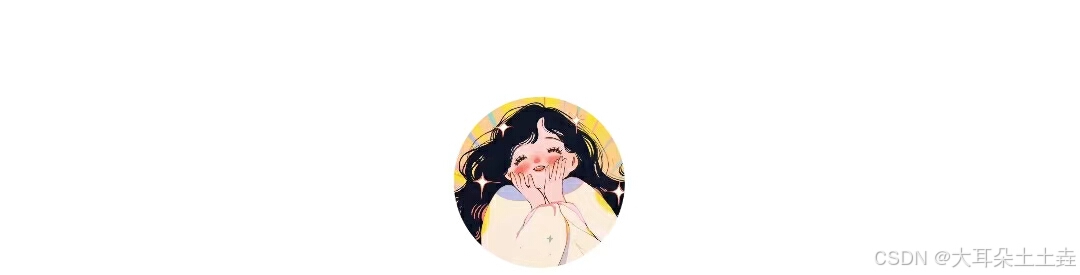

文章目录
- 1. 什么是设计模式
- 2. 日志认识
- 3. 日志实现
- 4. 结语
1. 什么是设计模式
IT行业这么火, 涌入的人很多. 俗话说林子大了啥鸟都有. 大佬和菜鸡们两极分化的越来越严重. 为了让菜鸡们不太拖大佬的后腿, 于是大佬们针对⼀些经典的常见的场景, 给定了⼀些对应的解决⽅案, 这个就是设计模式。
在IT行业中,设计模式(Design Patterns) 是一套被广泛认可的、用于解决软件设计中常见问题的最佳实践。它们提供了一种标准化的方法来处理特定的设计问题,并且可以帮助开发人员编写更清晰、更具可维护性的代码。
2. 日志认识
计算机中的日志是记录系统和软件运行中发生事件的文件,主要作用是监控运行状态、记录异常信息,帮助快速定位问题并⽀持程序员进⾏问题修复。它是系统维护、故障排查和安全管理的重要工具。
日志格式以下几个指标是必须得有的:
- 时间戳
- 日志等级
- 日志内容
以下几个指标是可选的:
- 文件名行号
- 进程,线程相关id信息等
日志有现成的解决方案,如:spdlog、glog、Boost.Log、Log4cxx等等,我们依旧采用自定义日志的方式。这里我们采用设计模式-策略模式来进行日志的设计,我们想要的日志格式如下:
[可读性很好的时间] [⽇志等级] [进程pid] [打印对应⽇志的⽂件名][⾏号] - 消息内容,⽀持可变参数
[2024-08-04 12:27:03] [DEBUG] [202938] [main.cc] [16] - hello world
[2024-08-04 12:27:03] [DEBUG] [202938] [main.cc] [17] - hello world
[2024-08-04 12:27:03] [DEBUG] [202938] [main.cc] [18] - hello world
[2024-08-04 12:27:03] [DEBUG] [202938] [main.cc] [20] - hello world
[2024-08-04 12:27:03] [DEBUG] [202938] [main.cc] [21] - hello world
[2024-08-04 12:27:03] [WARNING] [202938] [main.cc] [23] - hello world
3. 日志实现
- 首先我们需要设置日志等级:
// ⽇志等级enum class LogLevel{DEBUG,INFO,WARNING,ERROR,FATAL};// ⽇志转换成为字符串std::string LogLevelToString(LogLevel level){switch (level){case LogLevel::DEBUG:return "DEBUG";case LogLevel::INFO:return "INFO";case LogLevel::WARNING:return "WARNING";case LogLevel::ERROR:return "ERROR";case LogLevel::FATAL:return "FATAL";default:return "UNKNOWN";}}
- 设置日志时间
// 根据时间戳,获取可读性较强的时间信息std::string GetCurrTime(){time_t tm = time(nullptr);struct tm curr;localtime_r(&tm, &curr);char timebuffer[64];snprintf(timebuffer, sizeof(timebuffer), "%4d-%02d-%02d %02d:%02d:%02d ",curr.tm_year + 1900,//这是因为这里的年份比实际年份少1900curr.tm_mon+1,//这是因为月份是在0~11curr.tm_mday,curr.tm_hour,curr.tm_min,curr.tm_sec);return timebuffer;}
- 日志策略模式:
有了准备工作后,我们在开始设计日志类之前还需要确定日志的策略模式——也就是日志是往控制台上输出还是文件中输出。
// 策略模式,策略接⼝class LogStrategy{public:virtual ~LogStrategy() = default; // 策略的析构函数virtual void SyncLog(const std::string &message) = 0; // 不同模式核⼼是刷新⽅式的不同};
先定义一个策略模式的基类,然后分别设计控制台打印和文件打印两个子类,子类必须实现基类的纯虚函数接口
SyncLog
。
- 控制台日志策略:
// 控制台⽇志策略,就是⽇志只向显⽰器打印,⽅便我们debugclass ConsoleLogStrategy : public LogStrategy{public:void SyncLog(const std::string &message) override{LockGuard LockGuard(_mutex);std::cerr << message << std::endl;}~ConsoleLogStrategy(){// std::cout << "~ConsoleLogStrategy" << std::endl; // for debug}private:Mutex _mutex; // 显⽰器也是临界资源,保证输出线程安全};
因为打印时可能会有多个线程访问,所以需要在打印信息前进行加锁保护,相应的在文件中打印也需要保护。
- 文件日志策略:
class FileLogStrategy : public LogStrategy{public:// 构造函数,建⽴出来指定的⽬录结构和⽂件结构FileLogStrategy(const std::string logpath = defaultpath, std::string logfilename = defaultname): _logpath(logpath), _logfilename(logfilename){LockGuard lockguard(_mutex);if (std::filesystem::exists(_logpath))return;try{std::filesystem::create_directories(_logpath);}catch (const std::filesystem::filesystem_error &e){std::cerr << e.what() << '\n';}}// 将⼀条⽇志信息写⼊到⽂件中void SyncLog(const std::string &message) override{LockGuard lockguard(_mutex);std::string log = _logpath + _logfilename;std::ofstream out(log.c_str(), std::ios::app); // 追加⽅式if (!out.is_open())return;out << message << "\n";out.close();}~FileLogStrategy(){// std::cout << "~FileLogStrategy" << std::endl; // for debug}private:std::string _logpath;std::string _logfilename;Mutex _mutex; // 保证输出线程安全,粗狂⽅式下,可以不⽤};
- 具体日志类:
我们先确定日志策略模式,默认是控制台输出;然后定义一个内部类用来确定日志输出的信息:
// 具体的⽇志类class Logger{public:Logger(){// 默认使⽤显⽰器策略,如果⽤⼾⼆次指明了策略,会释放在申请,测试的时候注意析构次数UseConsoleStrategy();}~Logger(){}void UseConsoleStrategy(){_strategy = std::make_unique<ConsoleLogStrategy>();}void UseFileStrategy(){_strategy = std::make_unique<FileLogStrategy>();}class LogMessage{public:LogMessage(LogLevel type, std::string filename, int line, Logger &logger): _curr_time(GetCurrTime()),_pid(getpid()),_filename(filename),_line(line),_logger(logger){// stringstream不允许拷⻉,所以这⾥就当做格式化功能使⽤std::stringstream ssbuffer;ssbuffer << "[" << _curr_time <<"]"<< "[" << LogLevelToString(type) << "] "<< "[" << _pid << "] "<< "[" << _filename << "] "<< "[" << _line << "]"<< " - ";_loginfo = ssbuffer.str();}template <typename T>LogMessage &operator<<(const T &info){std::stringstream ssbuffer;ssbuffer << info;_loginfo += ssbuffer.str();return *this; // 返回当前LogMessage对象,⽅便下次继续进⾏<<}// RAII⻛格,析构的时候进⾏⽇志持久化,采⽤指定的策略~LogMessage(){if (_logger._strategy){_logger._strategy->SyncLog(_loginfo);}}private:LogLevel _type; // ⽇志等级std::string _curr_time; // ⽇志时间pid_t _pid; // 写⼊⽇志的进程IDstd::string _filename; // 对应的⽂件名int _line; // 对应的⽂件⾏号Logger &_logger; // 引⽤外部logger类, ⽅便使⽤策略进⾏刷新std::string _loginfo; // ⼀条合并完成的,完整的⽇志信息};LogMessage operator()(LogLevel type, std::string filename, int line){return LogMessage(type, filename, line, *this);}private:std::unique_ptr<LogStrategy> _strategy;};
使用智能指针方便管理与释放资源,因为需要自定义输出日志信息所以我们需要在内部类中重载
<<
,为了方便使用我们还在日志类中重载()
。
- 最后将上述内容放在一个命名空间
LogModule
内部,并定义一个日志类对象:
#include <iostream>
#include <string>
#include <fstream>
#include <memory>
#include <ctime>
#include <sstream>
#include <filesystem> // C++17, 需要⾼版本编译器和-std=c++17
#include <unistd.h>#include "Mutex.hpp"namespace LogModule
{using namespace MutexModule;// 默认路径和⽇志名称const std::string defaultpath = "./log/";const std::string defaultname = "log.txt";// ⽇志等级enum class LogLevel{DEBUG,INFO,WARNING,ERROR,FATAL};// ⽇志转换成为字符串std::string LogLevelToString(LogLevel level){switch (level){case LogLevel::DEBUG:return "DEBUG";case LogLevel::INFO:return "INFO";case LogLevel::WARNING:return "WARNING";case LogLevel::ERROR:return "ERROR";case LogLevel::FATAL:return "FATAL";default:return "UNKNOWN";}}// 根据时间戳,获取可读性较强的时间信息std::string GetCurrTime(){time_t tm = time(nullptr);struct tm curr;localtime_r(&tm, &curr);char timebuffer[64];snprintf(timebuffer, sizeof(timebuffer), "%4d-%02d-%02d %02d:%02d:%02d ",curr.tm_year + 1900,curr.tm_mon+1,curr.tm_mday,curr.tm_hour,curr.tm_min,curr.tm_sec);return timebuffer;}// 策略模式,策略接⼝class LogStrategy{public:virtual ~LogStrategy() = default; // 策略的构造函数virtual void SyncLog(const std::string &message) = 0; // 不同模式核⼼是刷新⽅式的不同};// 控制台⽇志策略,就是⽇志只向显⽰器打印,⽅便我们debugclass ConsoleLogStrategy : public LogStrategy{public:void SyncLog(const std::string &message) override{LockGuard LockGuard(_mutex);std::cerr << message << std::endl;}~ConsoleLogStrategy(){// std::cout << "~ConsoleLogStrategy" << std::endl; // for debug}private:Mutex _mutex; // 显⽰器也是临界资源,保证输出线程安全};// ⽂件⽇志策略class FileLogStrategy : public LogStrategy{public:// 构造函数,建⽴出来指定的⽬录结构和⽂件结构FileLogStrategy(const std::string logpath = defaultpath, std::string logfilename = defaultname): _logpath(logpath), _logfilename(logfilename){LockGuard lockguard(_mutex);if (std::filesystem::exists(_logpath))return;try{std::filesystem::create_directories(_logpath);}catch (const std::filesystem::filesystem_error &e){std::cerr << e.what() << '\n';}}// 将⼀条⽇志信息写⼊到⽂件中void SyncLog(const std::string &message) override{LockGuard lockguard(_mutex);std::string log = _logpath + _logfilename;std::ofstream out(log.c_str(), std::ios::app); // 追加⽅式if (!out.is_open())return;out << message << "\n";out.close();}~FileLogStrategy(){// std::cout << "~FileLogStrategy" << std::endl; // for debug}private:std::string _logpath;std::string _logfilename;Mutex _mutex; // 保证输出线程安全,粗狂⽅式下,可以不⽤};// 具体的⽇志类class Logger{public:Logger(){// 默认使⽤显⽰器策略,如果⽤⼾⼆次指明了策略,会释放在申请,测试的时候注意析构次数UseConsoleStrategy();}~Logger(){}void UseConsoleStrategy(){_strategy = std::make_unique<ConsoleLogStrategy>();}void UseFileStrategy(){_strategy = std::make_unique<FileLogStrategy>();}class LogMessage{public:LogMessage(LogLevel type, std::string filename, int line, Logger &logger): _curr_time(GetCurrTime()),_pid(getpid()),_filename(filename),_line(line),_logger(logger){// stringstream不允许拷⻉,所以这⾥就当做格式化功能使⽤std::stringstream ssbuffer;ssbuffer << "[" << _curr_time <<"]"<< "[" << LogLevelToString(type) << "] "<< "[" << _pid << "] "<< "[" << _filename << "] "<< "[" << _line << "]"<< " - ";_loginfo = ssbuffer.str();}template <typename T>LogMessage &operator<<(const T &info){std::stringstream ssbuffer;ssbuffer << info;_loginfo += ssbuffer.str();return *this; // 返回当前LogMessage对象,⽅便下次继续进⾏<<}// RAII⻛格,析构的时候进⾏⽇志持久化,采⽤指定的策略~LogMessage(){if (_logger._strategy){_logger._strategy->SyncLog(_loginfo);}}private:LogLevel _type; // ⽇志等级std::string _curr_time; // ⽇志时间pid_t _pid; // 写⼊⽇志的进程IDstd::string _filename; // 对应的⽂件名int _line; // 对应的⽂件⾏号Logger &_logger; // 引⽤外部logger类, ⽅便使⽤策略进⾏刷新std::string _loginfo; // ⼀条合并完成的,完整的⽇志信息};LogMessage operator()(LogLevel type, std::string filename, int line){return LogMessage(type, filename, line, *this);}private:std::unique_ptr<LogStrategy> _strategy;};//定义日志类对象Logger logger;// 使⽤宏,可以进⾏代码插⼊,⽅便随时获取⽂件名和⾏号#define LOG(type) logger(type, __FILE__, __LINE__)// 提供选择使⽤何种⽇志策略的⽅法#define ENABLE_CONSOLE_LOG_STRATEGY() logger.UseConsoleStrategy()#define ENABLE_FILE_LOG_STRATEGY() logger.UseFileStrategy()
}
此外我们还使用了宏方便调用。
- 测试代码:
#include <iostream>
#include "Log.hpp"
using namespace LogModule;
void fun()
{int a = 10;LOG(LogLevel::FATAL) << "hello world" << 1234 << ", 3.14" << 'c' << a;
}
int main()
{// ENABLE_CONSOLE_LOG_STRATEGY();LOG(LogLevel::DEBUG) << "hello world";LOG(LogLevel::ERROR) << "hello world";LOG(LogLevel::DEBUG) << "hello world";// ENABLE_FILE_LOG_STRATEGY();LOG(LogLevel::FATAL) << "hello world";LOG(LogLevel::INFO) << "hello world";LOG(LogLevel::WARNING) << "hello world";fun();return 0;
}
结果如下:
4. 结语
日志可以帮助我们快速准确的了解程序运行的状况,出现的错误以及相关内容;同时日志的设计模式如解耦也值得我们学习。以上就是今天所有的内容啦~ 完结撒花 ~ 🥳🎉🎉