#include <iostream>
#include <cstring>
using namespace std;template<typename T>
class Stack
{
private:int len;int count = 0;T *stack;
public:Stack():len(10) //无参构造{stack = new T[len];stack[len] = {0};}Stack(int len):len(len) //有参构造{stack = new T[len];stack[len] = {0};}Stack(Stack &other):len(other.len) //拷贝构造函数{stack = new T[len];for(int i=0;i<len;i++){stack[i] = other.stack[i];}}Stack &operator=(const Stack &other) //拷贝赋值函数{if(this != &other){this->count = other.count;this->len = other.len;int *newstack = new T[this->len];for(int i=0;i<len;i++){newstack[i] = other.stack[i];}delete [] stack;stack = newstack;}return *this;}~Stack() //析构函数{delete [] stack;}T top();bool empty();int size();int push(T n);void expand();void show();int pop();
};
template<typename T>
T Stack<T>::top()
{if(empty()){cout<<"error" <<endl;return -1;}return stack[count-1];
}
template<typename T>
bool Stack<T>::empty()
{return count == 0;
}template<typename T>
int Stack<T>::size()
{return count;
}template<typename T>
int Stack<T>::push(T n)
{if(count == len){expand();}stack[count++] = n;return 0;
}template<typename T>
void Stack<T>::expand()
{len = len * 2;int *newstack = new T[len];for(int i=0;i<count;i++){newstack[i] = stack[i];}delete [] stack;stack = newstack;
}
template<typename T>
void Stack<T>::show()
{for(int i=count-1;i>=0;i--){cout<<stack[i]<<" ";}cout<<endl;
}template<typename T>
int Stack<T>::pop()
{if(empty()){cout<<"error"<<endl;return -1;}stack[--count] = 0;return 0;
}
template<typename K>
class Queue
{
private:int len;int count = 0;K *queue;
public:Queue():len(10){queue = new K[len];queue[len] = {0};};Queue(int n):len(n){queue = new K[len];queue[len] = {0};};Queue(Queue &other):len(other.len){queue = new K[len];for(int i=0;i<len;i++){queue[i] = other.queue[i];}};Queue &operator=(const Queue &other) //拷贝赋值函数{if(this != &other){this->count = other.count;this->len = other.len;int *newqueue = new K[this->len];for(int i=0;i<len;i++){newqueue[i] = other.queue[i];}delete [] queue;queue = newqueue;}return *this;}~Queue(){delete []queue;};K front();K back();bool empty();int size();int push(K n);void pop();void expand();void show();
};
template<typename K>
K Queue<K>::front()
{if(empty()){cout<<"error"<<endl;return -1;}return queue[count-1];
}
template<typename K>
K Queue<K>::back()
{if(empty()){cout<<"error"<<endl;return -1;}return queue[0];
}
template<typename K>
bool Queue<K>::empty()
{return count == 0;
}
template<typename K>
int Queue<K>::size()
{return count;
}
template<typename K>
int Queue<K>::push(K n)
{if(count == len){expand();}queue[count++] = n;return 0;
}
template<typename K>
void Queue<K>::pop()
{for(int i=0;i<count;i++){queue[i] = queue[i+1];}count--;
}
template<typename K>
void Queue<K>::expand()
{len = len * 2;int *newqueue = new K[len];for(int i=0;i<count;i++){newqueue[i] = queue[i];}delete [] queue;queue = newqueue;
}
template<typename K>
void Queue<K>::show()
{for(int i=0;i<count;i++){cout<<queue[i]<<" ";}cout<<endl;
}
int main()
{Stack<int> s1(3);s1.push(1);s1.push(2);s1.push(3);s1.push(3);s1.pop();Stack<int> s2;s2 = s1;s1.show();s2.show();cout<<s2.top()<<" "<<s2.size()<<endl;cout<<"************"<<endl;Queue<int> q1(3);q1.push(1);q1.push(2);q1.push(3);q1.show();q1.pop();q1.show();return 0;
}
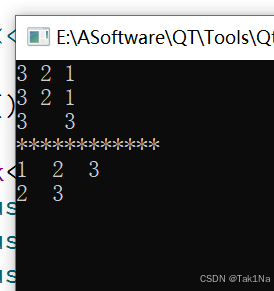
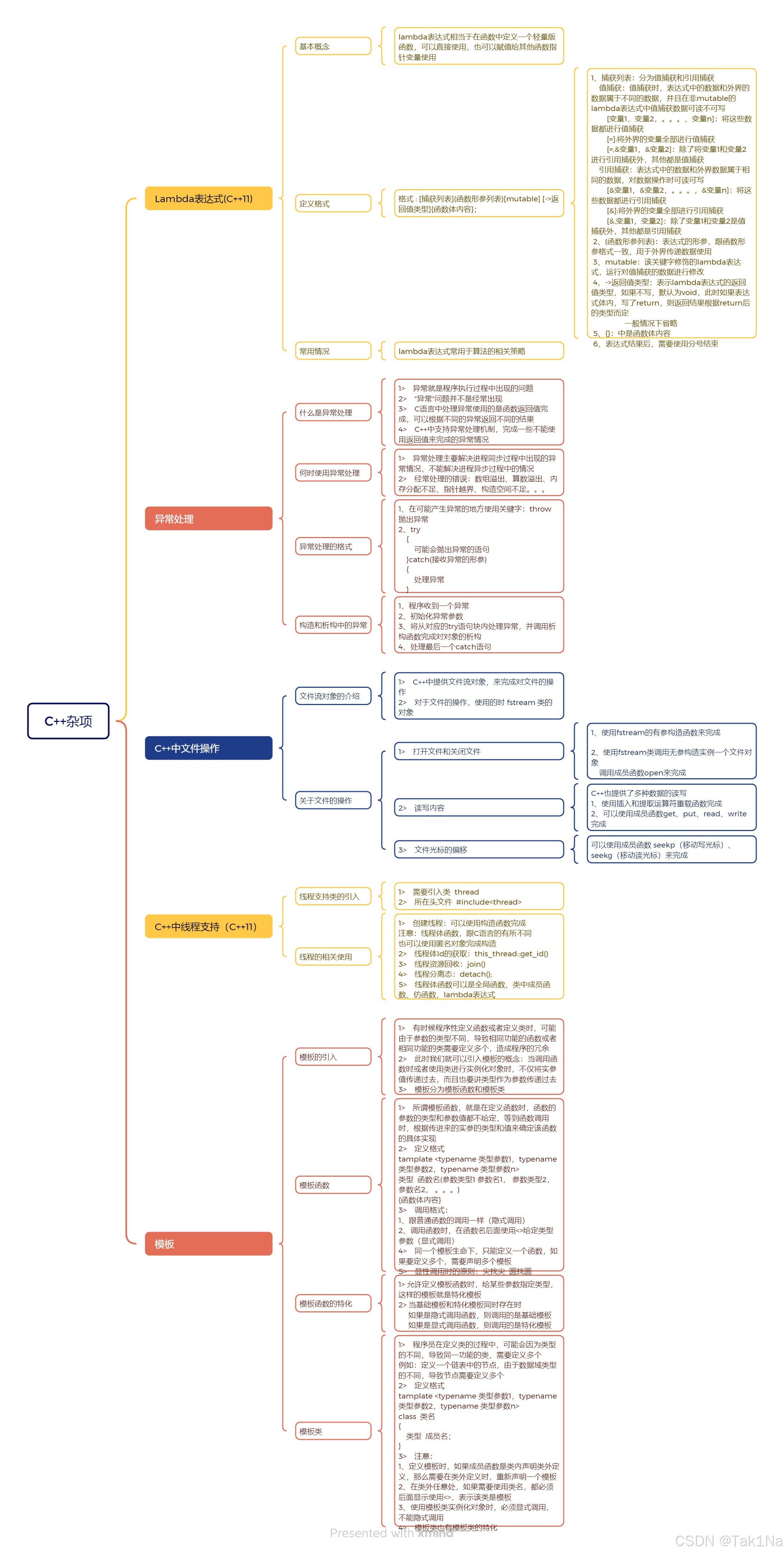