and().logout().permitAll();} }
Spring Boot:简化 Spring 开发
Spring Boot 是 Spring 框架的扩展项目,通过提供一套默认配置和一系列自动配置的 Starter,使得开发者可以快速搭建 Spring 应用程序。Spring Boot 提供了独立运行的 Spring 应用程序和嵌入式服务器(如 Tomcat、Jetty),大大简化了开发和部署过程。
@SpringBootApplication
public class Application {public static void main(String[] args) {SpringApplication.run(Application.class, args);}
}
Spring Cloud:构建微服务架构
Spring Cloud 是一组用于构建分布式系统和微服务架构的工具集。它基于 Spring Boot,提供了一整套解决方案,如服务注册与发现、分布式配置管理、断路器、负载均衡等。
-
服务注册与发现
使用 Netflix Eureka 实现服务注册与发现,使得微服务可以动态注册和发现彼此。
@EnableEurekaServer @SpringBootApplication public class EurekaServerApplication {public static void main(String[] args) {SpringApplication.run(EurekaServerApplication.class, args);} }
-
分布式配置管理
使用 Spring Cloud Config 管理分布式系统中的配置,使得配置可以集中管理和动态更新。
@EnableConfigServer @SpringBootApplication public class ConfigServerApplication {public static void main(String[] args) {SpringApplication.run(ConfigServerApplication.class, args);} }
-
断路器
使用 Netflix Hystrix 实现断路器模式,提高系统的容错性和稳定性。
@RestController public class HystrixController {@HystrixCommand(fallbackMethod = "fallback")@GetMapping("/service")public String service() {// 调用其他微服务return restTemplate.getForObject("http://other-service/api", String.class);}public String fallback() {return "Fallback response";} }
实际案例分析
为了更好地理解 Spring 框架的应用,下面通过一个实际案例分析,展示如何使用 Spring 进行企业级应用开发。
-
需求分析
假设我们需要开发一个电商平台,包括用户管理、商品管理、订单管理等模块。我们希望系统具有良好的扩展性和维护性。
-
架构设计
我们将系统划分为多个微服务,每个微服务负责特定的业务领域。使用 Spring Boot 快速搭建每个微服务,使用 Spring Cloud 实现服务之间的通信和管理。
-
实现用户管理服务
用户管理服务包括用户注册、登录、查询等功能。使用 Spring Data JPA 进行数据持久化,使用 Spring Security 实现用户认证和授权。
@Entity public class User {@Id@GeneratedValue(strategy = GenerationType.IDENTITY)private Long id;private String username;private String password;// getters and setters }
@Repository public interface UserRepository extends JpaRepository<User, Long> {User findByUsername(String username); }
@Service public class UserService {@Autowiredprivate UserRepository userRepository;public User register(User user) {return userRepository.save(user);}public User findByUsername(String username) {return userRepository.findByUsername(username);} }
@RestController public class UserController {@Autowiredprivate UserService userService;@PostMapping("/register")public User register(@RequestBody User user) {return userService.register(user);}@GetMapping("/user/{username}")public User getUser(@PathVariable String username) {return userService.findByUsername(username);} }
-
实现商品管理服务
商品管理服务包括商品的添加、查询、更新和删除等功能。使用 Spring Data JPA 进行数据持久化,使用 RESTful 风格的 API 提供服务。
@Entity public class Product {@Id@GeneratedValue(strategy = GenerationType.IDENTITY)private Long id;private String name;private Double price;// getters and setters }
@Repository public interface ProductRepository extends JpaRepository<Product, Long> { }
@Service public class ProductService {@Autowiredprivate ProductRepository productRepository;public Product addProduct(Product product) {return productRepository.save(product);}public List<Product> getAllProducts() {return productRepository.findAll();}public void deleteProduct(Long id) {productRepository.deleteById(id);} }
@RestController public class ProductController {@Autowiredprivate ProductService productService;@PostMapping("/products")public Product addProduct(@RequestBody Product product) {return productService.addProduct(product);}@GetMapping("/products")public List<Product> getAllProducts() {return productService.getAllProducts();}@DeleteMapping("/products/{id}")public void deleteProduct(@PathVariable Long id) {productService.deleteProduct(id);} }
-
实现订单管理服务
订单管理服务包括订单的创建、查询、更新和删除等功能。使用 Spring Data JPA 进行数据持久化,使用 RESTful 风格的 API 提供服务。
@Entity public class Order {@Id@GeneratedValue(strategy = GenerationType.IDENTITY)private Long id;private Long userId;private Long productId;private Integer quantity;private Double totalPrice;// getters and setters }
@Repository public interface OrderRepository extends JpaRepository<Order, Long> { }
@Service public class OrderService {@Autowiredprivate OrderRepository orderRepository;public Order createOrder(Order order) {return orderRepository.save(order);}public List<Order> getAllOrders() {return orderRepository.findAll();}public void deleteOrder(Long id) {orderRepository.deleteById(id);} }
@RestController public class OrderController {@Autowiredprivate OrderService orderService;@PostMapping("/orders")public Order createOrder(@RequestBody Order order) {return orderService.createOrder(order);}@GetMapping("/orders")public List<Order> getAllOrders() {return orderService.getAllOrders();}@DeleteMapping("/orders/{id}")public void deleteOrder(@PathVariable Long id) {orderService.deleteOrder(id);} }
结论
Spring 框架通过其丰富的模块和灵活的设计,为 Java 企业级开发提供了强大的支持。从依赖注入、面向切面编程到完整的 Web MVC 和安全解决方案,Spring 覆盖了应用开发的各个方面。Spring Boot 和 Spring Cloud 更是进一步简化了微服务架构的开发和部署,使得复杂的企业应用开发变得高效而有序。通过本文的介绍,相信读者能够更好地理解 Spring 框架的核心概念和实际应用,进而在实际项目中发挥其强大的威力。