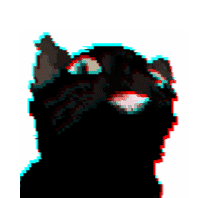

目录
- 安装
- 基本用法
- 使用多个提示框
- 动态选择(动态选项)
- 表单式输入
- 配合lerna在Vue中使用示例
Inquirer
是一个用于创建交互式命令行工具的 Node.js 库,常用于收集用户输入。它提供了多种类型的提示框,可以用于创建交互式应用程序(比如 CLI 工具),并且支持各种类型的输入,包括文本、选择框、确认框、密码输入框等。这个库在开发过程中非常有用,尤其是在构建脚本和自动化工具时。
安装
首先,你需要安装 inquirer
:
npm install inquirer
基本用法
- 文本输入框(input)
input
提示允许用户输入一段文本。
javascript">const inquirer = require('inquirer');inquirer.prompt([{type: 'input',name: 'username',message: 'Enter your username:'}
]).then(answers => {console.log(`Hello, ${answers.username}!`);
});
上面的代码会显示一个提示框,要求用户输入他们的用户名。
- 选择框(list)
list
提示允许用户从一组选项中选择一个。
javascript">inquirer.prompt([{type: 'list',name: 'color',message: 'Choose your favorite color:',choices: ['Red', 'Green', 'Blue']}
]).then(answers => {console.log(`Your favorite color is ${answers.color}`);
});
此代码会显示一个选择框,允许用户选择一个颜色。
- 多选框(checkbox)
checkbox
提示允许用户从多个选项中选择一个或多个。
javascript">inquirer.prompt([{type: 'checkbox',name: 'fruits',message: 'Select your favorite fruits:',choices: ['Apple', 'Banana', 'Cherry', 'Date']}
]).then(answers => {console.log(`You selected: ${answers.fruits.join(', ')}`);
});
在这个例子中,用户可以选择多个水果。
- 确认框(confirm)
confirm
提示要求用户确认某个操作(例如“是”或“否”)。
javascript">inquirer.prompt([{type: 'confirm',name: 'proceed',message: 'Do you want to proceed?'}
]).then(answers => {if (answers.proceed) {console.log('Proceeding...');} else {console.log('Operation cancelled.');}
});
- 密码输入框(password)
password
提示框会隐藏用户输入(用于收集密码等敏感信息)。
javascript">inquirer.prompt([{type: 'password',name: 'password',message: 'Enter your password:'}
]).then(answers => {console.log('Password entered:', answers.password);
});
使用多个提示框
你可以同时使用多个不同类型的提示框,通过将它们组合到 prompt
数组中。
javascript">inquirer.prompt([{type: 'input',name: 'name',message: 'What is your name?'},{type: 'list',name: 'color',message: 'What is your favorite color?',choices: ['Red', 'Green', 'Blue']}
]).then(answers => {console.log(`Hello, ${answers.name}. Your favorite color is ${answers.color}.`);
});
动态选择(动态选项)
inquirer
允许你根据用户输入动态生成选项。
javascript">inquirer.prompt([{type: 'input',name: 'country',message: 'Which country are you from?'},{type: 'list',name: 'city',message: 'Which city do you live in?',choices: function(answers) {const cities = {'USA': ['New York', 'Los Angeles', 'Chicago'],'India': ['Delhi', 'Mumbai', 'Bangalore']};return cities[answers.country] || [];}}
]).then(answers => {console.log(`You are from ${answers.city}, ${answers.country}.`);
});
在这个示例中,城市选项会根据用户选择的国家动态变化。
表单式输入
inquirer
还支持通过 form
提示收集多个输入。
javascript">const questions = [{type: 'input',name: 'name',message: 'What is your name?'},{type: 'input',name: 'age',message: 'What is your age?'}
];inquirer.prompt(questions).then(answers => {console.log(`Name: ${answers.name}, Age: ${answers.age}`);
});
配合lerna在Vue中使用示例
- 项目中根目录下新建文件
run-project.js
javascript">const fs = require('fs');
const path = require('path');
const { exec } = require('child_process');
const inquirer = require('inquirer');// 获取所有子项目
const packagesDir = path.join(__dirname, 'packages');
const projects = fs.readdirSync(packagesDir).filter((dir) => {const projectPath = path.join(packagesDir, dir);return fs.statSync(projectPath).isDirectory();
});// 如果没有子项目,提示并退出
if (projects.length === 0) {console.log('没有找到任何子项目!');process.exit(1);
}// 让用户选择要运行的项目
inquirer.prompt([{type: 'list',name: 'project',message: '请选择要运行的项目:',choices: projects,},]).then((answers) => {const selectedProject = answers.project;const projectPath = path.join(packagesDir, selectedProject);// 检查项目是否有 dev 脚本const packageJsonPath = path.join(projectPath, 'package.json');if (!fs.existsSync(packageJsonPath)) {console.log(`项目 ${selectedProject} 没有 package.json 文件!`);process.exit(1);}const packageJson = require(packageJsonPath);if (!packageJson.scripts || !packageJson.scripts.dev) {console.log(`项目 ${selectedProject} 没有 dev 脚本!`);process.exit(1);}// 运行项目的 dev 脚本console.log(`正在运行项目 ${selectedProject}...`);const childProcess = exec(`cd ${projectPath} && npm run dev`, (error, stdout, stderr) => {if (error) {console.error(`执行错误: ${error.message}`);return;}if (stderr) {console.error(`错误输出: ${stderr}`);return;}console.log(`输出: ${stdout}`);});// 监听子进程的输出childProcess.stdout.on('data', (data) => {console.log(data);});childProcess.stderr.on('data', (data) => {console.error(data);});}).catch((error) => {console.error('发生错误:', error);});
- 在package.json里面写入配置
javascript">"scripts": {"start": "node run-project.js"},
- 终端运行
npm start